README.md in rubype-0.2.5 vs README.md in rubype-0.2.6
- old
+ new
@@ -2,21 +2,24 @@
[](http://badge.fury.io/rb/rubype) [](https://travis-ci.org/gogotanaka/Rubype) [](https://gemnasium.com/gogotanaka/Rubype) [](https://codeclimate.com/github/gogotanaka/Rubype)
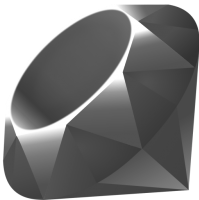
```rb
-# Assert class of both args is Numeric and class of return is String
-def sum(x, y)
- (x + y).to_s
+require 'rubype'
+class MyClass
+ # Assert first arg has method #to_i, second arg and return value are instance of Numeric.
+ def sum(x, y)
+ x.to_i + y
+ end
+ typesig :sum, [:to_i, Numeric] => Numeric
end
-typesig :sum, [Numeric, Numeric] => String
-# Assert first arg has method #to_i
-def sum(x, y)
- x.to_i + y
-end
-typesig :sum, [:to_i, Numeric] => Numeric
+MyClass.new.sum(:has_no_to_i, 2)
+#=> Rubype::ArgumentTypeError: for MyClass#sum's 1st argument
+# Expected: respond to :to_i,
+# Actual: :has_no_to_i
+# ...(stack trace)
```
This gem brings you advantage of type without changing existing code's behavior.
@@ -29,11 +32,18 @@
## Bad point:
* Checking type run every time method call... it might be overhead, but it's not big deal.
* There is no static analysis.
# Feature
+### Super clean!!!
+I know it's terrible to run your important code with such a hacked gem.
+But this contract is implemented by less than 80 lines source code!
+(It is not too little, works well enough)
+https://github.com/gogotanaka/Rubype/blob/develop/lib/rubype.rb#L2-L79
+You can read this over easily and even you can implement by yourself !(Don't need to use this gem, just take idea)
+
### Advantage of type
* Meaningful error
* Executable documentation
* Don't need to check type of method's arguments and return .
@@ -55,14 +65,20 @@
MyClass.new.sum(1, 2)
#=> 3
MyClass.new.sum(1, 'string')
-#=> Rubype::ArgumentTypeError: Expected MyClass#sum's 2nd argument to be Numeric but got "string" instead
+#=> Rubype::ArgumentTypeError: for MyClass#sum's 2nd argument
+# Expected: Numeric,
+# Actual: "string"
+# ...(stack trace)
MyClass.new.wrong_sum(1, 2)
-#=> Rubype::ReturnTypeError: Expected MyClass#wrong_sum to return Numeric but got "string" instead
+#=> Rubype::ReturnTypeError: for MyClass#wrong_sum's return
+# Expected: Numeric,
+# Actual: "string"
+# ...(stack trace)
# ex2: Assert object has specified method
class MyClass
def sum(x, y)
@@ -73,11 +89,14 @@
MyClass.new.sum('1', 2)
#=> 3
MyClass.new.sum(:has_no_to_i, 2)
-#=> Rubype::ArgumentTypeError: Expected MyClass#sum's 1st argument to have method #to_i but got :has_no_to_i instead
+#=> Rubype::ArgumentTypeError: for MyClass#sum's 1st argument
+# Expected: respond to :to_i,
+# Actual: :has_no_to_i
+# ...(stack trace)
# ex3: You can use Any class, if you want
class People
def marry(people)
@@ -88,11 +107,14 @@
People.new.marry(People.new)
#=> no error
People.new.marry('non people')
-#=> Rubype::ArgumentTypeError: Expected People#marry's 1st argument to be People but got "non people" instead
+#=> Rubype::ArgumentTypeError: for People#marry's 1st argument
+# Expected: People,
+# Actual: "non people"
+# ...(stack trace)
```
### Typed method can coexist with non-typed method
@@ -152,10 +174,9 @@
MyClass.new.method(:sum).arg_types
# => [:to_i, Numeric]
MyClass.new.method(:sum).return_type
# => Numeric
-
```
## Benchmarks
```ruby