README.md in rails-auth-0.2.0 vs README.md in rails-auth-0.3.0
- old
+ new
@@ -404,13 +404,49 @@
When an authorization error occurs, the `Rails::Auth::NotAuthorizedError`
exception is raised up the middleware chain. However, it's likely you would
prefer to show an error page than have an unhandled exception.
You can write your own middleware that catches `Rails::Auth::NotAuthorizedError`
-if you'd like. However, a default one is provided which renders a 403 response
-with a static page body if you find that helpful.
+if you'd like. However, this library includes two middleware for rescuing this
+exception for you and displaying an error page.
-To use it, add `Rails::Auth::ErrorPage::Middleware` to your app:
+#### Rails::Auth::ErrorPage::DebugMiddleware
+
+This middleware displays a detailed error page intended to help debug authorization errors:
+
+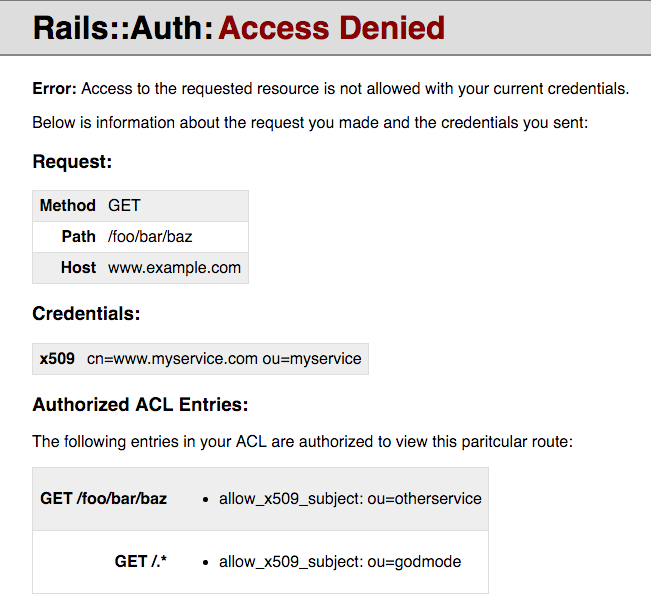
+
+Please be aware this middleware leaks information about your ACL to a potential attacker.
+Make sure you're ok with that information being public before using it. If you would like
+to avoid leaking that information, see `Rails::Auth::ErrorPage::Middleware` below.
+
+```ruby
+app = MyRackApp.new
+
+acl = Rails::Auth::ACL.from_yaml(
+ File.read("/path/to/my/acl.yaml")
+ matchers: { allow_x509_subject: Rails::Auth::X509::Matcher }
+)
+
+acl_auth = Rails::Auth::ACL::Middleware.new(app, acl: acl)
+
+x509_auth = Rails::Auth::X509::Middleware.new(
+ acl_auth,
+ ca_file: "/path/to/my/cabundle.pem"
+ cert_filters: { 'X-SSL-Client-Cert' => :pem },
+ require_cert: true
+)
+
+error_page = Rails::Auth::ErrorPage::Middleware.new(x509_auth, acl: acl)
+
+run error_page
+```
+
+#### Rails::Auth::ErrorPage::Middleware
+
+This middleware catches `Rails::Auth::NotAuthorizedError` and renders a given static HTML file,
+e.g. the 403.html file which ships with Rails. It will not give detailed errors to your users,
+but it also won't leak information to an attacker.
```ruby
app = MyRackApp.new
acl = Rails::Auth::ACL.from_yaml(