README.markdown in roar-1.0.0 vs README.markdown in roar-1.0.1
- old
+ new
@@ -6,14 +6,20 @@
Roar is a framework for parsing and rendering REST documents. Nothing more.
Representers let you define your API document structure and semantics. They allow both rendering representations from your models _and_ parsing documents to update your Ruby objects. The bi-directional nature of representers make them interesting for both server and client usage.
-Roar comes with built-in JSON, JSON-HAL, JSON-API and XML support. Its highly modulare architecture provides features like coercion, hypermedia, HTTP transport, client caching and more.
+Roar comes with built-in JSON, JSON-HAL, JSON-API and XML support. Its highly modular architecture provides features like coercion, hypermedia, HTTP transport, client caching and more.
Roar is completely framework-agnostic and loves being used in web kits like Rails, Webmachine, Sinatra, Padrino, etc. If you use Rails, consider [roar-rails](https://github.com/apotonick/roar-rails) for an enjoyable integration.
+<a href="https://leanpub.com/trailblazer">
+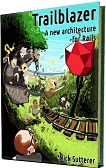
+</a>
+
+Roar is part of the [Trailblazer project](https://github.com/apotonick/trailblazer). Please [buy the book](https://leanpub.com/trailblazer) to support the development. Several chapters will be dedicated to Roar, its integration into operations, hypermedia formats and client-side usage.
+
## Representable
Roar is just a thin layer on top of the [representable](https://github.com/apotonick/representable) gem. While Roar gives you a DSL and behaviour for creating hypermedia APIs, representable implements all the mapping functionality.
If in need for a feature, make sure to check the [representable API docs](https://github.com/apotonick/representable) first.
@@ -233,11 +239,11 @@
## Coercion
Roar provides coercion with the [virtus](https://github.com/solnic/virtus) gem.
```ruby
-require 'roar/feature/coercion'
+require 'roar/coercion'
module SongRepresenter
include Roar::JSON
include Roar::Coercion
@@ -278,13 +284,26 @@
"http://songs/#{title}"
end
end
```
-The `Hypermedia` feature allows declaring links using the `::link` method.
+The `Hypermedia` feature allows declaring links using the `::link` method. In the block, you have access to the represented model. When using representer modules, the block is executed in the model's context.
+However, when using decorators, the context is the decorator instance, allowing you to access additional data. Use `represented` to retrieve model data.
+
```ruby
+class SongRepresenter < Roar::Decorator
+ # ..
+ link :self do
+ "http://songs/#{represented.title}"
+ end
+end
+```
+
+This will render links into your representation.
+
+```ruby
song.extend(SongRepresenter)
song.to_json #=> {"title":"Roxanne","links":[{"rel":"self","href":"http://songs/Roxanne"}]}
```
Per default, links are pushed into the hash using the `links` key. Link blocks are executed in represented context, allowing you to call any instance method of your model (here, we call `#title`).
@@ -356,10 +375,12 @@
end
```
Documentation for HAL can be found in the [API docs](http://rdoc.info/github/apotonick/roar/Roar/Representer/JSON/HAL).
+Make sure you [understand the different contexts](#hypermedia) for links when using decorators.
+
### Hypermedia
Including the `Roar::JSON::HAL` module adds some more DSL methods to your module. It still allows using `::link` but treats them slightly different.
```ruby
@@ -402,18 +423,20 @@
## JSON-API
Roar also supports [JSON-API](http://jsonapi.org/) - yay! It can render _and_ parse singular and collection documents.
+Note that you need representable >= 2.1.4 in your `Gemfile`.
+
### Resource
A minimal representation can be defined as follows.
```ruby
require 'roar/json/json_api'
module SongsRepresenter
- include Roar::JSON::JsonApi
+ include Roar::JSON::JSONAPI
type :songs
property :id
property :title
end