README.md in shoden-0.4.0 vs README.md in shoden-1.0
- old
+ new
@@ -1,19 +1,32 @@
-# Shôden - [](http://badge.fury.io/rb/shoden)
+# Shôden - [](https://travis-ci.org/elcuervo/shoden)
-
+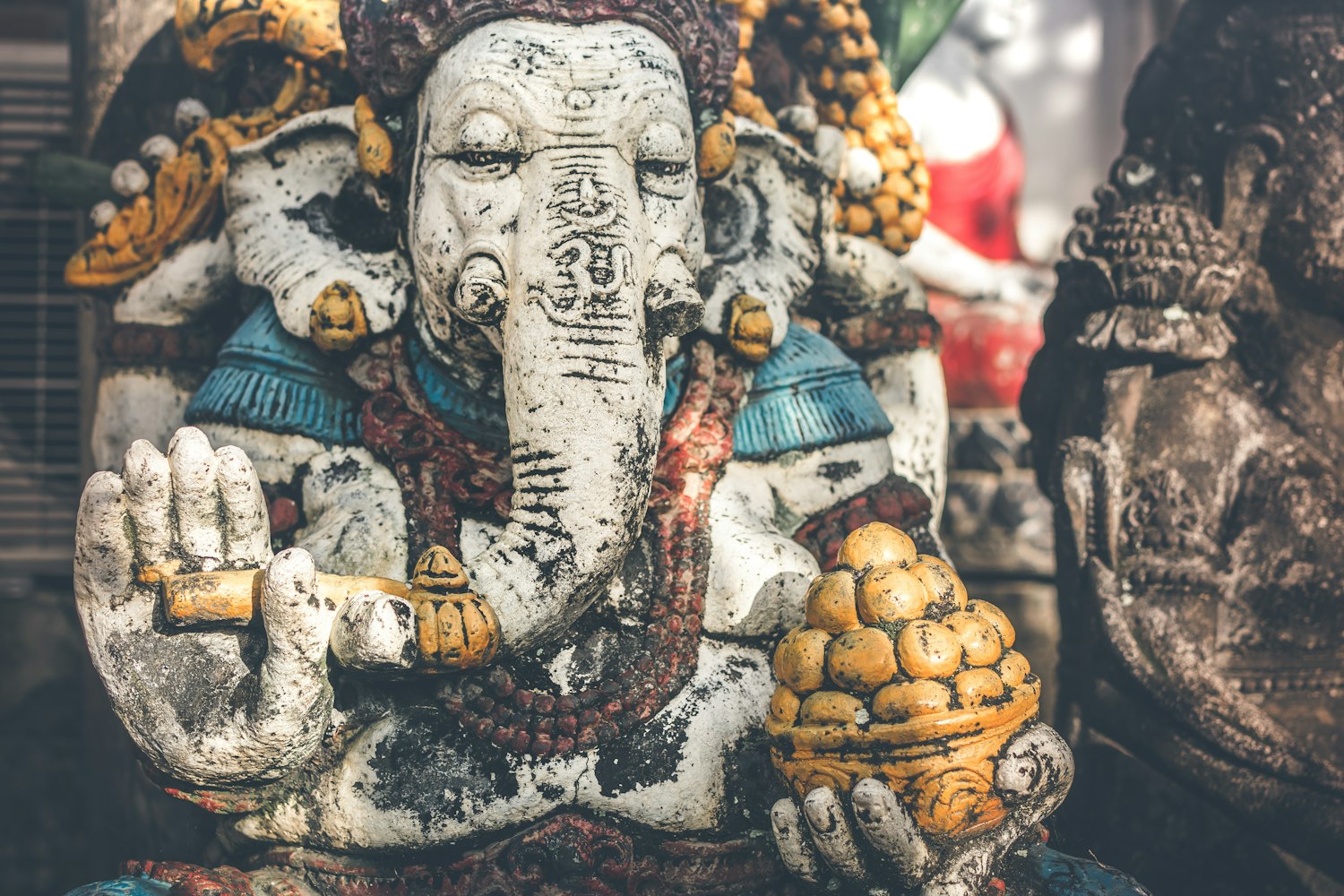
Shôden is a persistance library on top of Postgres.
-It is basically an [Ohm](https://github.com/soveran/ohm) clone but using
-Postgres as a main database.
+Uses JSONB as an storage abstraction so no need for migrations.
## Installation
```bash
gem install shoden
```
+## Connect
+
+Shoden connects by default using a `DATABASE_URL` env variable.
+But you can change the connection string by calling `Shoden.url=`
+
+## Setup
+
+Shoden needs a setup method to create the proper tables.
+You should do that after connecting
+
+```ruby
+Shoden.setup
+```
+
## Models
```ruby
class Fruit < Shoden::Model
attribute :type
@@ -45,10 +58,21 @@
reference :owner, :User
end
```
+## Attributes
+
+Shoden attributes offer you a way to type cast the values, or to perform changes
+in the data itself.
+
+```ruby
+class Shout < Shoden::Model
+ attribute :what, ->(x) { x.uppcase }
+end
+```
+
## Indexing
```ruby
class User < Shoden::Model
attribute :email
@@ -56,5 +80,19 @@
index :country
unique :email
end
```
+
+## Querying
+
+You can query models or relations using the `filter` method.
+
+```ruby
+User.filter(email: "elcuervo@elcuervo.net")
+User.first
+User.last
+User.count
+```
+
+You can go through the entire set using: `User.all` which will give you a
+`Enumerator::Lazy`