README.md in lhm-1.1.0 vs README.md in lhm-1.2.0
- old
+ new
@@ -1,6 +1,6 @@
-# Large Hadron Migrator [][4]
+# Large Hadron Migrator [][4]
Rails style database migrations are a useful way to evolve your data schema in
an agile manner. Most Rails projects start like this, and at first, making
changes is fast and easy.
@@ -20,11 +20,11 @@
is great if you are using this engine, but only solves half the problem.
At SoundCloud we started having migration pains quite a while ago, and after
looking around for third party solutions, we decided to create our
own. We called it Large Hadron Migrator, and it is a gem for online
-ActiveRecord migrations.
+ActiveRecord and DataMapper migrations.
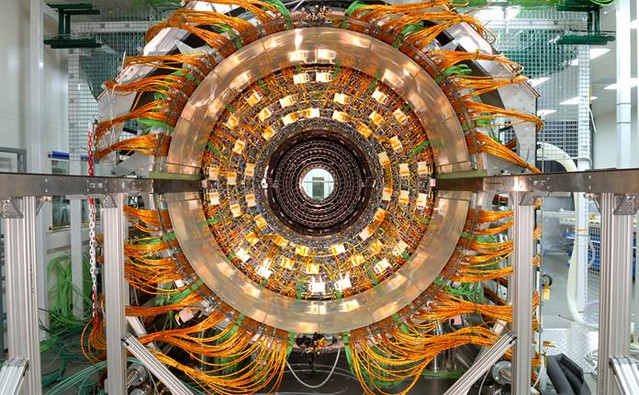
[The Large Hadron collider at CERN](http://en.wikipedia.org/wiki/Large_Hadron_Collider)
@@ -33,23 +33,27 @@
The basic idea is to perform the migration online while the system is live,
without locking the table. In contrast to [OAK][0] and the
[facebook tool][1], we only use a copy table and triggers.
The Large Hadron is a test driven Ruby solution which can easily be dropped
-into an ActiveRecord migration. It presumes a single auto incremented
-numerical primary key called id as per the Rails convention. Unlike the
-[twitter solution][2], it does not require the presence of an indexed
+into an ActiveRecord or DataMapper migration. It presumes a single auto
+incremented numerical primary key called id as per the Rails convention. Unlike
+the [twitter solution][2], it does not require the presence of an indexed
`updated_at` column.
## Requirements
Lhm currently only works with MySQL databases and requires an established
-ActiveRecord connection.
+ActiveRecord or DataMapper connection.
It is compatible and [continuously tested][4] with Ruby 1.8.7 and Ruby 1.9.x,
-ActiveRecord 2.3.x and 3.x as well as mysql and mysql2 adapters.
+ActiveRecord 2.3.x and 3.x (mysql and mysql2 adapters), as well as DataMapper
+1.2 (dm-mysql-adapter).
+Lhm also works with dm-master-slave-adapter, it'll bind to the master before
+running the migrations.
+
## Installation
Install it via `gem install lhm` or add `gem "lhm"` to your Gemfile.
## Usage
@@ -64,10 +68,14 @@
:adapter => 'mysql',
:host => '127.0.0.1',
:database => 'lhm'
)
+# or with DataMapper
+Lhm.setup(DataMapper.setup(:default, 'mysql://127.0.0.1/lhm'))
+
+# and migrate
Lhm.change_table :users do |m|
m.add_column :arbitrary, "INT(12)"
m.add_index [:arbitrary_id, :created_at]
m.ddl("alter table %s add column flag tinyint(1)" % m.name)
end
@@ -89,29 +97,57 @@
end
def self.down
Lhm.change_table :users do |m|
m.remove_index [:arbitrary_id, :created_at]
- m.remove_column :arbitrary)
+ m.remove_column :arbitrary
end
end
end
```
+Using dm-migrations, you'd define all your migrations as follows, and then call
+`migrate_up!` or `migrate_down!` as normal.
+
+```ruby
+require 'dm-migrations/migration_runner'
+require 'lhm'
+
+migration 1, :migrate_users do
+ up do
+ Lhm.change_table :users do |m|
+ m.add_column :arbitrary, "INT(12)"
+ m.add_index [:arbitrary_id, :created_at]
+ m.ddl("alter table %s add column flag tinyint(1)" % m.name)
+ end
+ end
+
+ down do
+ Lhm.change_table :users do |m|
+ m.remove_index [:arbitrary_id, :created_at]
+ m.remove_column :arbitrary
+ end
+ end
+end
+```
+
+**Note:** Lhm won't delete the old, leftover table. This is on purpose, in order
+to prevent accidental data loss.
+
## Table rename strategies
There are two different table rename strategies available: LockedSwitcher and
AtomicSwitcher.
-For all setups which use replication and a MySQL version
-affected by the the [binlog bug #39675](http://bugs.mysql.com/bug.php?id=39675),
-we recommend the LockedSwitcher strategy to avoid replication issues. This
-strategy locks the table being migrated and issues two ALTER TABLE statements.
-The AtomicSwitcher uses a single atomic RENAME TABLE query and should be favored
-in setups which do not suffer from the mentioned replication bug.
+The LockedSwitcher strategy locks the table being migrated and issues two ALTER TABLE statements.
+The AtomicSwitcher uses a single atomic RENAME TABLE query and is the favored solution.
-Lhm chooses the strategy automatically based on the used MySQL server version,
-but you can override the behavior with an option:
+Lhm chooses AtomicSwitcher if no strategy is specified, **unless** your version of MySQL is
+affected by [binlog bug #39675](http://bugs.mysql.com/bug.php?id=39675). If your version is
+affected, Lhm will raise an error if you don't specify a strategy. You're recommended
+to use the LockedSwitcher in these cases to avoid replication issues.
+
+To specify the strategy in your migration:
```ruby
Lhm.change_table :users, :atomic_switch => true do |m|
# ...
end