# Writing your own extension Check out the [example](https://nhnent.github.io/tui.editor/api/latest/tutorial-example12-writing-extension.html). ## Prepare ### Bower Let's start by setting up a bower and install *tui-editor* as development dependency. Of course, you can also set the webpack, babel, etc. according to your needs. ```sh bower init bower install -D tui-editor ``` ### Editor Dependencies Create a `index.html` in a project root directory. Then include dependencies like below to load *tui-editor*. ```html
... ``` ### Initialize Editor Now create a `div` for the editor in `body`, initialize it. ```html ... ... ``` Run the local server like [http-server](https://www.npmjs.com/package/http-server) to see if the code you've written so far works well. You should see the editor runs like below. 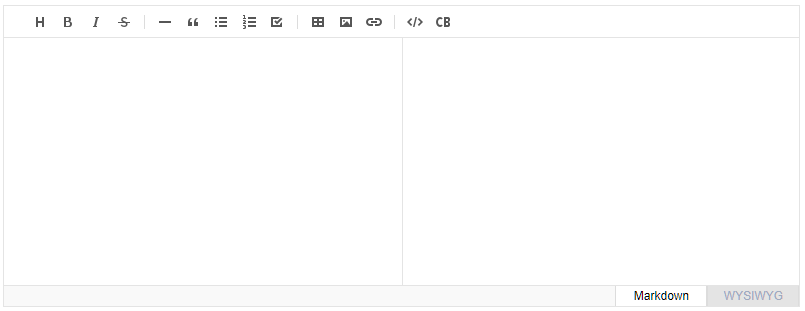 ## Define Extension Create `tui-editor-extYoutube.js` in project root and fill the code below. `defineExtension` static function takes **extension name** and **extension init function** as a callback function. The function will be executed when editor instances initialized. ```js tui.Editor.defineExtension('youtube', function() { console.log('youtube extension initialized'); }); ``` ## Enable Extension Include `tui-editor-extYoutube.js` in `index.html`. To enable the extension, pass the name of the extension 'youtube' to the exts option as a string. ```html ``` Refresh the browser and open the developer console to see if the code you've written so far works well. You should see 'youtube extension initialized' text on the developer console. ## codeBlockManager `CodeBlockManager` can transform *code block* in markdown while [markdown-it](https://github.com/markdown-it/markdown-it) transform markdown to HTML. Call `setReplace` with the *language* and a *callback* function to define what/how the language codeblock is converted. Beware, **tui-editor** shares one **markdown-it** instance. So It will affect all editor instances. ```js var Editor = tui.Editor; Editor.defineExtension('youtube', function() { Editor.codeBlockManager.setReplacer('youtube', function (code) { return 'play youtube id: ' + code; }); }); ``` Refresh the brwoser and this time add code block ` ```youtube ` with some text in markdown editor. Do you see the text on the *preview* transformed? ## youtube player We're almost done. Now implement real code to render youtube. Embeding Youtube can be done by adding `iframe`. But **tui-editor** will eliminate `iframe` due to security reason. So We're going to pass a `div` and add `iframe` in next tick. ```js var Editor = tui.Editor; Editor.defineExtension('youtube', function() { Editor.codeBlockManager.setReplacer('youtube', function(youtubeId) { var wrapperId = 'yt' + Math.random().toString(36).substr(2, 10); setTimeout(renderYoutube.bind(null, wrapperId, youtubeId), 0); return ''; }); }); function renderYoutube(wrapperId, youtubeId) { var el = document.querySelector('#' + wrapperId); el.innerHTML = ''; } ``` Reload the browser and write below in *markdown view*. ````markdown ```youtube XGSy3_Czz8k ``` ```` 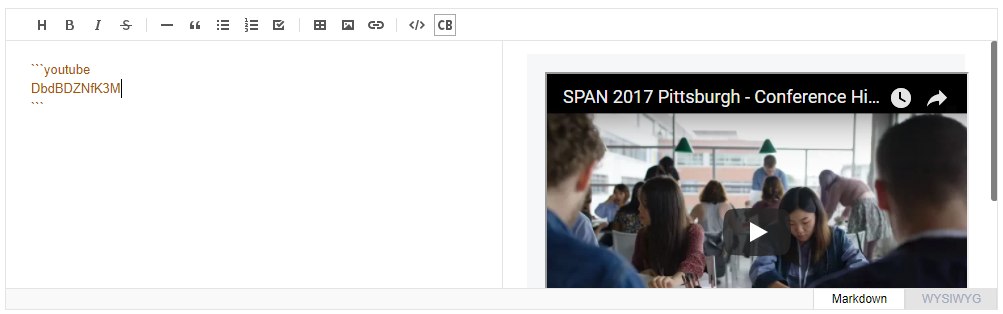 YEY! It works! By the way, this code is only for demonstraition to show you how extensions work. This code has vulnerabilities. You should at least sanitize user inputs. ## UMD This is final. We accessed through namespace `tui.Editor`. To provide ability to work with tools like webapck, wrap it with *umd*. And then you can publish to npm, bower. ```js (function(root, factory) { if (typeof define === 'function' && define.amd) { define(['tui-editor'], factory); } else if (typeof exports === 'object') { factory(require('tui-editor')); } else { factory(root['tui']['Editor']); } })(this, function(Editor) { Editor.defineExtension('youtube', function() { Editor.codeBlockManager.setReplacer('youtube', function(youtubeId) { var wrapperId = 'yt' + Math.random().toString(36).substr(2, 10); setTimeout(renderYoutube.bind(null, wrapperId, youtubeId), 0); return ''; }); }); function renderYoutube(wrapperId, youtubeId) { var el = document.querySelector('#' + wrapperId); el.innerHTML = ''; } }); ``` Send us your extension link, we are happy to link your extension repository. 😄