README.md in sinclair-1.0.0 vs README.md in sinclair-1.1.0
- old
+ new
@@ -1,13 +1,18 @@
Sinclair
========
+[](https://codeclimate.com/github/darthjee/sinclair)
+[](https://codeclimate.com/github/darthjee/sinclair/coverage)
+[](https://codeclimate.com/github/darthjee/sinclair)
+
+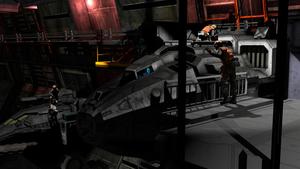
+
This gem helps the creation of complex concern with class methods
-Getting started
+Installation
---------------
-1. Installation
- Install it
```ruby
gem install sinclair
```
@@ -20,11 +25,12 @@
```bash
bundle install sinclair
```
-2. Using it:
+Usage
+---------------
The concern builder can actully be used in two ways, as an stand alone object capable of
adding methods to your class or by extending it for more complex logics
- Stand Alone usage:
```ruby
@@ -148,5 +154,72 @@
returns
```
false
```
+
+RSspec matcher
+---------------
+
+You can use the provided matcher to check that your builder is adding a method correctly
+
+```ruby
+class DefaultValue
+ delegate :build, to: :builder
+ attr_reader :klass, :method, :value
+
+ def initialize(klass, method, value)
+ @klass = klass
+ @method = method
+ @value = value
+ end
+
+ private
+
+ def builder
+ @builder ||= Sinclair.new(klass).tap do |b|
+ b.add_method(method) { value }
+ end
+ end
+end
+
+RSpec.configure do |config|
+ config.include Sinclair::Matchers
+end
+
+RSpec.describe DefaultValue do
+ let(:klass) { Class.new }
+ let(:method) { :the_method }
+ let(:value) { Random.rand(100) }
+ let(:builder) { described_class.new(klass, method, value) }
+ let(:instance) { klass.new }
+
+ context 'when the builder runs' do
+ it do
+ expect do
+ described_class.new(klass, method, value).build
+ end.to add_method(method).to(instance)
+ end
+ end
+
+ context 'when the builder runs' do
+ it do
+ expect do
+ described_class.new(klass, method, value).build
+ end.to add_method(method).to(klass)
+ end
+ end
+end
+```
+
+```bash
+> bundle exec rspec
+```
+
+```string
+DefaultValue
+ when the builder runs
+ should add method 'the_method' to #<Class:0x0000000146c160> instances
+ when the builder runs
+ should add method 'the_method' to #<Class:0x0000000143a1b0> instances
+
+```