README.md in ember-cli-rails-0.3.5 vs README.md in ember-cli-rails-0.4.0
- old
+ new
@@ -1,27 +1,27 @@
-# EmberCLI Rails
+# Ember CLI Rails
-EmberCLI Rails is an integration story between (surprise suprise) EmberCLI and
+Ember CLI Rails is an integration story between (surprise surprise) Ember CLI and
Rails 3.1 and up. It is designed to provide an easy way to organize your Rails backed
-EmberCLI application with a specific focus on upgradeability. Rails and Ember
-[slash EmberCLI] are maintained by different teams with different goals. As
+Ember CLI application with a specific focus on upgradeability. Rails and Ember
+[slash Ember CLI] are maintained by different teams with different goals. As
such, we believe that it is important to ensure smooth upgrading of both
aspects of your application.
A large contingent of Ember developers use Rails. And Rails is awesome. With
the upcoming changes to Ember 2.0 and the Ember community's desire to unify
-around EmberCLI it is now more important than ever to ensure that Rails and
-EmberCLI can coexist and development still be fun!
+around Ember CLI it is now more important than ever to ensure that Rails and
+Ember CLI can coexist and development still be fun!
To this end we have created a minimum set of features (which we will outline
below) to allow you keep your Rails workflow while minimizing the risk of
upgrade pain with your Ember build tools.
For example, end-to-end tests with frameworks like Cucumber should just work.
You should still be able leverage the asset pipeline, and all the conveniences
that Rails offers. And you should get all the new goodies like ES6 modules and
-EmberCLI addons too! Without further ado, let's get in there!
+Ember CLI addons too! Without further ado, let's get in there!
## Installation
Firstly, you'll have to include the gem in your `Gemfile` and `bundle install`
@@ -44,16 +44,19 @@
end
```
##### options
-- app - this represents the name of the EmberCLI application.
+- `app` - this represents the name of the Ember CLI application.
-- path - the path, where your EmberCLI applications is located. The default
+- `build_timeout` - seconds to allow Ember to build the application before
+ timing out
+
+- `path` - the path where your Ember CLI application is located. The default
value is the name of your app in the Rails root.
-- enable - a lambda that accepts each requests' path. The default value is a
+- `enable` - a lambda that accepts each request's path. The default value is a
lambda that returns `true`.
```ruby
EmberCLI.configure do |c|
c.app :adminpanel # path is "<your-rails-root>/adminpanel"
@@ -61,24 +64,28 @@
path: "/path/to/your/ember-cli-app/on/disk",
enable: -> path { path.starts_with?("/app/") }
end
```
-Once you've updated your initializer to taste, you need to install the
-[ember-cli-rails-addon](https://github.com/rondale-sc/ember-cli-rails-addon).
+Once you've updated your initializer to taste, install Ember CLI if it is not already installed, and use it to generate your Ember CLI app in the location/s specified in the initializer. For example:
-For each of your EmberCLI applications install the addon with:
+```sh
+cd frontend
+ember init
+```
+You will also need to install the [ember-cli-rails-addon](https://github.com/rondale-sc/ember-cli-rails-addon). For each of your Ember CLI applications, run:
+
```sh
-npm install --save-dev ember-cli-rails-addon@0.0.11
+npm install --save-dev ember-cli-rails-addon@0.0.13
```
-And that's it!
+And that's it! You should now be able to start up your Rails server and see your Ember CLI app.
-### Multiple EmberCLI apps
+### Multiple Ember CLI apps
-In the initializer you may specify multiple EmberCLI apps, each of which can be
+In the initializer you may specify multiple Ember CLI apps, each of which can be
referenced with the view helper independently. You'd accomplish this like so:
```ruby
EmberCLI.configure do |c|
c.app :frontend
@@ -86,39 +93,128 @@
end
```
## Usage
-You render your EmberCLI app by including the corresponding JS/CSS tags in whichever
-Rails view you'd like the Ember app to appear.
+First, specify in your controller that you don't want to render the layout
+(since EmberCLI's `index.html` is a fully-formed HTML document):
-For example, if you had the following Rails app
+```rb
+# app/controllers/application.rb
+class ApplicationController < ActionController::Base
+ def index
+ render layout: false
+ end
+end
+```
+To render the EmberCLI generated `index.html` into the view,
+use the `include_ember_index_html` helper:
+
+
+```erb
+<!-- /app/views/application/index.html.erb -->
+<%= include_ember_index_html :frontend %>
+```
+
+To inject markup into page, pass in a block that accepts the `head`, and
+(optionally) the `body`:
+
+```erb
+<!-- /app/views/application/index.html.erb -->
+<%= include_ember_index_html :frontend do |head| %>
+ <%= head.append do %>
+ <%= csrf_meta_tags %>
+ <% end %>
+<% end %>
+```
+
+The asset paths will be replaced with asset pipeline generated paths.
+
+*NOTE*
+
+This helper **requires** that the `index.html` file exists.
+
+If you see `Errno::ENOENT` errors in development, your requests are timing out
+before EmberCLI finishes compiling the application.
+
+To prevent race conditions, increase your `build_timeout` to ensure that the
+build finishes before your request is processed.
+
+### Rendering the EmberCLI generated JS and CSS
+
+In addition to rendering the EmberCLI generated `index.html`, you can inject the
+`<script>` and `<link>` tags into your Rails generated views:
+
+```erb
+<!-- /app/views/application/index.html.erb -->
+<%= include_ember_script_tags :frontend %>
+<%= include_ember_stylesheet_tags :frontend %>
+```
+
+### Other routes
+
+Rendering Ember applications at routes other than `/` requires additional setup to avoid an Ember `UnrecognizedURLError`.
+
+For instance, if you had Ember applications named `:frontend` and `:admin_panel` and you wanted to serve them at `/frontend` and `/admin_panel`, you would set up the following Rails routes:
+
```rb
# /config/routes.rb
Rails.application.routes.draw do
root 'application#index'
+ get 'frontend' => 'frontend#index'
+ get 'admin_panel' => 'admin_panel#index'
end
-# /app/controllers/application_controller.rb
-class ApplicationController < ActionController::Base
+# /app/controllers/frontend_controller.rb
+class FrontendController < ActionController::Base
def index
render :index
end
end
+
+# /app/controllers/admin_panel_controller.rb
+class AdminPanelController < ActionController::Base
+ def index
+ render :index
+ end
+end
```
-and if you had created an Ember app `:frontend` in your initializer, then you
-could render your app at the `/` route with the following view:
+Additionally, you would have to modify each Ember app's `baseURL` to point to the correct route:
-```erb
-<!-- /app/views/application/index.html.erb -->
-<%= include_ember_script_tags :frontend %>
-<%= include_ember_stylesheet_tags :frontend %>
+```javascript
+/* /app/frontend/config/environment.js */
+module.exports = function(environment) {
+ var ENV = {
+ modulePrefix: 'frontend',
+ environment: environment,
+ baseURL: '/frontend', // originally '/'
+ ...
+ }
+}
+
+/* /app/admin_panel/config/environment.js */
+module.exports = function(environment) {
+ var ENV = {
+ modulePrefix: 'admin_panel',
+ environment: environment,
+ baseURL: '/admin_panel', // originally '/'
+ ...
+ }
+}
```
+Lastly, you would configure each app's `router.js` file so that `rootURL` points to the `baseURL` you just created:
-Your Ember application will now be served at the `/` route.
+```javascript
+/* app/frontend/app/router.js */
+var Router = Ember.Router.extend({
+ rootURL: config.baseURL, // add this line
+ location: config.locationType
+});
+```
+Repeat for `app/admin_panel/app/router.js`. Now your Ember apps will render properly at the alternative routes.
## CSRF Tokens
Your Rails controllers, by default, are expecting a valid authenticity token to be submitted with non-`GET` requests.
Without it you'll receive a `422 Unprocessable Entity` error, specifically: `ActionController::InvalidAuthenticityToken`.
@@ -165,13 +261,25 @@
example, `/ember-tests`) and the name of the Ember app.
For example, to view tests of the `frontend` app, visit
`http://localhost:3000/ember-tests/frontend`.
+## Serving from multi-process servers in development
+
+If you're using a multi-process server ([Puma], [Unicorn], etc.) in development,
+make sure it's configured to run a single worker process.
+
+Without restricting the server to a single process, [it is possible for multiple
+EmberCLI runners to clobber each others' work][#94].
+
+[Puma]: https://github.com/puma/puma
+[Unicorn]: https://rubygems.org/gems/unicorn
+[#94]: https://github.com/thoughtbot/ember-cli-rails/issues/94#issuecomment-77627453
+
## Enabling LiveReload
-In order to get LiveReload up and running with EmberCLI Rails, you can install
+In order to get LiveReload up and running with Ember CLI Rails, you can install
[guard](https://github.com/guard/guard) and
[guard-livereload](https://github.com/guard/guard-livereload) gems, run `guard
init` and then add the following to your `Guardfile`.
```ruby
@@ -180,16 +288,16 @@
watch %r{your-appname/app/\w+/.+\.(js|hbs|html|css|<other-extensions>)}
# ...
end
```
-This tells Guard to watch your EmberCLI app for any changes to the JavaScript,
+This tells Guard to watch your Ember CLI app for any changes to the JavaScript,
Handlebars, HTML, or CSS files within `app` path. Take note that other
extensions can be added to the line (such as `coffee` for CoffeeScript) to
watch them for changes as well.
-*NOTE:* EmberCLI creates symlinks in `your-appname/tmp` directory, which cannot
+*NOTE:* Ember CLI creates symlinks in `your-appname/tmp` directory, which cannot
be handled properly by Guard. This might lead to performance issues on some
platforms (most notably on OSX), as well as warnings being printed by latest
versions of Guard. As a work-around, one might use
[`directories`](https://github.com/guard/guard/wiki/Guardfile-DSL---Configuring-Guard#directories)
option, explicitly specifying directories to watch, e.g. adding the following
@@ -200,66 +308,62 @@
directories %w[app config lib spec your-appname/app]
```
## Heroku
-In order to deploy EmberCLI Rails app to Heroku:
+To configure your Ember CLI Rails app to be ready to deploy on Heroku:
-First, enable Heroku Multi Buildpack by running the following command:
+1. Run `rails g ember-cli:heroku` generator
+1. [Add the NodeJS buildpack][buildpack] and configure NPM to include the
+ `bower` dependency's executable file.
```sh
-heroku buildpacks:set https://github.com/heroku/heroku-buildpack-multi
+heroku buildpacks:add --index 1 https://github.com/heroku/heroku-buildpack-nodejs
+heroku config:set NPM_CONFIG_PRODUCTION=false
```
-Next, specify which buildpacks to use by creating a `.buildpacks` file in the project root containing:
+You should be ready to deploy.
-```
-https://github.com/heroku/heroku-buildpack-nodejs
-https://github.com/heroku/heroku-buildpack-ruby
-```
+**NOTE** Run the generator each time you introduce additional EmberCLI
+applications into the project.
-Add `rails_12factor` gem to your production group in Gemfile, then run `bundle
-install`:
+[buildpack]: https://devcenter.heroku.com/articles/using-multiple-buildpacks-for-an-app#adding-a-buildpack
-```ruby
-gem "rails_12factor", group: :production
-```
+## Capistrano
-Add a `package.json` file containing `{}` to the root of your Rails project.
-This is to make sure it'll be detected by the NodeJS buildpack.
+To deploy an EmberCLI-Rails application with Capistrano, make sure your
+EmberCLI app's `package.json` file includes the `bower` package as a development
+dependency:
-Make sure you have `bower` as a npm dependency of your ember-cli app.
-
-Add a `postinstall` task to your EmberCLI app's `package.json`. This will
-ensure that during the deployment process, Heroku will install all dependencies
-found in both `node_modules` and `bower_components`.
-
-```javascript
+```json
{
- # ...
- "scripts": {
- # ...
- "postinstall": "node_modules/bower/bin/bower install"¬
+ "devDependencies": {
+ "bower": "*"
}
}
```
-ember-cli-rails adds your ember apps' build process to the rails asset compilation process.
+## Experiencing Slow Build/Deploy Times?
+Remove `ember-cli-uglify` from your `package.json` file, and run
+`npm remove ember-cli-uglify`. This will improve your build/deploy
+time by about 10 minutes.
-Now you should be ready to deploy.
+The reason build/deploy times were slow is because ember uglified the JS and
+then added the files to the asset pipeline. Rails would then try and uglify
+the JS again, and this would be considerably slower than normal.
## Additional Information
-When running in the development environment, EmberCLI Rails runs `ember build`
+When running in the development environment, Ember CLI Rails runs `ember build`
with the `--output-path` and `--watch` flags on. The `--watch` flag tells
-EmberCLI to watch for file system events and rebuild when an EmberCLI file is
+Ember CLI to watch for file system events and rebuild when an Ember CLI file is
changed. The `--output-path` flag specifies where the distribution files will
-be put. EmberCLI Rails does some fancy stuff to get it into your asset path
+be put. Ember CLI Rails does some fancy stuff to get it into your asset path
without polluting your git history. Note that for this to work, you must have
`config.consider_all_requests_local = true` set in
`config/environments/development.rb`, otherwise the middleware responsible for
-building EmberCLI will not be enabled.
+building Ember CLI will not be enabled.
Alternatively, if you want to override the default behavior in any given Rails
environment, you can manually set the `config.use_ember_middleware` and
`config.use_ember_live_recompilation` flags in the environment-specific config
file.
@@ -288,10 +392,21 @@
`RAILS_ENV` will be absent in production builds.
[ember-cli-mirage]: http://ember-cli-mirage.com/docs/latest/
+### `SKIP_EMBER`
+
+To disable asset compilation entirely, set an environment variable
+`SKIP_EMBER=1`.
+
+This can be useful when an application's frontend is developed locally with
+EmberCLI-Rails, but deployed separately (for example, with
+[ember-cli-deploy][ember-cli-deploy]).
+
+[ember-cli-deploy]: https://github.com/ember-cli/ember-cli-deploy
+
#### Ember Dependencies
Ember has several dependencies. Some of these dependencies might already be
present in your asset list. For example jQuery is bundled in `jquery-rails` gem.
If you have the jQuery assets included on your page you may want to exclude them
@@ -305,12 +420,56 @@
end
```
jQuery and Handlebars are the main use cases for this flag.
+## Ruby and Rails support
+
+This project supports:
+
+* Ruby versions `>= 2.1.0`
+* Rails `3.2.x` and `>=4.1.x`.
+
+To learn more about supported versions and upgrades, read the [upgrading guide].
+
+[upgrading guide]: /UPGRADING.md
+
## Contributing
-1. Fork it (https://github.com/rwz/ember-cli-rails/fork)
-2. Create your feature branch (`git checkout -b my-new-feature`)
-3. Commit your changes (`git commit -am 'Add some feature'`)
-4. Push to the branch (`git push origin my-new-feature`)
-5. Create a new Pull Request
+See the [CONTRIBUTING] document.
+Thank you, [contributors]!
+
+ [CONTRIBUTING]: CONTRIBUTING.md
+ [contributors]: https://github.com/thoughtbot/ember-cli-rails/graphs/contributors
+
+## License
+
+Open source templates are Copyright (c) 2015 thoughtbot, inc.
+It contains free software that may be redistributed
+under the terms specified in the [LICENSE] file.
+
+[LICENSE]: /LICENSE.txt
+
+## About
+
+ember-cli-rails was originally created by
+[Pavel Pravosud][rwz] and
+[Jonathan Jackson][rondale-sc].
+
+ember-cli-rails is maintained by [Sean Doyle][seanpdoyle] and [Jonathan
+Jackson][rondale-sc].
+
+[rwz]: https://github.com/rwz
+[rondale-sc]: https://github.com/rondale-sc
+[seanpdoyle]: https://github.com/seanpdoyle
+
+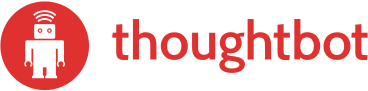
+
+ember-cli-rails is maintained and funded by thoughtbot, inc.
+The names and logos for thoughtbot are trademarks of thoughtbot, inc.
+
+We love open source software!
+See [our other projects][community]
+or [hire us][hire] to help build your product.
+
+ [community]: https://thoughtbot.com/community?utm_source=github
+ [hire]: https://thoughtbot.com/hire-us?utm_source=github