\n\n\n\n## Copyright and license\n\nCode and documentation copyright 2011-2015 Twitter, Inc. Code released under [the MIT license](https://github.com/twbs/bootstrap/blob/master/LICENSE). Docs released under [Creative Commons](https://github.com/twbs/bootstrap/blob/master/docs/LICENSE).\n",
"readmeFilename": "README.md",
"_id": "bootstrap@3.3.5",
"_from": "bootstrap@3.3.5",
"realName": "bootstrap",
"dependencies": {},
"extraneous": false,
"path": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media/node_modules/pui-css-media/node_modules/bootstrap",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media/node_modules/pui-css-media/node_modules/bootstrap",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
}
},
"readme": "# pui-css-media\n\nA CSS media component that can be installed via this npm package. The package provides all of the\nCSS you need to use the component.\n\n\n\n## Installation\n\nTo install the package, from the command line, type:\n\n```\nnpm install pui-css-media\n```\n\n## Usage\n\n```html\n\n\n\n```\n\n\nYou can find more examples of the media component in the [pui style guide](http://styleguide.pivotal.io/)\n\n*****************************************\n\nThis is a component of Pivotal UI. It is a Pivotal specific implementation of Bootstrap.\n\n[Styleguide](http://styleguide.pivotal.io)\n[Github](https://github.com/pivotal-cf/pivotal-ui)\n\n(c) Copyright 2015 Pivotal Software, Inc. All Rights Reserved.",
"readmeFilename": "README.md",
"_id": "pui-css-media@3.0.0-alpha.2",
"_from": "pui-css-media@3.0.0-alpha.2",
"realName": "pui-css-media",
"path": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media/node_modules/pui-css-media",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media/node_modules/pui-css-media",
"parent": "[Circular]",
"depth": 2,
"peerDependencies": {}
}
},
"readme": "# pui-react-media\n\nReact components for laying out images/audio/video next to text content\n\nPivotal UI React ([GitHub](https://github.com/pivotal-cf/pivotal-ui-react), [npm](https://www.npmjs.com/browse/keyword/pivotal%20ui%20modularized)) is a collection of [React](https://facebook.github.io/react/) components for rapidly building and prototyping UIs.\n\nThis component requires React v0.13\n\nSee the [Pivotal UI Styleguide](http://styleguide.pivotal.io/) for fully rendered examples.\n\n## Components\n\n### Media\n\nDisplays a media object (images, video, or audio) to the left or right of a block of content\n\n```js\nvar Media = require('pui-react-media').Media;\nvar Image = require('pui-react-image').Image;\nvar MyComponent = React.createClass({\n render() {\n var image = ;\n return (Content );\n }\n});\n```\n\n#### Properties\n\n- `leftImage`\n - `Element`: ``, ``, or `` to be shown left of the content\n\n- `rightImage`\n - `Element`: ``, ``, or `` to be shown right of the content\n\n- `vAlign`\n - `String`: One of `middle` or `bottom`--if given, re-positions the content vertically\n\n- `stackSize`\n - `String`: One of `xsmall`, `small`, `medium`, or `large`--if given, sets the breakpoint at which the media object stacks\n\n- `leftMediaSpacing`\n - `String`: One of `small`, `medium`, `large` (default), or `xlarge`--sets the amount of space between the left media and the content\n\n- `rightMediaSpacing`\n - `String`: One of `small`, `medium`, `large` (default), or `xlarge`--sets the amount of space between the right media and the content\n\n\n### Flag\n\nDisplays a media object (images, video, or audio) to the left or right of a block of content with vertical centering\n\n\n\n#### Properties\n\n- `leftImage`\n - `Element`: ``, ``, or `` to be shown left of the content\n\n- `rightImage`\n - `Element`: ``, ``, or `` to be shown right of the content\n\n- `stackSize`\n - `String`: One of `xsmall`, `small`, `medium`, or `large`--if given, sets the breakpoint at which the media object stacks\n\n- `leftMediaSpacing`\n - `String`: One of `small`, `medium`, `large` (default), or `xlarge`--sets the amount of space between the left media and the content\n\n- `rightMediaSpacing`\n - `String`: One of `small`, `medium`, `large` (default), or `xlarge`--sets the amount of space between the right media and the content\n\n\n*****************************************\n\n(c) Copyright 2015 Pivotal Software, Inc. All Rights Reserved.\n",
"readmeFilename": "README.md",
"_id": "pui-react-media@3.0.0-alpha.2",
"_from": "pui-react-media@3.0.0-alpha.2",
"realName": "pui-react-media",
"path": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/pui-react-media",
"parent": "[Circular]",
"depth": 1,
"extraneous": false
},
"react": {
"name": "react",
"description": "React is a JavaScript library for building user interfaces.",
"version": "0.14.7",
"keywords": [
"react"
],
"homepage": "https://facebook.github.io/react/",
"bugs": {
"url": "https://github.com/facebook/react/issues"
},
"license": "BSD-3-Clause",
"files": [
"LICENSE",
"PATENTS",
"addons.js",
"react.js",
"addons/",
"dist/",
"lib/"
],
"main": "react.js",
"repository": {
"type": "git",
"url": "git://github.com/facebook/react"
},
"engines": {
"node": ">=0.10.0"
},
"dependencies": {
"envify": {
"name": "envify",
"version": "3.4.0",
"description": "Selectively replace Node-style environment variables with plain strings.",
"main": "index.js",
"scripts": {
"test": "node test.js | tap-spec"
},
"bin": {
"envify": "bin/envify"
},
"repository": {
"type": "git",
"url": "git://github.com/hughsk/envify.git"
},
"author": {
"name": "Hugh Kennedy",
"email": "hughskennedy@gmail.com",
"url": "http://hughskennedy.com/"
},
"license": "MIT",
"devDependencies": {
"tap-spec": "^1.0.1",
"tape": "~2.3.2"
},
"dependencies": {
"through": {
"name": "through",
"version": "2.3.8",
"description": "simplified stream construction",
"main": "index.js",
"scripts": {
"test": "set -e; for t in test/*.js; do node $t; done"
},
"devDependencies": {
"stream-spec": "~0.3.5",
"tape": "~2.3.2",
"from": "~0.1.3"
},
"keywords": [
"stream",
"streams",
"user-streams",
"pipe"
],
"author": {
"name": "Dominic Tarr",
"email": "dominic.tarr@gmail.com",
"url": "dominictarr.com"
},
"license": "MIT",
"repository": {
"type": "git",
"url": "https://github.com/dominictarr/through.git"
},
"homepage": "https://github.com/dominictarr/through",
"testling": {
"browsers": [
"ie/8..latest",
"ff/15..latest",
"chrome/20..latest",
"safari/5.1..latest"
],
"files": "test/*.js"
},
"readme": "#through\n\n[](http://travis-ci.org/dominictarr/through)\n[](https://ci.testling.com/dominictarr/through)\n\nEasy way to create a `Stream` that is both `readable` and `writable`. \n\n* Pass in optional `write` and `end` methods.\n* `through` takes care of pause/resume logic if you use `this.queue(data)` instead of `this.emit('data', data)`.\n* Use `this.pause()` and `this.resume()` to manage flow.\n* Check `this.paused` to see current flow state. (`write` always returns `!this.paused`).\n\nThis function is the basis for most of the synchronous streams in \n[event-stream](http://github.com/dominictarr/event-stream).\n\n``` js\nvar through = require('through')\n\nthrough(function write(data) {\n this.queue(data) //data *must* not be null\n },\n function end () { //optional\n this.queue(null)\n })\n```\n\nOr, can also be used _without_ buffering on pause, use `this.emit('data', data)`,\nand this.emit('end')\n\n``` js\nvar through = require('through')\n\nthrough(function write(data) {\n this.emit('data', data)\n //this.pause() \n },\n function end () { //optional\n this.emit('end')\n })\n```\n\n## Extended Options\n\nYou will probably not need these 99% of the time.\n\n### autoDestroy=false\n\nBy default, `through` emits close when the writable\nand readable side of the stream has ended.\nIf that is not desired, set `autoDestroy=false`.\n\n``` js\nvar through = require('through')\n\n//like this\nvar ts = through(write, end, {autoDestroy: false})\n//or like this\nvar ts = through(write, end)\nts.autoDestroy = false\n```\n\n## License\n\nMIT / Apache2\n",
"readmeFilename": "readme.markdown",
"bugs": {
"url": "https://github.com/dominictarr/through/issues"
},
"_id": "through@2.3.8",
"_from": "through@~2.3.4",
"realName": "through",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/through",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/through",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
},
"jstransform": {
"name": "jstransform",
"version": "10.1.0",
"description": "A simple AST visitor-based JS transformer",
"contributors": [
{
"name": "Jeff Morrison",
"email": "jeffmo@fb.com"
}
],
"main": "src/jstransform",
"repository": {
"type": "git",
"url": "git@github.com:facebook/jstransform.git"
},
"keywords": [
"transformer",
"compiler",
"syntax",
"visitor"
],
"dependencies": {
"base62": {
"author": {
"name": "Andrew Nesbitt",
"email": "andrewnez@gmail.com",
"url": "http://andrew-nesbitt.com/"
},
"name": "base62",
"description": "Javascript Base62 encode/decoder",
"keywords": [
"base-62"
],
"version": "0.1.1",
"repository": {
"type": "git",
"url": "git://github.com/andrew/base62.js.git"
},
"main": "base62.js",
"engines": {
"node": "*"
},
"scripts": {
"test": "mocha test"
},
"devDependencies": {
"mocha": "1.7.x"
},
"readme": "# Base62.js \n[](http://travis-ci.org/andrew/base62.js)\n\nA javascript Base62 encode/decoder for node.js\n\n## Install\n\n npm install base62\n\n## Usage\n\n Base62 = require('base62')\n Base62.encode(999) // 'g7'\n Base62.decode('g7') // 999\n\n## Development\n\nSource hosted at [GitHub](http://github.com/andrew/base62.js).\nReport Issues/Feature requests on [GitHub Issues](http://github.com/andrew/split/base62.js).\n\n### Note on Patches/Pull Requests\n\n * Fork the project.\n * Make your feature addition or bug fix.\n * Add tests for it. This is important so I don't break it in a future version unintentionally.\n * Send me a pull request. Bonus points for topic branches.\n\n## Copyright\n\nCopyright (c) 2012 Andrew Nesbitt. See [LICENSE](https://github.com/andrew/base62.js/blob/master/LICENSE) for details.\n",
"readmeFilename": "Readme.md",
"bugs": {
"url": "https://github.com/andrew/base62.js/issues"
},
"_id": "base62@0.1.1",
"_from": "base62@0.1.1",
"realName": "base62",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/base62",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/base62",
"parent": "[Circular]",
"depth": 4,
"peerDependencies": {}
},
"esprima-fb": {
"name": "esprima-fb",
"description": "Facebook-specific fork of the esprima project",
"homepage": "https://github.com/facebook/esprima/tree/fb-harmony",
"main": "esprima.js",
"bin": {
"esparse": "./bin/esparse.js",
"esvalidate": "./bin/esvalidate.js"
},
"version": "13001.1001.0-dev-harmony-fb",
"files": [
"bin",
"test/run.js",
"test/runner.js",
"test/test.js",
"test/compat.js",
"test/reflect.js",
"esprima.js"
],
"engines": {
"node": ">=0.4.0"
},
"author": {
"name": "Ariya Hidayat",
"email": "ariya.hidayat@gmail.com"
},
"maintainers": [
{
"name": "Jeff Morrison",
"email": "jeffmo@fb.com",
"url": "https://www.facebook.com/lbljeffmo"
}
],
"repository": {
"type": "git",
"url": "http://github.com/facebook/esprima.git"
},
"bugs": {
"url": "http://issues.esprima.org"
},
"licenses": [
{
"type": "BSD",
"url": "http://github.com/facebook/esprima/raw/master/LICENSE.BSD"
}
],
"devDependencies": {
"eslint": "~0.12.0",
"jscs": "~1.10.0",
"istanbul": "~0.2.6",
"escomplex-js": "1.0.0",
"complexity-report": "~1.1.1",
"regenerate": "~0.5.4",
"unicode-6.3.0": "~0.1.0",
"json-diff": "~0.3.1",
"commander": "~2.5.0"
},
"scripts": {
"generate-regex": "node tools/generate-identifier-regex.js",
"test": "node test/run.js && npm run lint && npm run coverage",
"lint": "npm run check-version && npm run eslint && npm run jscs && npm run complexity",
"check-version": "node tools/check-version.js",
"jscs": "jscs esprima.js test/*test.js",
"eslint": "node node_modules/eslint/bin/eslint.js esprima.js",
"complexity": "node tools/list-complexity.js && cr -s -l -w --maxcyc 18 esprima.js",
"coverage": "npm run analyze-coverage && npm run check-coverage",
"analyze-coverage": "node node_modules/istanbul/lib/cli.js cover test/runner.js",
"check-coverage": "node node_modules/istanbul/lib/cli.js check-coverage --statement 100 --branch 100 --function 100",
"benchmark": "node test/benchmarks.js",
"benchmark-quick": "node test/benchmarks.js quick"
},
"readme": "**Esprima** ([esprima.org](http://esprima.org), BSD license) is a high performance,\nstandard-compliant [ECMAScript](http://www.ecma-international.org/publications/standards/Ecma-262.htm)\nparser written in ECMAScript (also popularly known as\n[JavaScript](http://en.wikipedia.org/wiki/JavaScript>JavaScript)).\nEsprima is created and maintained by [Ariya Hidayat](http://twitter.com/ariyahidayat),\nwith the help of [many contributors](https://github.com/ariya/esprima/contributors).\n\n**Esprima-FB** is a fork of the [Harmony branch](https://github.com/ariya/esprima/tree/harmony) of Esprima that implements [JSX specification](https://github.com/facebook/jsx) on top of ECMAScript syntax.\n\n### Features\n\n- Full support for ECMAScript 5.1 ([ECMA-262](http://www.ecma-international.org/publications/standards/Ecma-262.htm))\n- Experimental support for ES6/Harmony (module, class, destructuring, ...)\n- Full support for [JSX syntax extensions](https://github.com/facebook/jsx).\n- Sensible [syntax tree format](https://github.com/facebook/jsx/blob/master/AST.md) compatible with Mozilla\n[Parser AST](https://developer.mozilla.org/en/SpiderMonkey/Parser_API)\n- Optional tracking of syntax node location (index-based and line-column)\n- Heavily tested (> 650 [unit tests](http://esprima.org/test/) with [full code coverage](http://esprima.org/test/coverage.html))\n- Ongoing support for ES6/Harmony (module, class, destructuring, ...)\n\n### Versioning rules\n\nIn order to follow semver rules and keep reference to original Esprima versions at the same time, we left 3 digits of each version part to refer to upstream harmony branch. We then take the most significant digit.\n\n**Example:** 4001.3001.0000-dev-harmony-fb aligns with 1.1.0-dev-harmony (aka 001.001.000-dev-harmony) in upstream, with our own changes on top.\n\nEsprima-FB serves as a **building block** for JSX language tools and transpiler implementations (such as [React](https://github.com/facebook/react) or [JSXDOM](https://github.com/vjeux/jsxdom)).\n\nEsprima-FB runs on many popular web browsers, as well as other ECMAScript platforms such as\n[Rhino](http://www.mozilla.org/rhino) and [Node.js](https://npmjs.org/package/esprima).\n\nFor more information on original Esprima, check the web site [esprima.org](http://esprima.org).\n",
"readmeFilename": "README.md",
"_id": "esprima-fb@13001.1001.0-dev-harmony-fb",
"_from": "esprima-fb@13001.1001.0-dev-harmony-fb",
"realName": "esprima-fb",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/esprima-fb",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/esprima-fb",
"parent": "[Circular]",
"depth": 4,
"peerDependencies": {}
},
"source-map": {
"name": "source-map",
"description": "Generates and consumes source maps",
"version": "0.1.31",
"homepage": "https://github.com/mozilla/source-map",
"author": {
"name": "Nick Fitzgerald",
"email": "nfitzgerald@mozilla.com"
},
"contributors": [
{
"name": "Tobias Koppers",
"email": "tobias.koppers@googlemail.com"
},
{
"name": "Duncan Beevers",
"email": "duncan@dweebd.com"
},
{
"name": "Stephen Crane",
"email": "scrane@mozilla.com"
},
{
"name": "Ryan Seddon",
"email": "seddon.ryan@gmail.com"
},
{
"name": "Miles Elam",
"email": "miles.elam@deem.com"
},
{
"name": "Mihai Bazon",
"email": "mihai.bazon@gmail.com"
},
{
"name": "Michael Ficarra",
"email": "github.public.email@michael.ficarra.me"
},
{
"name": "Todd Wolfson",
"email": "todd@twolfson.com"
},
{
"name": "Alexander Solovyov",
"email": "alexander@solovyov.net"
},
{
"name": "Felix Gnass",
"email": "fgnass@gmail.com"
},
{
"name": "Conrad Irwin",
"email": "conrad.irwin@gmail.com"
},
{
"name": "usrbincc",
"email": "usrbincc@yahoo.com"
},
{
"name": "David Glasser",
"email": "glasser@davidglasser.net"
},
{
"name": "Chase Douglas",
"email": "chase@newrelic.com"
},
{
"name": "Evan Wallace",
"email": "evan.exe@gmail.com"
},
{
"name": "Heather Arthur",
"email": "fayearthur@gmail.com"
}
],
"repository": {
"type": "git",
"url": "http://github.com/mozilla/source-map.git"
},
"directories": {
"lib": "./lib"
},
"main": "./lib/source-map.js",
"engines": {
"node": ">=0.8.0"
},
"licenses": [
{
"type": "BSD",
"url": "http://opensource.org/licenses/BSD-3-Clause"
}
],
"dependencies": {
"amdefine": {
"name": "amdefine",
"description": "Provide AMD's define() API for declaring modules in the AMD format",
"version": "1.0.0",
"homepage": "http://github.com/jrburke/amdefine",
"author": {
"name": "James Burke",
"email": "jrburke@gmail.com",
"url": "http://github.com/jrburke"
},
"license": "BSD-3-Clause AND MIT",
"repository": {
"type": "git",
"url": "https://github.com/jrburke/amdefine.git"
},
"main": "./amdefine.js",
"engines": {
"node": ">=0.4.2"
},
"readme": "# amdefine\n\nA module that can be used to implement AMD's define() in Node. This allows you\nto code to the AMD API and have the module work in node programs without\nrequiring those other programs to use AMD.\n\n## Usage\n\n**1)** Update your package.json to indicate amdefine as a dependency:\n\n```javascript\n \"dependencies\": {\n \"amdefine\": \">=0.1.0\"\n }\n```\n\nThen run `npm install` to get amdefine into your project.\n\n**2)** At the top of each module that uses define(), place this code:\n\n```javascript\nif (typeof define !== 'function') { var define = require('amdefine')(module) }\n```\n\n**Only use these snippets** when loading amdefine. If you preserve the basic structure,\nwith the braces, it will be stripped out when using the [RequireJS optimizer](#optimizer).\n\nYou can add spaces, line breaks and even require amdefine with a local path, but\nkeep the rest of the structure to get the stripping behavior.\n\nAs you may know, because `if` statements in JavaScript don't have their own scope, the var\ndeclaration in the above snippet is made whether the `if` expression is truthy or not. If\nRequireJS is loaded then the declaration is superfluous because `define` is already already\ndeclared in the same scope in RequireJS. Fortunately JavaScript handles multiple `var`\ndeclarations of the same variable in the same scope gracefully.\n\nIf you want to deliver amdefine.js with your code rather than specifying it as a dependency\nwith npm, then just download the latest release and refer to it using a relative path:\n\n[Latest Version](https://github.com/jrburke/amdefine/raw/latest/amdefine.js)\n\n### amdefine/intercept\n\nConsider this very experimental.\n\nInstead of pasting the piece of text for the amdefine setup of a `define`\nvariable in each module you create or consume, you can use `amdefine/intercept`\ninstead. It will automatically insert the above snippet in each .js file loaded\nby Node.\n\n**Warning**: you should only use this if you are creating an application that\nis consuming AMD style defined()'d modules that are distributed via npm and want\nto run that code in Node.\n\nFor library code where you are not sure if it will be used by others in Node or\nin the browser, then explicitly depending on amdefine and placing the code\nsnippet above is suggested path, instead of using `amdefine/intercept`. The\nintercept module affects all .js files loaded in the Node app, and it is\ninconsiderate to modify global state like that unless you are also controlling\nthe top level app.\n\n#### Why distribute AMD-style modules via npm?\n\nnpm has a lot of weaknesses for front-end use (installed layout is not great,\nshould have better support for the `baseUrl + moduleID + '.js' style of loading,\nsingle file JS installs), but some people want a JS package manager and are\nwilling to live with those constraints. If that is you, but still want to author\nin AMD style modules to get dynamic require([]), better direct source usage and\npowerful loader plugin support in the browser, then this tool can help.\n\n#### amdefine/intercept usage\n\nJust require it in your top level app module (for example index.js, server.js):\n\n```javascript\nrequire('amdefine/intercept');\n```\n\nThe module does not return a value, so no need to assign the result to a local\nvariable.\n\nThen just require() code as you normally would with Node's require(). Any .js\nloaded after the intercept require will have the amdefine check injected in\nthe .js source as it is loaded. It does not modify the source on disk, just\nprepends some content to the text of the module as it is loaded by Node.\n\n#### How amdefine/intercept works\n\nIt overrides the `Module._extensions['.js']` in Node to automatically prepend\nthe amdefine snippet above. So, it will affect any .js file loaded by your\napp.\n\n## define() usage\n\nIt is best if you use the anonymous forms of define() in your module:\n\n```javascript\ndefine(function (require) {\n var dependency = require('dependency');\n});\n```\n\nor\n\n```javascript\ndefine(['dependency'], function (dependency) {\n\n});\n```\n\n## RequireJS optimizer integration. \n\nVersion 1.0.3 of the [RequireJS optimizer](http://requirejs.org/docs/optimization.html)\nwill have support for stripping the `if (typeof define !== 'function')` check\nmentioned above, so you can include this snippet for code that runs in the\nbrowser, but avoid taking the cost of the if() statement once the code is\noptimized for deployment.\n\n## Node 0.4 Support\n\nIf you want to support Node 0.4, then add `require` as the second parameter to amdefine:\n\n```javascript\n//Only if you want Node 0.4. If using 0.5 or later, use the above snippet.\nif (typeof define !== 'function') { var define = require('amdefine')(module, require) }\n```\n\n## Limitations\n\n### Synchronous vs Asynchronous\n\namdefine creates a define() function that is callable by your code. It will\nexecute and trace dependencies and call the factory function *synchronously*,\nto keep the behavior in line with Node's synchronous dependency tracing.\n\nThe exception: calling AMD's callback-style require() from inside a factory\nfunction. The require callback is called on process.nextTick():\n\n```javascript\ndefine(function (require) {\n require(['a'], function(a) {\n //'a' is loaded synchronously, but\n //this callback is called on process.nextTick().\n });\n});\n```\n\n### Loader Plugins\n\nLoader plugins are supported as long as they call their load() callbacks\nsynchronously. So ones that do network requests will not work. However plugins\nlike [text](http://requirejs.org/docs/api.html#text) can load text files locally.\n\nThe plugin API's `load.fromText()` is **not supported** in amdefine, so this means\ntranspiler plugins like the [CoffeeScript loader plugin](https://github.com/jrburke/require-cs)\nwill not work. This may be fixable, but it is a bit complex, and I do not have\nenough node-fu to figure it out yet. See the source for amdefine.js if you want\nto get an idea of the issues involved.\n\n## Tests\n\nTo run the tests, cd to **tests** and run:\n\n```\nnode all.js\nnode all-intercept.js\n```\n\n## License\n\nNew BSD and MIT. Check the LICENSE file for all the details.\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/jrburke/amdefine/issues"
},
"_id": "amdefine@1.0.0",
"_from": "amdefine@>=0.0.4",
"scripts": {},
"realName": "amdefine",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/source-map/node_modules/amdefine",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/source-map/node_modules/amdefine",
"parent": "[Circular]",
"depth": 5,
"peerDependencies": {}
}
},
"devDependencies": {
"dryice": ">=0.4.8"
},
"scripts": {
"test": "node test/run-tests.js",
"build": "node Makefile.dryice.js"
},
"readme": "# Source Map\n\nThis is a library to generate and consume the source map format\n[described here][format].\n\nThis library is written in the Asynchronous Module Definition format, and works\nin the following environments:\n\n* Modern Browsers supporting ECMAScript 5 (either after the build, or with an\n AMD loader such as RequireJS)\n\n* Inside Firefox (as a JSM file, after the build)\n\n* With NodeJS versions 0.8.X and higher\n\n## Node\n\n $ npm install source-map\n\n## Building from Source (for everywhere else)\n\nInstall Node and then run\n\n $ git clone https://fitzgen@github.com/mozilla/source-map.git\n $ cd source-map\n $ npm link .\n\nNext, run\n\n $ node Makefile.dryice.js\n\nThis should spew a bunch of stuff to stdout, and create the following files:\n\n* `dist/source-map.js` - The unminified browser version.\n\n* `dist/source-map.min.js` - The minified browser version.\n\n* `dist/SourceMap.jsm` - The JavaScript Module for inclusion in Firefox source.\n\n## Examples\n\n### Consuming a source map\n\n var rawSourceMap = {\n version: 3,\n file: 'min.js',\n names: ['bar', 'baz', 'n'],\n sources: ['one.js', 'two.js'],\n sourceRoot: 'http://example.com/www/js/',\n mappings: 'CAAC,IAAI,IAAM,SAAUA,GAClB,OAAOC,IAAID;CCDb,IAAI,IAAM,SAAUE,GAClB,OAAOA'\n };\n\n var smc = new SourceMapConsumer(rawSourceMap);\n\n console.log(smc.sources);\n // [ 'http://example.com/www/js/one.js',\n // 'http://example.com/www/js/two.js' ]\n\n console.log(smc.originalPositionFor({\n line: 2,\n column: 28\n }));\n // { source: 'http://example.com/www/js/two.js',\n // line: 2,\n // column: 10,\n // name: 'n' }\n\n console.log(smc.generatedPositionFor({\n source: 'http://example.com/www/js/two.js',\n line: 2,\n column: 10\n }));\n // { line: 2, column: 28 }\n\n smc.eachMapping(function (m) {\n // ...\n });\n\n### Generating a source map\n\nIn depth guide:\n[**Compiling to JavaScript, and Debugging with Source Maps**](https://hacks.mozilla.org/2013/05/compiling-to-javascript-and-debugging-with-source-maps/)\n\n#### With SourceNode (high level API)\n\n function compile(ast) {\n switch (ast.type) {\n case 'BinaryExpression':\n return new SourceNode(\n ast.location.line,\n ast.location.column,\n ast.location.source,\n [compile(ast.left), \" + \", compile(ast.right)]\n );\n case 'Literal':\n return new SourceNode(\n ast.location.line,\n ast.location.column,\n ast.location.source,\n String(ast.value)\n );\n // ...\n default:\n throw new Error(\"Bad AST\");\n }\n }\n\n var ast = parse(\"40 + 2\", \"add.js\");\n console.log(compile(ast).toStringWithSourceMap({\n file: 'add.js'\n }));\n // { code: '40 + 2',\n // map: [object SourceMapGenerator] }\n\n#### With SourceMapGenerator (low level API)\n\n var map = new SourceMapGenerator({\n file: \"source-mapped.js\"\n });\n\n map.addMapping({\n generated: {\n line: 10,\n column: 35\n },\n source: \"foo.js\",\n original: {\n line: 33,\n column: 2\n },\n name: \"christopher\"\n });\n\n console.log(map.toString());\n // '{\"version\":3,\"file\":\"source-mapped.js\",\"sources\":[\"foo.js\"],\"names\":[\"christopher\"],\"mappings\":\";;;;;;;;;mCAgCEA\"}'\n\n## API\n\nGet a reference to the module:\n\n // NodeJS\n var sourceMap = require('source-map');\n\n // Browser builds\n var sourceMap = window.sourceMap;\n\n // Inside Firefox\n let sourceMap = {};\n Components.utils.import('resource:///modules/devtools/SourceMap.jsm', sourceMap);\n\n### SourceMapConsumer\n\nA SourceMapConsumer instance represents a parsed source map which we can query\nfor information about the original file positions by giving it a file position\nin the generated source.\n\n#### new SourceMapConsumer(rawSourceMap)\n\nThe only parameter is the raw source map (either as a string which can be\n`JSON.parse`'d, or an object). According to the spec, source maps have the\nfollowing attributes:\n\n* `version`: Which version of the source map spec this map is following.\n\n* `sources`: An array of URLs to the original source files.\n\n* `names`: An array of identifiers which can be referrenced by individual\n mappings.\n\n* `sourceRoot`: Optional. The URL root from which all sources are relative.\n\n* `sourcesContent`: Optional. An array of contents of the original source files.\n\n* `mappings`: A string of base64 VLQs which contain the actual mappings.\n\n* `file`: The generated filename this source map is associated with.\n\n#### SourceMapConsumer.prototype.originalPositionFor(generatedPosition)\n\nReturns the original source, line, and column information for the generated\nsource's line and column positions provided. The only argument is an object with\nthe following properties:\n\n* `line`: The line number in the generated source.\n\n* `column`: The column number in the generated source.\n\nand an object is returned with the following properties:\n\n* `source`: The original source file, or null if this information is not\n available.\n\n* `line`: The line number in the original source, or null if this information is\n not available.\n\n* `column`: The column number in the original source, or null or null if this\n information is not available.\n\n* `name`: The original identifier, or null if this information is not available.\n\n#### SourceMapConsumer.prototype.generatedPositionFor(originalPosition)\n\nReturns the generated line and column information for the original source,\nline, and column positions provided. The only argument is an object with\nthe following properties:\n\n* `source`: The filename of the original source.\n\n* `line`: The line number in the original source.\n\n* `column`: The column number in the original source.\n\nand an object is returned with the following properties:\n\n* `line`: The line number in the generated source, or null.\n\n* `column`: The column number in the generated source, or null.\n\n#### SourceMapConsumer.prototype.sourceContentFor(source)\n\nReturns the original source content for the source provided. The only\nargument is the URL of the original source file.\n\n#### SourceMapConsumer.prototype.eachMapping(callback, context, order)\n\nIterate over each mapping between an original source/line/column and a\ngenerated line/column in this source map.\n\n* `callback`: The function that is called with each mapping. Mappings have the\n form `{ source, generatedLine, generatedColumn, originalLine, originalColumn,\n name }`\n\n* `context`: Optional. If specified, this object will be the value of `this`\n every time that `callback` is called.\n\n* `order`: Either `SourceMapConsumer.GENERATED_ORDER` or\n `SourceMapConsumer.ORIGINAL_ORDER`. Specifies whether you want to iterate over\n the mappings sorted by the generated file's line/column order or the\n original's source/line/column order, respectively. Defaults to\n `SourceMapConsumer.GENERATED_ORDER`.\n\n### SourceMapGenerator\n\nAn instance of the SourceMapGenerator represents a source map which is being\nbuilt incrementally.\n\n#### new SourceMapGenerator(startOfSourceMap)\n\nTo create a new one, you must pass an object with the following properties:\n\n* `file`: The filename of the generated source that this source map is\n associated with.\n\n* `sourceRoot`: An optional root for all relative URLs in this source map.\n\n#### SourceMapGenerator.fromSourceMap(sourceMapConsumer)\n\nCreates a new SourceMapGenerator based on a SourceMapConsumer\n\n* `sourceMapConsumer` The SourceMap.\n\n#### SourceMapGenerator.prototype.addMapping(mapping)\n\nAdd a single mapping from original source line and column to the generated\nsource's line and column for this source map being created. The mapping object\nshould have the following properties:\n\n* `generated`: An object with the generated line and column positions.\n\n* `original`: An object with the original line and column positions.\n\n* `source`: The original source file (relative to the sourceRoot).\n\n* `name`: An optional original token name for this mapping.\n\n#### SourceMapGenerator.prototype.setSourceContent(sourceFile, sourceContent)\n\nSet the source content for an original source file.\n\n* `sourceFile` the URL of the original source file.\n\n* `sourceContent` the content of the source file.\n\n#### SourceMapGenerator.prototype.applySourceMap(sourceMapConsumer[, sourceFile])\n\nApplies a SourceMap for a source file to the SourceMap.\nEach mapping to the supplied source file is rewritten using the\nsupplied SourceMap. Note: The resolution for the resulting mappings\nis the minimium of this map and the supplied map.\n\n* `sourceMapConsumer`: The SourceMap to be applied.\n\n* `sourceFile`: Optional. The filename of the source file.\n If omitted, sourceMapConsumer.file will be used.\n\n#### SourceMapGenerator.prototype.toString()\n\nRenders the source map being generated to a string.\n\n### SourceNode\n\nSourceNodes provide a way to abstract over interpolating and/or concatenating\nsnippets of generated JavaScript source code, while maintaining the line and\ncolumn information associated between those snippets and the original source\ncode. This is useful as the final intermediate representation a compiler might\nuse before outputting the generated JS and source map.\n\n#### new SourceNode(line, column, source[, chunk[, name]])\n\n* `line`: The original line number associated with this source node, or null if\n it isn't associated with an original line.\n\n* `column`: The original column number associated with this source node, or null\n if it isn't associated with an original column.\n\n* `source`: The original source's filename.\n\n* `chunk`: Optional. Is immediately passed to `SourceNode.prototype.add`, see\n below.\n\n* `name`: Optional. The original identifier.\n\n#### SourceNode.fromStringWithSourceMap(code, sourceMapConsumer)\n\nCreates a SourceNode from generated code and a SourceMapConsumer.\n\n* `code`: The generated code\n\n* `sourceMapConsumer` The SourceMap for the generated code\n\n#### SourceNode.prototype.add(chunk)\n\nAdd a chunk of generated JS to this source node.\n\n* `chunk`: A string snippet of generated JS code, another instance of\n `SourceNode`, or an array where each member is one of those things.\n\n#### SourceNode.prototype.prepend(chunk)\n\nPrepend a chunk of generated JS to this source node.\n\n* `chunk`: A string snippet of generated JS code, another instance of\n `SourceNode`, or an array where each member is one of those things.\n\n#### SourceNode.prototype.setSourceContent(sourceFile, sourceContent)\n\nSet the source content for a source file. This will be added to the\n`SourceMap` in the `sourcesContent` field.\n\n* `sourceFile`: The filename of the source file\n\n* `sourceContent`: The content of the source file\n\n#### SourceNode.prototype.walk(fn)\n\nWalk over the tree of JS snippets in this node and its children. The walking\nfunction is called once for each snippet of JS and is passed that snippet and\nthe its original associated source's line/column location.\n\n* `fn`: The traversal function.\n\n#### SourceNode.prototype.walkSourceContents(fn)\n\nWalk over the tree of SourceNodes. The walking function is called for each\nsource file content and is passed the filename and source content.\n\n* `fn`: The traversal function.\n\n#### SourceNode.prototype.join(sep)\n\nLike `Array.prototype.join` except for SourceNodes. Inserts the separator\nbetween each of this source node's children.\n\n* `sep`: The separator.\n\n#### SourceNode.prototype.replaceRight(pattern, replacement)\n\nCall `String.prototype.replace` on the very right-most source snippet. Useful\nfor trimming whitespace from the end of a source node, etc.\n\n* `pattern`: The pattern to replace.\n\n* `replacement`: The thing to replace the pattern with.\n\n#### SourceNode.prototype.toString()\n\nReturn the string representation of this source node. Walks over the tree and\nconcatenates all the various snippets together to one string.\n\n### SourceNode.prototype.toStringWithSourceMap(startOfSourceMap)\n\nReturns the string representation of this tree of source nodes, plus a\nSourceMapGenerator which contains all the mappings between the generated and\noriginal sources.\n\nThe arguments are the same as those to `new SourceMapGenerator`.\n\n## Tests\n\n[](https://travis-ci.org/mozilla/source-map)\n\nInstall NodeJS version 0.8.0 or greater, then run `node test/run-tests.js`.\n\nTo add new tests, create a new file named `test/test-.js`\nand export your test functions with names that start with \"test\", for example\n\n exports[\"test doing the foo bar\"] = function (assert, util) {\n ...\n };\n\nThe new test will be located automatically when you run the suite.\n\nThe `util` argument is the test utility module located at `test/source-map/util`.\n\nThe `assert` argument is a cut down version of node's assert module. You have\naccess to the following assertion functions:\n\n* `doesNotThrow`\n\n* `equal`\n\n* `ok`\n\n* `strictEqual`\n\n* `throws`\n\n(The reason for the restricted set of test functions is because we need the\ntests to run inside Firefox's test suite as well and so the assert module is\nshimmed in that environment. See `build/assert-shim.js`.)\n\n[format]: https://docs.google.com/document/d/1U1RGAehQwRypUTovF1KRlpiOFze0b-_2gc6fAH0KY0k/edit\n[feature]: https://wiki.mozilla.org/DevTools/Features/SourceMap\n[Dryice]: https://github.com/mozilla/dryice\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/mozilla/source-map/issues"
},
"_id": "source-map@0.1.31",
"_from": "source-map@0.1.31",
"realName": "source-map",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/source-map",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform/node_modules/source-map",
"parent": "[Circular]",
"depth": 4,
"peerDependencies": {}
}
},
"licenses": [
{
"type": "Apache-2.0",
"url": "http://www.apache.org/licenses/LICENSE-2.0"
}
],
"engines": {
"node": ">=0.8.8"
},
"devDependencies": {
"jest-cli": "^0.2",
"jshint": "^2.5.10"
},
"jest": {
"scriptPreprocessor": "/jestPreprocessor.js",
"setupEnvScriptFile": "/jestEnvironment.js",
"testPathIgnorePatterns": [
"/node_modules/",
"/__tests__/[^/]*/.+"
]
},
"scripts": {
"prepublish": "jest && jshint --config=.jshintrc --exclude=node_modules .",
"test": "jest && jshint --config=.jshintrc --exclude=node_modules ."
},
"readme": "# JSTransform [](https://travis-ci.org/facebook/jstransform)\n\nA simple utility for pluggable JS syntax transforms using the esprima parser.\n\n* Makes it simple to write and plug-in syntax transformations\n* Makes it simple to coalesce multiple syntax transformations in a single pass of the AST\n* Gives complete control over the formatting of the output on a per-transformation basis\n* Supports source map generation\n* Comes pre-bundled with a small set of (optional) ES6 -> ES5 transforms\n\nNOTE: If you're looking for a library for writing new greenfield JS transformations, consider looking at the [Recast](https://github.com/benjamn/recast) library instead of jstransform. We are still actively supporting jstransform (and intend to for the foreseeable future), but longer term we would like to direct efforts toward Recast. Recast does a far better job of supporting a multi-pass JS transformation pipeline, and this is important when attempting to apply many transformations to a source file.\n\n## Examples\nUsing a pre-bundled or existing transform:\n```js\n/**\n * Reads a source file that may (or may not) contain ES6 classes, transforms it\n * to ES5 compatible code using the pre-bundled ES6 class visitors, and prints \n * out the result.\n */\nvar es6ClassVisitors = require('jstransform/visitors/es6-class-visitors').visitorList;\nvar fs = require('fs');\nvar jstransform = require('jstransform');\n\nvar originalFileContents = fs.readFileSync('path/to/original/file.js', 'utf-8');\n\nvar transformedFileData = jstransform.transform(\n es6ClassVisitors,\n originalFileContents\n);\n\nconsole.log(transformedFileData.code);\n```\n\nUsing multiple pre-bundled or existing transforms at once:\n```js\n/**\n * Reads a source file that may (or may not) contain ES6 classes *or* arrow\n * functions, transforms them to ES5 compatible code using the pre-bundled ES6 \n * visitors, and prints out the result.\n */\nvar es6ArrowFuncVisitors = require('jstransform/visitors/es6-arrow-function-visitors').visitorList;\nvar es6ClassVisitors = require('jstransform/visitors/es6-class-visitors').visitorList;\nvar jstransform = require('jstransform');\n\n// Normally you'd read this from the filesystem, but I'll just use a string here\n// to simplify the example.\nvar originalFileContents = \"var a = (param1) => param1; class FooClass {}\";\n\nvar transformedFileData = jstransform.transform(\n es6ClassVisitors.concat(es6ArrowFuncVisitors),\n originalFileContents\n);\n\n// var a = function(param1) {return param1;}; function FooClass(){\"use strict\";}\nconsole.log(transformedFileData.code);\n```\n\nWriting a simple custom transform:\n```js\n/**\n * Creates a custom transformation visitor that prefixes all calls to the\n * `eval()` function with a call to `alert()` saying how much of a clown you are\n * for using eval.\n */\nvar jstransform = require('jstransform');\nvar utils = require('jstransform/src/utils');\n\nvar Syntax = jstransform.Syntax;\n\nfunction visitEvalCallExpressions(traverse, node, path, state) {\n // Appends an alert() call to the output buffer *before* the visited node\n // (in this case the eval call) is appended to the output buffer\n utils.append('alert(\"...eval?...really?...\");', state);\n\n // Now we copy the eval expression to the output buffer from the original\n // source\n utils.catchup(node.range[1], state);\n}\nvisitEvalCallExpressions.test = function(node, path, state) {\n return node.type === Syntax.CallExpression\n && node.callee.type === Syntax.Identifier\n && node.callee.name === 'eval';\n};\n\n// Normally you'd read this from the filesystem, but I'll just use a string here\n// to simplify the example.\nvar originalFileContents = \"eval('foo');\";\n\nvar transformedFileData = jstransform.transform(\n [visitEvalCallExpressions], // Multiple visitors may be applied at once, so an\n // array is always expected for the first argument\n originalFileContents\n);\n\n// alert(\"...eval?...really?...\");eval('foo');\nconsole.log(transformedFileData.code);\n```\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/facebook/jstransform/issues"
},
"_id": "jstransform@10.1.0",
"_from": "jstransform@^10.0.1",
"realName": "jstransform",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify/node_modules/jstransform",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
}
},
"keywords": [
"environment",
"variables",
"browserify",
"browserify-transform",
"transform",
"source",
"configuration"
],
"readme": "# envify [](http://travis-ci.org/hughsk/envify) [](http://github.com/hughsk/stability-badges) #\n\nSelectively replace Node-style environment variables with plain strings.\nAvailable as a standalone CLI tool and a\n[Browserify](http://browserify.org) v2 transform.\n\nWorks best in combination with [uglifyify](http://github.com/hughsk/uglifyify).\n\n## Installation ##\n\nIf you're using the module with Browserify:\n\n``` bash\nnpm install envify browserify\n```\n\nOr, for the CLI:\n\n``` bash\nsudo npm install -g envify\n```\n\n## Usage ##\n\nenvify will replace your environment variable checks with ordinary strings -\nonly the variables you use will be included, so you don't have to worry about,\nsay, `AWS_SECRET_KEY` leaking through either. Take this example script:\n\n``` javascript\nif (process.env.NODE_ENV === \"development\") {\n console.log('development only')\n}\n```\n\nAfter running it through envify with `NODE_ENV` set to `production`, you'll\nget this:\n\n``` javascript\nif (\"production\" === \"development\") {\n console.log('development only')\n}\n```\n\nBy running this through a good minifier (e.g.\n[UglifyJS2](https://github.com/mishoo/UglifyJS)), the above code would be\nstripped out completely.\n\nHowever, if you bundled the same script with `NODE_ENV` set to `development`:\n\n``` javascript\nif (\"development\" === \"development\") {\n console.log('development only')\n}\n```\n\nThe `if` statement will evaluate to `true`, so the code won't be removed.\n\n## CLI Usage ##\n\nWith browserify:\n\n``` bash\nbrowserify index.js -t envify > bundle.js\n```\n\nOr standalone:\n\n``` bash\nenvify index.js > bundle.js\n```\n\nYou can also specify additional custom environment variables using\nbrowserify's [subarg](http://github.com/substack/subarg) syntax, which is\navailable in versions 3.25.0 and above:\n\n``` bash\nbrowserify index.js -t [ envify --NODE_ENV development ] > bundle.js\nbrowserify index.js -t [ envify --NODE_ENV production ] > bundle.js\n```\n\n## Module Usage ##\n\n**require('envify')**\n\nReturns a transform stream that updates based on the Node process'\n`process.env` object.\n\n**require('envify/custom')([environment])**\n\nIf you want to stay away from your environment variables, you can supply\nyour own object to use in its place:\n\n``` javascript\nvar browserify = require('browserify')\n , envify = require('envify/custom')\n , fs = require('fs')\n\nvar b = browserify('main.js')\n , output = fs.createWriteStream('bundle.js')\n\nb.transform(envify({\n NODE_ENV: 'development'\n}))\nb.bundle().pipe(output)\n```\n\n## Purging `process.env` ##\n\nBy default, environment variables that are not defined will be left untouched.\nThis is because in some cases, you might want to run an envify transform over\nyour source more than once, and removing these values would make that\nimpossible.\n\nHowever, if any references to `process.env` are remaining after transforming\nyour source with envify, browserify will automatically insert its shim for\nNode's process object, which will increase the size of your bundle. This weighs\nin at around 2KB, so if you're trying to be conservative with your bundle size\nyou can \"purge\" these remaining variables such that any missing ones are simply\nreplaced with undefined.\n\nTo do so through the command-line, simply use the subarg syntax and include\n`purge` after `envify`, e.g.:\n\n``` bash\nbrowserify index.js -t [ envify purge --NODE_ENV development ]\n```\n\nOr if you're using the module API, you can pass `_: \"purge\"` into your\narguments like so:\n\n``` javascript\nb.transform(envify({\n _: 'purge'\n , NODE_ENV: 'development'\n}))\n```\n\n## Contributors ##\n\n* [hughsk](http://github.com/hughsk)\n* [benjamn](http://github.com/benjamn)\n* [zag2art](http://github.com/zag2art)\n* [bjoerge](http://github.com/bjoerge)\n* [andreypopp](http://github.com/andreypopp)\n* [jupl](http://github.com/jupl)\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/hughsk/envify/issues"
},
"_id": "envify@3.4.0",
"_from": "envify@^3.0.0",
"realName": "envify",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/envify",
"parent": "[Circular]",
"depth": 2,
"peerDependencies": {}
},
"fbjs": {
"name": "fbjs",
"version": "0.6.1",
"description": "A collection of utility libraries used by other Facebook JS projects",
"main": "index.js",
"repository": {
"type": "git",
"url": "git://github.com/facebook/fbjs"
},
"scripts": {
"build": "gulp build",
"lint": "eslint .",
"prepublish": "npm run build",
"pretest": "node node_modules/fbjs-scripts/node/check-dev-engines.js package.json",
"test": "NODE_ENV=test jest",
"typecheck": "flow check src"
},
"author": "",
"license": "BSD-3-Clause",
"devDependencies": {
"babel": "^5.4.7",
"babel-eslint": "4.1.5",
"del": "^1.2.1",
"eslint": "1.10.3",
"fbjs-scripts": "file:scripts",
"flow-bin": "^0.18.1",
"gulp": "^3.9.0",
"gulp-babel": "^5.1.0",
"gulp-flatten": "^0.2.0",
"gulp-rename": "^1.2.2",
"jest-cli": "^0.7.1",
"merge-stream": "^1.0.0",
"object-assign": "^4.0.1",
"run-sequence": "^1.1.0"
},
"files": [
"LICENSE",
"PATENTS",
"README.md",
"flow/",
"index.js",
"lib/",
"module-map.json"
],
"jest": {
"modulePathIgnorePatterns": [
"/lib/",
"/node_modules/"
],
"persistModuleRegistryBetweenSpecs": true,
"preprocessorIgnorePatterns": [
"/node_modules/"
],
"rootDir": "",
"scriptPreprocessor": "node_modules/fbjs-scripts/jest/preprocessor.js",
"setupEnvScriptFile": "node_modules/fbjs-scripts/jest/environment.js",
"testPathDirs": [
"/src"
],
"unmockedModulePathPatterns": [
"/node_modules/",
"/src/(?!(__forks__/fetch.js$|fetch/))"
]
},
"dependencies": {
"core-js": {
"name": "core-js",
"description": "Standard library",
"version": "1.2.6",
"repository": {
"type": "git",
"url": "https://github.com/zloirock/core-js.git"
},
"main": "index.js",
"devDependencies": {
"webpack": "1.12.x",
"LiveScript": "1.3.x",
"grunt": "0.4.x",
"grunt-cli": "0.1.x",
"grunt-livescript": "0.5.x",
"grunt-contrib-uglify": "0.10.x",
"grunt-contrib-watch": "0.6.x",
"grunt-contrib-clean": "0.6.x",
"grunt-contrib-copy": "0.8.x",
"grunt-karma": "0.12.x",
"karma": "0.13.x",
"karma-qunit": "0.1.x",
"karma-chrome-launcher": "0.2.x",
"karma-ie-launcher": "0.2.x",
"karma-firefox-launcher": "0.1.x",
"karma-phantomjs-launcher": "0.2.x",
"promises-aplus-tests": "2.1.x",
"eslint": "1.9.x"
},
"scripts": {
"grunt": "grunt",
"lint": "eslint es5 es6 es7 js web core fn modules",
"promises-tests": "promises-aplus-tests tests/promises-aplus/adapter",
"test": "npm run lint && npm run grunt livescript client karma:continuous library karma:continuous-library && npm run promises-tests && lsc tests/commonjs"
},
"license": "MIT",
"keywords": [
"ES5",
"ECMAScript 5",
"ES6",
"ECMAScript 6",
"ES7",
"ECMAScript 7",
"Harmony",
"Strawman",
"Map",
"Set",
"WeakMap",
"WeakSet",
"Promise",
"Symbol",
"Array generics",
"setImmediate",
"Dict",
"partial application"
],
"readme": "# core-js\n\n[](https://gitter.im/zloirock/core-js?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) [](https://www.npmjs.com/package/core-js) [](http://npm-stat.com/charts.html?package=core-js&author=&from=2014-11-18&to=2114-11-18) [](https://travis-ci.org/zloirock/core-js) [](https://david-dm.org/zloirock/core-js#info=devDependencies)\n\nModular compact standard library for JavaScript. Includes polyfills for [ECMAScript 5](#ecmascript-5), [ECMAScript 6](#ecmascript-6): [symbols](#ecmascript-6-symbol), [collections](#ecmascript-6-collections), [iterators](#ecmascript-6-iterators), [promises](#ecmascript-6-promise), [ECMAScript 7 proposals](#ecmascript-7); [setImmediate](#setimmediate), [array generics](#mozilla-javascript-array-generics). Some additional features such as [dictionaries](#dict) or [extended partial application](#partial-application). You can require only standardized features polyfills, use features without global namespace pollution or create a custom build.\n\n[Example](http://goo.gl/mfHYm2):\n```javascript\nArray.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]\n'*'.repeat(10); // => '**********'\nPromise.resolve(32).then(log); // => 32\nsetImmediate(log, 42); // => 42\n```\n\n[Without global namespace pollution](http://goo.gl/WBhs43):\n```javascript\nvar core = require('core-js/library'); // With a modular system, otherwise use global `core`\ncore.Array.from(new core.Set([1, 2, 3, 2, 1])); // => [1, 2, 3]\ncore.String.repeat('*', 10); // => '**********'\ncore.Promise.resolve(32).then(core.log); // => 32\ncore.setImmediate(core.log, 42); // => 42\n```\n\n- [Usage](#usage)\n - [Basic](#basic)\n - [CommonJS](#commonjs)\n - [Custom build](#custom-build)\n- [Features](#features)\n - [ECMAScript 5](#ecmascript-5)\n - [ECMAScript 6](#ecmascript-6)\n - [ECMAScript 6: Object](#ecmascript-6-object)\n - [ECMAScript 6: Function](#ecmascript-6-function)\n - [ECMAScript 6: Array](#ecmascript-6-array)\n - [ECMAScript 6: String](#ecmascript-6-string)\n - [ECMAScript 6: RegExp](#ecmascript-6-regexp)\n - [ECMAScript 6: Number](#ecmascript-6-number)\n - [ECMAScript 6: Math](#ecmascript-6-math)\n - [ECMAScript 6: Symbol](#ecmascript-6-symbol)\n - [ECMAScript 6: Collections](#ecmascript-6-collections)\n - [ECMAScript 6: Iterators](#ecmascript-6-iterators)\n - [ECMAScript 6: Promise](#ecmascript-6-promise)\n - [ECMAScript 6: Reflect](#ecmascript-6-reflect)\n - [ECMAScript 7](#ecmascript-7)\n - [Mozilla JavaScript: Array generics](#mozilla-javascript-array-generics)\n - [Web standards](#web-standards)\n - [setTimeout / setInterval](#settimeout--setinterval)\n - [setImmediate](#setimmediate)\n - [Non-standard](#non-standard)\n - [Object](#object)\n - [Dict](#dict)\n - [Partial application](#partial-application)\n - [Number Iterator](#number-iterator)\n - [Escaping HTML](#escaping-html)\n - [delay](#delay)\n - [console](#console)\n- [Missing polyfills](#missing-polyfills)\n- [Changelog](./CHANGELOG.md)\n\n## Usage\n### Basic\n```\nnpm i core-js\nbower install core.js\n```\n\n```javascript\n// Default\nrequire('core-js');\n// Without global namespace pollution\nvar core = require('core-js/library');\n// Shim only\nrequire('core-js/shim');\n```\nIf you need complete build for browser, use builds from `core-js/client` path: [default](https://raw.githack.com/zloirock/core-js/master/client/core.min.js), [without global namespace pollution](https://raw.githack.com/zloirock/core-js/master/client/library.min.js), [shim only](https://raw.githack.com/zloirock/core-js/master/client/shim.min.js).\n\nWarning: if you uses `core-js` with the extension of native objects, require all needed `core-js` modules at the beginning of entry point of your application, otherwise maybe conflicts.\n\n### CommonJS\nYou can require only needed modules.\n\n```js\nrequire('core-js/es5'); // if you need support IE8-\nrequire('core-js/fn/set');\nrequire('core-js/fn/array/from');\nrequire('core-js/fn/array/find-index');\nArray.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]\n[1, 2, NaN, 3, 4].findIndex(isNaN); // => 2\n\n// or, w/o global namespace pollution:\n\nvar core = require('core-js/library/es5'); // if you need support IE8-\nvar Set = require('core-js/library/fn/set');\nvar from = require('core-js/library/fn/array/from');\nvar findIndex = require('core-js/library/fn/array/find-index');\nfrom(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]\nfindIndex([1, 2, NaN, 3, 4], isNaN); // => 2\n```\nAvailable entry points for methods / constructors, as above examples, excluding features from [`es5`](#ecmascript-5) module (this module requires completely in ES3 environment before all other modules).\n\nAvailable namespaces: for example, `core-js/es6/array` (`core-js/library/es6/array`) contains all [ES6 `Array` features](#ecmascript-6-array), `core-js/es6` (`core-js/library/es6`) contains all ES6 features.\n\n### Custom build\n```\nnpm i core-js && cd node_modules/core-js && npm i\nnpm run grunt build:core.dict,es6 -- --blacklist=es6.promise,es6.math --library=on --path=custom uglify\n```\nWhere `core.dict` and `es6` are modules (namespaces) names, which will be added to the build, `es6.promise` and `es6.math` are modules (namespaces) names, which will be excluded from the build, `--library=on` is flag for build without global namespace pollution and `custom` is target file name.\n\nAvailable namespaces: for example, `es6.array` contains [ES6 `Array` features](#ecmascript-6-array), `es6` contains all modules whose names start with `es6`.\n\nAvailable custom build from js code (required `webpack`):\n```js\nrequire('core-js/build')({\n modules: ['es6', 'core.dict'], // modules / namespaces\n blacklist: ['es6.reflect'], // blacklist of modules / namespaces\n library: false, // flag for build without global namespace pollution\n}, function(err, code){ // callback\n // ...\n});\n```\n## Features:\n### ECMAScript 5\nModule [`es5`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es5.js), nothing new - without examples.\n```javascript\nObject\n .create(proto | null, descriptors?) -> object\n .getPrototypeOf(object) -> proto | null\n .defineProperty(target, key, desc) -> target, cap for ie8-\n .defineProperties(target, descriptors) -> target, cap for ie8-\n .getOwnPropertyDescriptor(object, key) -> desc\n .getOwnPropertyNames(object) -> array\n .keys(object) -> array\nArray\n .isArray(var) -> bool\n #slice(start?, end?) -> array, fix for ie7-\n #join(string = ',') -> string, fix for ie7-\n #indexOf(var, from?) -> int\n #lastIndexOf(var, from?) -> int\n #every(fn(val, index, @), that) -> bool\n #some(fn(val, index, @), that) -> bool\n #forEach(fn(val, index, @), that) -> void\n #map(fn(val, index, @), that) -> array\n #filter(fn(val, index, @), that) -> array\n #reduce(fn(memo, val, index, @), memo?) -> var\n #reduceRight(fn(memo, val, index, @), memo?) -> var\nFunction\n #bind(object, ...args) -> boundFn(...args)\nDate\n .now() -> int\n #toISOString() -> string\n```\nSome features moved to [another modules / namespaces](#ecmascript-6), but available as part of `es5` namespace too:\n```js\nObject\n .seal(object) -> object, cap for ie8-\n .freeze(object) -> object, cap for ie8-\n .preventExtensions(object) -> object, cap for ie8-\n .isSealed(object) -> bool, cap for ie8-\n .isFrozen(object) -> bool, cap for ie8-\n .isExtensible(object) -> bool, cap for ie8-\nString\n #trim() -> str\n```\n\n### ECMAScript 6\n#### ECMAScript 6: Object\nModules [`es6.object.assign`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.assign.js), [`es6.object.is`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.is.js), [`es6.object.set-prototype-of`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.set-prototype-of.js) and [`es6.object.to-string`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.to-string.js).\n```javascript\nObject\n .assign(target, ...src) -> target\n .is(a, b) -> bool\n .setPrototypeOf(target, proto | null) -> target (required __proto__ - IE11+)\n #toString() -> string, ES6 fix: @@toStringTag support\n```\n[Example](http://goo.gl/VzmY3j):\n```javascript\nvar foo = {q: 1, w: 2}\n , bar = {e: 3, r: 4}\n , baz = {t: 5, y: 6};\nObject.assign(foo, bar, baz); // => foo = {q: 1, w: 2, e: 3, r: 4, t: 5, y: 6}\n\nObject.is(NaN, NaN); // => true\nObject.is(0, -0); // => false\nObject.is(42, 42); // => true\nObject.is(42, '42'); // => false\n\nfunction Parent(){}\nfunction Child(){}\nObject.setPrototypeOf(Child.prototype, Parent.prototype);\nnew Child instanceof Child; // => true\nnew Child instanceof Parent; // => true\n\nvar O = {};\nO[Symbol.toStringTag] = 'Foo';\n'' + O; // => '[object Foo]'\n```\nIn ES6 most `Object` static methods should work with primitives. Modules [`es6.object.freeze`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.freeze.js), [`es6.object.seal`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.seal.js), [`es6.object.prevent-extensions`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.prevent-extensions.js), [`es6.object.is-frozen`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.is-frozen.js), [`es6.object.is-sealed`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.is-sealed.js), [`es6.object.is-extensible`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.is-extensible.js), [`es6.object.get-own-property-descriptor`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.get-own-property-descriptor.js), [`es6.object.get-prototype-of`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.get-prototype-of.js), [`es6.object.keys`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.keys.js), [`es6.object.get-own-property-names`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.object.get-own-property-names.js).\n```javascript\nObject\n .freeze(var) -> var\n .seal(var) -> var\n .preventExtensions(var) -> var\n .isFrozen(var) -> bool\n .isSealed(var) -> bool\n .isExtensible(var) -> bool\n .getOwnPropertyDescriptor(var, key) -> desc | undefined\n .getPrototypeOf(var) -> object | null\n .keys(var) -> array\n .getOwnPropertyNames(var) -> array\n```\n[Example](http://goo.gl/35lPSi):\n```javascript\nObject.keys('qwe'); // => ['0', '1', '2']\nObject.getPrototypeOf('qwe') === String.prototype; // => true\n```\n#### ECMAScript 6: Function\nModules [`es6.function.name`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.function.name.js) and [`es6.function.has-instance`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.function.has-instance.js).\n```javascript\nFunction\n #name -> string (IE9+)\n #@@hasInstance(var) -> bool\n```\n[Example](http://goo.gl/zqu3Wp):\n```javascript\n(function foo(){}).name // => 'foo'\n```\n#### ECMAScript 6: Array\nModules [`es6.array.from`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.from.js), [`es6.array.of`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.of.js), [`es6.array.copy-within`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.copy-within.js), [`es6.array.fill`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.fill.js), [`es6.array.find`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.find.js) and [`es6.array.find-index`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.find-index.js).\n```javascript\nArray\n .from(iterable | array-like, mapFn(val, index)?, that) -> array\n .of(...args) -> array\n #copyWithin(target = 0, start = 0, end = @length) -> @\n #fill(val, start = 0, end = @length) -> @\n #find(fn(val, index, @), that) -> val\n #findIndex(fn(val, index, @), that) -> index\n #@@unscopables -> object (cap)\n```\n[Example](http://goo.gl/nxmJTe):\n```javascript\nArray.from(new Set([1, 2, 3, 2, 1])); // => [1, 2, 3]\nArray.from({0: 1, 1: 2, 2: 3, length: 3}); // => [1, 2, 3]\nArray.from('123', Number); // => [1, 2, 3]\nArray.from('123', function(it){\n return it * it;\n}); // => [1, 4, 9]\n\nArray.of(1); // => [1]\nArray.of(1, 2, 3); // => [1, 2, 3]\n\nfunction isOdd(val){\n return val % 2;\n}\n[4, 8, 15, 16, 23, 42].find(isOdd); // => 15\n[4, 8, 15, 16, 23, 42].findIndex(isOdd); // => 2\n[4, 8, 15, 16, 23, 42].find(isNaN); // => undefined\n[4, 8, 15, 16, 23, 42].findIndex(isNaN); // => -1\n\nArray(5).fill(42); // => [42, 42, 42, 42, 42]\n\n[1, 2, 3, 4, 5].copyWithin(0, 3); // => [4, 5, 3, 4, 5]\n```\n#### ECMAScript 6: String\nModules [`es6.string.from-code-point`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.from-code-point.js), [`es6.string.raw`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.raw.js), [`es6.string.code-point-at`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.code-point-at.js), [`es6.string.ends-with`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.ends-with.js), [`es6.string.includes`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.includes.js), [`es6.string.repeat`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.repeat.js), [`es6.string.starts-with`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.starts-with.js) and [`es6.string.trim`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.trim.js).\n```javascript\nString\n .fromCodePoint(...codePoints) -> str\n .raw({raw}, ...substitutions) -> str\n #includes(str, from?) -> bool\n #startsWith(str, from?) -> bool\n #endsWith(str, from?) -> bool\n #repeat(num) -> str\n #codePointAt(pos) -> uint\n #trim() -> str, ES6 fix\n```\n[Examples](http://goo.gl/RMyFBo):\n```javascript\n'foobarbaz'.includes('bar'); // => true\n'foobarbaz'.includes('bar', 4); // => false\n'foobarbaz'.startsWith('foo'); // => true\n'foobarbaz'.startsWith('bar', 3); // => true\n'foobarbaz'.endsWith('baz'); // => true\n'foobarbaz'.endsWith('bar', 6); // => true\n\n'string'.repeat(3); // => 'stringstringstring'\n\n'𠮷'.codePointAt(0); // => 134071\nString.fromCodePoint(97, 134071, 98); // => 'a𠮷b'\n\nvar name = 'Bob';\nString.raw`Hi\\n${name}!`; // => 'Hi\\\\nBob!' (ES6 template string syntax)\nString.raw({raw: 'test'}, 0, 1, 2); // => 't0e1s2t'\n```\n#### ECMAScript 6: RegExp\nModules [`es6.regexp.constructor`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.constructor.js) and [`es6.regexp.flags`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.flags.js).\n\nSupport well-known [symbols](#ecmascript-6-symbol) `@@match`, `@@replace`, `@@search` and `@@split`, modules [`es6.regexp.match`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.match.js), [`es6.regexp.replace`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.replace.js), [`es6.regexp.search`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.search.js) and [`es6.regexp.split`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.regexp.split.js).\n```\nString\n #match(tpl) -> var, ES6 fix for support @@match\n #replace(tpl, replacer) -> var, ES6 fix for support @@replace\n #search(tpl) -> var, ES6 fix for support @@search\n #split(tpl, limit) -> var, ES6 fix for support @@split\n[new] RegExp(pattern, flags?) -> regexp, ES6 fix: can alter flags (IE9+)\n #flags -> str (IE9+)\n #@@match(str) -> array | null\n #@@replace(str, replacer) -> string\n #@@search(str) -> index\n #@@split(str, limit) -> array\n```\n[Examples](http://goo.gl/vLV603):\n```javascript\nRegExp(/./g, 'm'); // => /./m\n\n/foo/.flags; // => ''\n/foo/gim.flags; // => 'gim'\n\n'foo'.match({[Symbol.match]: _ => 1}); // => 1\n'foo'.replace({[Symbol.replace]: _ => 2}); // => 2\n'foo'.search({[Symbol.search]: _ => 3}); // => 3\n'foo'.split({[Symbol.split]: _ => 4}); // => 4\n```\n#### ECMAScript 6: Number\nModule [`es6.number.constructor`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.constructor.js). `Number` constructor support binary and octal literals, [example](http://goo.gl/jRd6b3):\n```javascript\nNumber('0b1010101'); // => 85\nNumber('0o7654321'); // => 2054353\n```\n`Number`: modules [`es6.number.epsilon`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.epsilon.js), [`es6.number.is-finite`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.is-finite.js), [`es6.number.is-integer`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.is-integer.js), [`es6.number.is-nan`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.is-nan.js), [`es6.number.is-safe-integer`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.is-safe-integer.js), [`es6.number.max-safe-integer`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.max-safe-integer.js), [`es6.number.min-safe-integer`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.min-safe-integer.js), [`es6.number.parse-float`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.parse-float.js), [`es6.number.parse-int`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.number.parse-int.js).\n```javascript\n[new] Number(var) -> number | number object\n .EPSILON -> num\n .isFinite(num) -> bool\n .isInteger(num) -> bool\n .isNaN(num) -> bool\n .isSafeInteger(num) -> bool\n .MAX_SAFE_INTEGER -> int\n .MIN_SAFE_INTEGER -> int\n .parseFloat(str) -> num\n .parseInt(str) -> int\n```\n#### ECMAScript 6: Math\n`Math`: modules [`es6.math.acosh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.acosh.js), [`es6.math.asinh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.asinh.js), [`es6.math.atanh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.atanh.js), [`es6.math.cbrt`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.cbrt.js), [`es6.math.clz32`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.clz32.js), [`es6.math.cosh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.cosh.js), [`es6.math.expm1`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.expm1.js), [`es6.math.fround`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.fround.js), [`es6.math.hypot`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.hypot.js), [`es6.math.imul`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.imul.js), [`es6.math.log10`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.log10.js), [`es6.math.log1p`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.log1p.js), [`es6.math.log2`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.log2.js), [`es6.math.sign`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.sign.js), [`es6.math.sinh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.sinh.js), [`es6.math.tanh`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.tanh.js), [`es6.math.trunc`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.math.trunc.js).\n```javascript\nMath\n .acosh(num) -> num\n .asinh(num) -> num\n .atanh(num) -> num\n .cbrt(num) -> num\n .clz32(num) -> uint\n .cosh(num) -> num\n .expm1(num) -> num\n .fround(num) -> num\n .hypot(...args) -> num\n .imul(num, num) -> int\n .log1p(num) -> num\n .log10(num) -> num\n .log2(num) -> num\n .sign(num) -> 1 | -1 | 0 | -0 | NaN\n .sinh(num) -> num\n .tanh(num) -> num\n .trunc(num) -> num\n```\n\n#### ECMAScript 6: Symbol\nModule [`es6.symbol`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.symbol.js).\n```javascript\nSymbol(description?) -> symbol\n .hasInstance -> @@hasInstance\n .isConcatSpreadable -> @@isConcatSpreadable\n .iterator -> @@iterator\n .match -> @@match\n .replace -> @@replace\n .search -> @@search\n .species -> @@species\n .split -> @@split\n .toPrimitive -> @@toPrimitive\n .toStringTag -> @@toStringTag\n .unscopables -> @@unscopables\n .for(key) -> symbol\n .keyFor(symbol) -> key\n .useSimple() -> void\n .useSetter() -> void\nObject\n .getOwnPropertySymbols(object) -> array\n```\nAlso wrapped some methods for correct work with `Symbol` polyfill.\n```js\nObject\n .create(proto | null, descriptors?) -> object\n .defineProperty(target, key, desc) -> target\n .defineProperties(target, descriptors) -> target\n .getOwnPropertyDescriptor(var, key) -> desc | undefined\n .getOwnPropertyNames(var) -> array\n #propertyIsEnumerable(key) -> bool\nJSON\n .stringify(target, replacer?, space?) -> string | undefined\n```\n[Basic example](http://goo.gl/BbvWFc):\n```javascript\nvar Person = (function(){\n var NAME = Symbol('name');\n function Person(name){\n this[NAME] = name;\n }\n Person.prototype.getName = function(){\n return this[NAME];\n };\n return Person;\n})();\n\nvar person = new Person('Vasya');\nlog(person.getName()); // => 'Vasya'\nlog(person['name']); // => undefined\nlog(person[Symbol('name')]); // => undefined, symbols are uniq\nfor(var key in person)log(key); // => only 'getName', symbols are not enumerable\n```\n`Symbol.for` & `Symbol.keyFor` [example](http://goo.gl/0pdJjX):\n```javascript\nvar symbol = Symbol.for('key');\nsymbol === Symbol.for('key'); // true\nSymbol.keyFor(symbol); // 'key'\n```\n[Example](http://goo.gl/mKVOQJ) with methods for getting own object keys:\n```javascript\nvar O = {a: 1};\nObject.defineProperty(O, 'b', {value: 2});\nO[Symbol('c')] = 3;\nObject.keys(O); // => ['a']\nObject.getOwnPropertyNames(O); // => ['a', 'b']\nObject.getOwnPropertySymbols(O); // => [Symbol(c)]\nReflect.ownKeys(O); // => ['a', 'b', Symbol(c)]\n```\n#### Caveats when using `Symbol` polyfill:\n\n* We can't add new primitive type, `Symbol` returns object.\n* `Symbol.for` and `Symbol.keyFor` can't be shimmed cross-realm.\n* By default, to hide the keys, `Symbol` polyfill defines setter in `Object.prototype`. For this reason, uncontrolled creation of symbols can cause memory leak and the `in` operator is not working correctly with `Symbol` polyfill: `Symbol() in {} // => true`.\n\nYou can disable defining setters in `Object.prototype`. [Example](http://goo.gl/N5UD7J):\n```javascript\nSymbol.useSimple();\nvar s1 = Symbol('s1')\n , o1 = {};\no1[s1] = true;\nfor(var key in o1)log(key); // => 'Symbol(s1)_t.qamkg9f3q', w/o native Symbol\n\nSymbol.useSetter();\nvar s2 = Symbol('s2')\n , o2 = {};\no2[s2] = true;\nfor(var key in o2)log(key); // nothing\n```\n* Currently, `core-js` not adds setters to `Object.prototype` for well-known symbols for correct work something like `Symbol.iterator in foo`. It can cause problems with their enumerability.\n\n#### ECMAScript 6: Collections\n`core-js` uses native collections in most case, just fixes methods / constructor, if it's required, and in old environment uses fast polyfill (O(1) lookup).\n#### Map\nModule [`es6.map`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.map.js). About iterators from this module [here](#ecmascript-6-iterators).\n```javascript\nnew Map(iterable (entries) ?) -> map\n #clear() -> void\n #delete(key) -> bool\n #forEach(fn(val, key, @), that) -> void\n #get(key) -> val\n #has(key) -> bool\n #set(key, val) -> @\n #size -> uint\n```\n[Example](http://goo.gl/RDbROF):\n```javascript\nvar a = [1];\n\nvar map = new Map([['a', 1], [42, 2]]);\nmap.set(a, 3).set(true, 4);\n\nlog(map.size); // => 4\nlog(map.has(a)); // => true\nlog(map.has([1])); // => false\nlog(map.get(a)); // => 3\nmap.forEach(function(val, key){\n log(val); // => 1, 2, 3, 4\n log(key); // => 'a', 42, [1], true\n});\nmap.delete(a);\nlog(map.size); // => 3\nlog(map.get(a)); // => undefined\nlog(Array.from(map)); // => [['a', 1], [42, 2], [true, 4]]\n```\n#### Set\nModule [`es6.set`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.set.js). About iterators from this module [here](#ecmascript-6-iterators).\n```javascript\nnew Set(iterable?) -> set\n #add(key) -> @\n #clear() -> void\n #delete(key) -> bool\n #forEach(fn(el, el, @), that) -> void\n #has(key) -> bool\n #size -> uint\n```\n[Example](http://goo.gl/7XYya3):\n```javascript\nvar set = new Set(['a', 'b', 'a', 'c']);\nset.add('d').add('b').add('e');\nlog(set.size); // => 5\nlog(set.has('b')); // => true\nset.forEach(function(it){\n log(it); // => 'a', 'b', 'c', 'd', 'e'\n});\nset.delete('b');\nlog(set.size); // => 4\nlog(set.has('b')); // => false\nlog(Array.from(set)); // => ['a', 'c', 'd', 'e']\n```\n#### WeakMap\nModule [`es6.weak-map`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.weak-map.js).\n```javascript\nnew WeakMap(iterable (entries) ?) -> weakmap\n #delete(key) -> bool\n #get(key) -> val\n #has(key) -> bool\n #set(key, val) -> @\n```\n[Example](http://goo.gl/SILXyw):\n```javascript\nvar a = [1]\n , b = [2]\n , c = [3];\n\nvar wmap = new WeakMap([[a, 1], [b, 2]]);\nwmap.set(c, 3).set(b, 4);\nlog(wmap.has(a)); // => true\nlog(wmap.has([1])); // => false\nlog(wmap.get(a)); // => 1\nwmap.delete(a);\nlog(wmap.get(a)); // => undefined\n\n// Private properties store:\nvar Person = (function(){\n var names = new WeakMap;\n function Person(name){\n names.set(this, name);\n }\n Person.prototype.getName = function(){\n return names.get(this);\n };\n return Person;\n})();\n\nvar person = new Person('Vasya');\nlog(person.getName()); // => 'Vasya'\nfor(var key in person)log(key); // => only 'getName'\n```\n#### WeakSet\nModule [`es6.weak-set`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.weak-set.js).\n```javascript\nnew WeakSet(iterable?) -> weakset\n #add(key) -> @\n #delete(key) -> bool\n #has(key) -> bool\n```\n[Example](http://goo.gl/TdFbEx):\n```javascript\nvar a = [1]\n , b = [2]\n , c = [3];\n\nvar wset = new WeakSet([a, b, a]);\nwset.add(c).add(b).add(c);\nlog(wset.has(b)); // => true\nlog(wset.has([2])); // => false\nwset.delete(b);\nlog(wset.has(b)); // => false\n```\n#### Caveats when using collections polyfill:\n\n* Frozen objects as collection keys are supported, but not recomended - it's slow (O(n) instead of O(1)) and, for weak-collections, leak.\n* Weak-collections polyfill stores values as hidden properties of keys. It works correct and not leak in most cases. However, it is desirable to store a collection longer than its keys.\n\n#### ECMAScript 6: Iterators\nModules [`es6.string.iterator`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.string.iterator.js) and [`es6.array.iterator`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.array.iterator.js):\n```javascript\nString\n #@@iterator() -> iterator\nArray\n #values() -> iterator\n #keys() -> iterator\n #entries() -> iterator (entries)\n #@@iterator() -> iterator\nArguments\n #@@iterator() -> iterator (available only in core-js methods)\n```\nModules [`es6.map`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.map.js) and [`es6.set`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.set.js):\n```javascript\nMap\n #values() -> iterator\n #keys() -> iterator\n #entries() -> iterator (entries)\n #@@iterator() -> iterator (entries)\nSet\n #values() -> iterator\n #keys() -> iterator\n #entries() -> iterator (entries)\n #@@iterator() -> iterator\n```\nModule [`web.dom.iterable`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/web.dom.iterable.js):\n```javascript\nNodeList\n #@@iterator() -> iterator\n```\n[Example](http://goo.gl/nzHVQF):\n```javascript\nvar string = 'a𠮷b';\n\nfor(var val of string)log(val); // => 'a', '𠮷', 'b'\n\nvar array = ['a', 'b', 'c'];\n\nfor(var val of array)log(val); // => 'a', 'b', 'c'\nfor(var val of array.values())log(val); // => 'a', 'b', 'c'\nfor(var key of array.keys())log(key); // => 0, 1, 2\nfor(var [key, val] of array.entries()){\n log(key); // => 0, 1, 2\n log(val); // => 'a', 'b', 'c'\n}\n\nvar map = new Map([['a', 1], ['b', 2], ['c', 3]]);\n\nfor(var [key, val] of map){\n log(key); // => 'a', 'b', 'c'\n log(val); // => 1, 2, 3\n}\nfor(var val of map.values())log(val); // => 1, 2, 3\nfor(var key of map.keys())log(key); // => 'a', 'b', 'c'\nfor(var [key, val] of map.entries()){\n log(key); // => 'a', 'b', 'c'\n log(val); // => 1, 2, 3\n}\n\nvar set = new Set([1, 2, 3, 2, 1]);\n\nfor(var val of set)log(val); // => 1, 2, 3\nfor(var val of set.values())log(val); // => 1, 2, 3\nfor(var key of set.keys())log(key); // => 1, 2, 3\nfor(var [key, val] of set.entries()){\n log(key); // => 1, 2, 3\n log(val); // => 1, 2, 3\n}\n\nfor(var x of document.querySelectorAll('*')){\n log(x.id);\n}\n```\nModules [`core.is-iterable`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.is-iterable.js), [`core.get-iterator`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.get-iterator.js), [`core.get-iterator-method`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.get-iterator-method.js) - helpers for check iterable / get iterator in `library` version or, for example, for `arguments` object:\n```javascript\ncore\n .isIterable(var) -> bool\n .getIterator(iterable) -> iterator\n .getIteratorMethod(var) -> function | undefined\n```\n[Example](http://goo.gl/SXsM6D):\n```js\nvar list = (function(){\n return arguments;\n})(1, 2, 3);\n\nlog(core.isIterable(list)); // true;\n\nvar iter = core.getIterator(list);\nlog(iter.next().value); // 1\nlog(iter.next().value); // 2\nlog(iter.next().value); // 3\nlog(iter.next().value); // undefined\n\ncore.getIterator({}); // TypeError: [object Object] is not iterable!\n\nvar iterFn = core.getIteratorMethod(list);\nlog(typeof iterFn); // 'function'\nvar iter = iterFn.call(list);\nlog(iter.next().value); // 1\nlog(iter.next().value); // 2\nlog(iter.next().value); // 3\nlog(iter.next().value); // undefined\n\nlog(core.getIteratorMethod({})); // undefined\n```\n#### ECMAScript 6: Promise\nModule [`es6.promise`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.promise.js).\n```javascript\nnew Promise(executor(resolve(var), reject(var))) -> promise\n #then(resolved(var), rejected(var)) -> promise\n #catch(rejected(var)) -> promise\n .resolve(var || promise) -> promise\n .reject(var) -> promise\n .all(iterable) -> promise\n .race(iterable) -> promise\n```\nBasic [example](http://goo.gl/vGrtUC):\n```javascript\nfunction sleepRandom(time){\n return new Promise(function(resolve, reject){\n setTimeout(resolve, time * 1e3, 0 | Math.random() * 1e3);\n });\n}\n\nlog('Run'); // => Run\nsleepRandom(5).then(function(result){\n log(result); // => 869, after 5 sec.\n return sleepRandom(10);\n}).then(function(result){\n log(result); // => 202, after 10 sec.\n}).then(function(){\n log('immediately after'); // => immediately after\n throw Error('Irror!');\n}).then(function(){\n log('will not be displayed');\n}).catch(log); // => => Error: Irror!\n```\n`Promise.resolve` and `Promise.reject` [example](http://goo.gl/vr8TN3):\n```javascript\nPromise.resolve(42).then(log); // => 42\nPromise.reject(42).catch(log); // => 42\n\nPromise.resolve($.getJSON('/data.json')); // => ES6 promise\n```\n`Promise.all` [example](http://goo.gl/RdoDBZ):\n```javascript\nPromise.all([\n 'foo',\n sleepRandom(5),\n sleepRandom(15),\n sleepRandom(10) // after 15 sec:\n]).then(log); // => ['foo', 956, 85, 382]\n```\n`Promise.race` [example](http://goo.gl/L8ovkJ):\n```javascript\nfunction timeLimit(promise, time){\n return Promise.race([promise, new Promise(function(resolve, reject){\n setTimeout(reject, time * 1e3, Error('Await > ' + time + ' sec'));\n })]);\n}\n\ntimeLimit(sleepRandom(5), 10).then(log); // => 853, after 5 sec.\ntimeLimit(sleepRandom(15), 10).catch(log); // Error: Await > 10 sec\n```\nECMAScript 7 [async functions](https://tc39.github.io/ecmascript-asyncawait) [example](http://goo.gl/wnQS4j):\n```javascript\nvar delay = time => new Promise(resolve => setTimeout(resolve, time))\n\nasync function sleepRandom(time){\n await delay(time * 1e3);\n return 0 | Math.random() * 1e3;\n};\nasync function sleepError(time, msg){\n await delay(time * 1e3);\n throw Error(msg);\n};\n\n(async () => {\n try {\n log('Run'); // => Run\n log(await sleepRandom(5)); // => 936, after 5 sec.\n var [a, b, c] = await Promise.all([\n sleepRandom(5),\n sleepRandom(15),\n sleepRandom(10)\n ]);\n log(a, b, c); // => 210 445 71, after 15 sec.\n await sleepError(5, 'Irror!');\n log('Will not be displayed');\n } catch(e){\n log(e); // => Error: 'Irror!', after 5 sec.\n }\n})();\n```\n\n##### Unhandled rejection tracking\n\n`core-js` `Promise` supports (but not adds to native implementations) unhandled rejection tracking.\n\n[Node.js](https://gist.github.com/benjamingr/0237932cee84712951a2):\n```js\nprocess.on('unhandledRejection', (reason, promise) => console.log(reason, promise));\nPromise.reject(42);\n// 42 [object Promise]\n\n```\nIn a browser, by default, you will see notify in the console, or you can add a custom handler, [example](http://goo.gl/izTr2I):\n```js\nwindow.onunhandledrejection = e => log(e.reason, e.promise);\nPromise.reject(42);\n// 42 [object Promise]\n```\n**Warning**: The problem here - we can't add it to native `Promise` implementations, but by idea `core-js` should use enough correct native implementation if it's available. Currently, most native implementations are buggy and `core-js` uses polyfill, but the situation will be changed. If someone wanna use this hook everywhere - he should delete `window.Promise` before inclusion `core-js`.\n\n\n#### ECMAScript 6: Reflect\nModules [`es6.reflect.apply`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.apply.js), [`es6.reflect.construct`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.construct.js), [`es6.reflect.define-property`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.define-property.js), [`es6.reflect.delete-property`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.delete-property.js), [`es6.reflect.enumerate`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.enumerate.js), [`es6.reflect.get`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.get.js), [`es6.reflect.get-own-property-descriptor`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.get-own-property-descriptor.js), [`es6.reflect.get-prototype-of`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.get-prototype-of.js), [`es6.reflect.has`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.has.js), [`es6.reflect.is-extensible`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.is-extensible.js), [`es6.reflect.own-keys`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.own-keys.js), [`es6.reflect.prevent-extensions`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.prevent-extensions.js), [`es6.reflect.set`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.set.js), [`es6.reflect.set-prototype-of`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es6.reflect.set-prototype-of.js).\n```javascript\nReflect\n .apply(target, thisArgument, argumentsList) -> var\n .construct(target, argumentsList, newTarget?) -> object\n .defineProperty(target, propertyKey, attributes) -> bool\n .deleteProperty(target, propertyKey) -> bool\n .enumerate(target) -> iterator\n .get(target, propertyKey, receiver?) -> var\n .getOwnPropertyDescriptor(target, propertyKey) -> desc\n .getPrototypeOf(target) -> object | null\n .has(target, propertyKey) -> bool\n .isExtensible(target) -> bool\n .ownKeys(target) -> array\n .preventExtensions(target) -> bool\n .set(target, propertyKey, V, receiver?) -> bool\n .setPrototypeOf(target, proto) -> bool (required __proto__ - IE11+)\n```\n[Example](http://goo.gl/gVT0cH):\n```javascript\nvar O = {a: 1};\nObject.defineProperty(O, 'b', {value: 2});\nO[Symbol('c')] = 3;\nReflect.ownKeys(O); // => ['a', 'b', Symbol(c)]\n\nfunction C(a, b){\n this.c = a + b;\n}\n\nvar instance = Reflect.construct(C, [20, 22]);\ninstance.c; // => 42\n```\n### ECMAScript 7\n* `Array#includes` [proposal](https://github.com/domenic/Array.prototype.includes) - module [`es7.array.includes`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.array.includes.js)\n* `String#at` [proposal](https://github.com/mathiasbynens/String.prototype.at) - module [`es7.string.at`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.string.at.js)\n* `String#padLeft`, `String#padRight` [proposal](https://github.com/ljharb/proposal-string-pad-left-right) - modules [`es7.string.pad-left`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.string.pad-left.js), [`es7.string.pad-right`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.string.pad-right.js)\n* `String#trimLeft`, `String#trimRight` [proposal](https://github.com/sebmarkbage/ecmascript-string-left-right-trim) - modules [`es7.string.trim-left`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.string.trim-right.js), [`es7.string.trim-right`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.string.trim-right.js)\n* `Object.values`, `Object.entries` [proposal](https://github.com/ljharb/proposal-object-values-entries) - modules [`es7.object.values`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.object.values.js), [`es7.object.entries`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.object.entries.js)\n* `Object.getOwnPropertyDescriptors` [proposal](https://gist.github.com/WebReflection/9353781) - module [`es7.object.get-own-property-descriptors`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.object.get-own-property-descriptors.js)\n* `RegExp.escape` [proposal](https://github.com/benjamingr/RexExp.escape) - module [`es7.regexp.escape`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.regexp.escape.js)\n* `Map#toJSON`, `Set#toJSON` [proposal](https://github.com/DavidBruant/Map-Set.prototype.toJSON) - modules [`es7.map.to-json`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.map.to-json.js), [`es7.set.to-json`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/es7.set.to-json.js)\n\n```javascript\nArray\n #includes(var, from?) -> bool\nString\n #at(index) -> string\n #padLeft(length, fillStr = ' ') -> string\n #padRight(length, fillStr = ' ') -> string\n #trimLeft() -> string\n #trimRight() -> string\nObject\n .values(object) -> array\n .entries(object) -> array\n .getOwnPropertyDescriptors(object) -> object\nRegExp\n .escape(str) -> str\nMap\n #toJSON() -> array\nSet\n #toJSON() -> array\n```\n[Examples](http://goo.gl/aUZQRH):\n```javascript\n[1, 2, 3].includes(2); // => true\n[1, 2, 3].includes(4); // => false\n[1, 2, 3].includes(2, 2); // => false\n\n[NaN].indexOf(NaN); // => -1\n[NaN].includes(NaN); // => true\nArray(1).indexOf(undefined); // => -1\nArray(1).includes(undefined); // => true\n\n'a𠮷b'.at(1); // => '𠮷'\n'a𠮷b'.at(1).length; // => 2\n\n'hello'.padLeft(10); // => ' hello'\n'hello'.padLeft(10, '1234'); // => '41234hello'\n'hello'.padRight(10); // => 'hello '\n'hello'.padRight(10, '1234'); // => 'hello12341'\n\n' hello '.trimLeft(); // => 'hello '\n' hello '.trimRight(); // => ' hello'\n\nObject.values({a: 1, b: 2, c: 3}); // => [1, 2, 3]\nObject.entries({a: 1, b: 2, c: 3}); // => [['a', 1], ['b', 2], ['c', 3]]\n\n// Shallow object cloning with prototype and descriptors:\nvar copy = Object.create(Object.getPrototypeOf(O), Object.getOwnPropertyDescriptors(O));\n// Mixin:\nObject.defineProperties(target, Object.getOwnPropertyDescriptors(source));\n\nRegExp.escape('Hello, []{}()*+?.\\\\^$|!'); // => 'Hello, \\[\\]\\{\\}\\(\\)\\*\\+\\?\\.\\\\\\^\\$\\|!'\n\nJSON.stringify(new Map([['a', 'b'], ['c', 'd']])); // => '[[\"a\",\"b\"],[\"c\",\"d\"]]'\nJSON.stringify(new Set([1, 2, 3, 2, 1])); // => '[1,2,3]'\n```\n### Mozilla JavaScript: Array generics\nModule [`js.array.statics`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/js.array.statics.js).\n```javascript\nArray\n .{...ArrayPrototype methods}\n```\n\n```javascript\nArray.slice(arguments, 1);\n\nArray.join('abcdef', '+'); // => 'a+b+c+d+e+f'\n\nvar form = document.getElementsByClassName('form__input');\nArray.reduce(form, function(memo, it){\n memo[it.name] = it.value;\n return memo;\n}, {}); // => {name: 'Vasya', age: '42', sex: 'yes, please'}\n```\n### Web standards\n#### setTimeout / setInterval\nModule [`web.timers`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/web.timers.js). Additional arguments fix for IE9-.\n```javascript\nsetTimeout(fn(...args), time, ...args) -> id\nsetInterval(fn(...args), time, ...args) -> id\n```\n```javascript\n// Before:\nsetTimeout(log.bind(null, 42), 1000);\n// After:\nsetTimeout(log, 1000, 42);\n```\n#### setImmediate\nModule [`web.immediate`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/web.immediate.js). [`setImmediate` proposal](https://developer.mozilla.org/en-US/docs/Web/API/Window.setImmediate) polyfill.\n```javascript\nsetImmediate(fn(...args), ...args) -> id\nclearImmediate(id) -> void\n```\n[Example](http://goo.gl/6nXGrx):\n```javascript\nsetImmediate(function(arg1, arg2){\n log(arg1, arg2); // => Message will be displayed with minimum delay\n}, 'Message will be displayed', 'with minimum delay');\n\nclearImmediate(setImmediate(function(){\n log('Message will not be displayed');\n}));\n```\n### Non-standard\n#### Object\nModules [`core.object.is-object`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.object.is-object.js), [`core.object.classof`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.object.classof.js), [`core.object.define`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.object.define.js), [`core.object.make`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.object.make.js).\n```javascript\nObject\n .isObject(var) -> bool\n .classof(var) -> string \n .define(target, mixin) -> target\n .make(proto | null, mixin?) -> object\n```\nObject classify [examples](http://goo.gl/YZQmGo):\n```javascript\nObject.isObject({}); // => true\nObject.isObject(isNaN); // => true\nObject.isObject(null); // => false\n\nvar classof = Object.classof;\n\nclassof(null); // => 'Null'\nclassof(undefined); // => 'Undefined'\nclassof(1); // => 'Number'\nclassof(true); // => 'Boolean'\nclassof('string'); // => 'String'\nclassof(Symbol()); // => 'Symbol'\n\nclassof(new Number(1)); // => 'Number'\nclassof(new Boolean(true)); // => 'Boolean'\nclassof(new String('string')); // => 'String'\n\nvar fn = function(){}\n , list = (function(){return arguments})(1, 2, 3);\n\nclassof({}); // => 'Object'\nclassof(fn); // => 'Function'\nclassof([]); // => 'Array'\nclassof(list); // => 'Arguments'\nclassof(/./); // => 'RegExp'\nclassof(new TypeError); // => 'Error'\n\nclassof(new Set); // => 'Set'\nclassof(new Map); // => 'Map'\nclassof(new WeakSet); // => 'WeakSet'\nclassof(new WeakMap); // => 'WeakMap'\nclassof(new Promise(fn)); // => 'Promise'\n\nclassof([].values()); // => 'Array Iterator'\nclassof(new Set().values()); // => 'Set Iterator'\nclassof(new Map().values()); // => 'Map Iterator'\n\nclassof(Math); // => 'Math'\nclassof(JSON); // => 'JSON'\n\nfunction Example(){}\nExample.prototype[Symbol.toStringTag] = 'Example';\n\nclassof(new Example); // => 'Example'\n```\n`Object.define` and `Object.make` [examples](http://goo.gl/rtpD5Z):\n```javascript\n// Before:\nObject.defineProperty(target, 'c', {\n enumerable: true,\n configurable: true,\n get: function(){\n return this.a + this.b;\n }\n});\n\n// After:\nObject.define(target, {\n get c(){\n return this.a + this.b;\n }\n});\n\n// Shallow object cloning with prototype and descriptors:\nvar copy = Object.make(Object.getPrototypeOf(src), src);\n\n// Simple inheritance:\nfunction Vector2D(x, y){\n this.x = x;\n this.y = y;\n}\nObject.define(Vector2D.prototype, {\n get xy(){\n return Math.hypot(this.x, this.y);\n }\n});\nfunction Vector3D(x, y, z){\n Vector2D.apply(this, arguments);\n this.z = z;\n}\nVector3D.prototype = Object.make(Vector2D.prototype, {\n constructor: Vector3D,\n get xyz(){\n return Math.hypot(this.x, this.y, this.z);\n }\n});\n\nvar vector = new Vector3D(9, 12, 20);\nlog(vector.xy); // => 15\nlog(vector.xyz); // => 25\nvector.y++;\nlog(vector.xy); // => 15.811388300841896\nlog(vector.xyz); // => 25.495097567963924\n```\n#### Dict\nModule [`core.dict`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.dict.js). Based on [TC39 discuss](https://github.com/rwaldron/tc39-notes/blob/master/es6/2012-11/nov-29.md#collection-apis-review) / [strawman](http://wiki.ecmascript.org/doku.php?id=harmony:modules_standard#dictionaries).\n```javascript\n[new] Dict(iterable (entries) | object ?) -> dict\n .isDict(var) -> bool\n .values(object) -> iterator\n .keys(object) -> iterator\n .entries(object) -> iterator (entries)\n .has(object, key) -> bool\n .get(object, key) -> val\n .set(object, key, value) -> object\n .forEach(object, fn(val, key, @), that) -> void\n .map(object, fn(val, key, @), that) -> new @\n .mapPairs(object, fn(val, key, @), that) -> new @\n .filter(object, fn(val, key, @), that) -> new @\n .some(object, fn(val, key, @), that) -> bool\n .every(object, fn(val, key, @), that) -> bool\n .find(object, fn(val, key, @), that) -> val\n .findKey(object, fn(val, key, @), that) -> key\n .keyOf(object, var) -> key\n .includes(object, var) -> bool\n .reduce(object, fn(memo, val, key, @), memo?) -> var\n```\n`Dict` create object without prototype from iterable or simple object. [Example](http://goo.gl/pnp8Vr):\n```javascript\nvar map = new Map([['a', 1], ['b', 2], ['c', 3]]);\n\nDict(); // => {__proto__: null}\nDict({a: 1, b: 2, c: 3}); // => {__proto__: null, a: 1, b: 2, c: 3}\nDict(map); // => {__proto__: null, a: 1, b: 2, c: 3}\nDict([1, 2, 3].entries()); // => {__proto__: null, 0: 1, 1: 2, 2: 3}\n\nvar dict = Dict({a: 42});\ndict instanceof Object; // => false\ndict.a; // => 42\ndict.toString; // => undefined\n'a' in dict; // => true\n'hasOwnProperty' in dict; // => false\n\nDict.isDict({}); // => false\nDict.isDict(Dict()); // => true\n```\n`Dict.keys`, `Dict.values` and `Dict.entries` returns iterators for objects, [examples](http://goo.gl/xAvECH):\n```javascript\nvar dict = {a: 1, b: 2, c: 3};\n\nfor(var key of Dict.keys(dict))log(key); // => 'a', 'b', 'c'\n\nfor(var val of Dict.values(dict))log(val); // => 1, 2, 3\n\nfor(var [key, val] of Dict.entries(dict)){\n log(key); // => 'a', 'b', 'c'\n log(val); // => 1, 2, 3\n}\n\nnew Map(Dict.entries(dict)); // => Map {a: 1, b: 2, c: 3}\n```\nBasic dict operations for objects with prototype [example](http://goo.gl/B28UnG):\n```js\n'q' in {q: 1}; // => true\n'toString' in {}; // => true\n\nDict.has({q: 1}, 'q'); // => true\nDict.has({}, 'toString'); // => false\n\n({q: 1})['q']; // => 1\n({}).toString; // => function toString(){ [native code] }\n\nDict.get({q: 1}, 'q'); // => 1\nDict.get({}, 'toString'); // => undefined\n\nvar O = {};\nO['q'] = 1;\nO['q']; // => 1\nO['__proto__'] = {w: 2};\nO['__proto__']; // => {w: 2}\nO['w']; // => 2\n\nvar O = {};\nDict.set(O, 'q', 1);\nO['q']; // => 1\nDict.set(O, '__proto__', {w: 2});\nO['__proto__']; // => {w: 2}\nO['w']; // => undefined\n```\nOther methods of `Dict` module are static equialents of `Array.prototype` methods for dictionaries, [examples](http://goo.gl/xFi1RH):\n```javascript\nvar dict = {a: 1, b: 2, c: 3};\n\nDict.forEach(dict, console.log, console);\n// => 1, 'a', {a: 1, b: 2, c: 3}\n// => 2, 'b', {a: 1, b: 2, c: 3}\n// => 3, 'c', {a: 1, b: 2, c: 3}\n\nDict.map(dict, function(it){\n return it * it;\n}); // => {a: 1, b: 4, c: 9}\n\nDict.mapPairs(dict, function(val, key){\n if(key != 'b')return [key + key, val * val];\n}); // => {aa: 1, cc: 9}\n\nDict.filter(dict, function(it){\n return it % 2;\n}); // => {a: 1, c: 3}\n\nDict.some(dict, function(it){\n return it === 2;\n}); // => true\n\nDict.every(dict, function(it){\n return it === 2;\n}); // => false\n\nDict.find(dict, function(it){\n return it > 2;\n}); // => 3\nDict.find(dict, function(it){\n return it > 4;\n}); // => undefined\n\nDict.findKey(dict, function(it){\n return it > 2;\n}); // => 'c'\nDict.findKey(dict, function(it){\n return it > 4;\n}); // => undefined\n\nDict.keyOf(dict, 2); // => 'b'\nDict.keyOf(dict, 4); // => undefined\n\nDict.includes(dict, 2); // => true\nDict.includes(dict, 4); // => false\n\nDict.reduce(dict, function(memo, it){\n return memo + it;\n}); // => 6\nDict.reduce(dict, function(memo, it){\n return memo + it;\n}, ''); // => '123'\n```\n#### Partial application\nModule [`core.function.part`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.function.part.js).\n```javascript\nFunction\n #part(...args | _) -> fn(...args)\n```\n`Function#part` partial apply function without `this` binding. Uses global variable `_` (`core._` for builds without global namespace pollution) as placeholder and not conflict with `Underscore` / `LoDash`. [Examples](http://goo.gl/p9ZJ8K):\n```javascript\nvar fn1 = log.part(1, 2);\nfn1(3, 4); // => 1, 2, 3, 4\n\nvar fn2 = log.part(_, 2, _, 4);\nfn2(1, 3); // => 1, 2, 3, 4\n\nvar fn3 = log.part(1, _, _, 4);\nfn3(2, 3); // => 1, 2, 3, 4\n\nfn2(1, 3, 5); // => 1, 2, 3, 4, 5\nfn2(1); // => 1, 2, undefined, 4\n```\n#### Number Iterator\nModules [`core.number.iterator`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.number.iterator.js).\n```javascript\nNumber\n #@@iterator() -> iterator\n```\n[Examples](http://goo.gl/o45pCN):\n```javascript\nfor(var i of 3)log(i); // => 0, 1, 2\n\n[...10]; // => [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]\n\nArray.from(10, Math.random); // => [0.9817775336559862, 0.02720663254149258, ...]\n\nArray.from(10, function(it){\n return this + it * it;\n}, .42); // => [0.42, 1.42, 4.42, 9.42, 16.42, 25.42, 36.42, 49.42, 64.42, 81.42]\n```\n#### Escaping HTML\nModules [`core.string.escape-html`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.string.escape-html.js) and [`core.string.unescape-html`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.string.unescape-html.js).\n```javascript\nString\n #escapeHTML() -> str\n #unescapeHTML() -> str\n```\n[Examples](http://goo.gl/6bOvsQ):\n```javascript\n''.escapeHTML(); // => '<script>doSomething();</script>'\n'<script>doSomething();</script>'.unescapeHTML(); // => ''\n```\n#### delay\nModule [`core.delay`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.delay.js). [Promise](#ecmascript-6-promise)-returning delay function, [esdiscuss](https://esdiscuss.org/topic/promise-returning-delay-function). [Example](http://goo.gl/lbucba):\n```javascript\ndelay(1e3).then(() => log('after 1 sec'));\n\n(async () => {\n await delay(3e3);\n log('after 3 sec');\n \n while(await delay(3e3))log('each 3 sec');\n})();\n```\n#### Console\nModule [`core.log`](https://github.com/zloirock/core-js/blob/v1.2.6/modules/core.log.js). Console cap for old browsers and some additional functionality. In IE, Node.js / IO.js and Firebug `console` methods not require call from `console` object, but in Chromium and V8 this throws error. For some reason, we can't replace `console` methods by their bound versions. Add `log` object with bound console methods. Some more sugar: `log` is shortcut for `log.log`, we can disable output.\n```javascript\nlog ==== log.log\n .{...console API}\n .enable() -> void\n .disable() -> void\n```\n```javascript\n// Before:\nif(window.console && console.warn)console.warn(42);\n// After:\nlog.warn(42);\n\n// Before:\nsetTimeout(console.warn.bind(console, 42), 1000);\n[1, 2, 3].forEach(console.warn, console);\n// After:\nsetTimeout(log.warn, 1000, 42);\n[1, 2, 3].forEach(log.warn);\n\n// log is shortcut for log.log\nsetImmediate(log, 42); // => 42\n\nlog.disable();\nlog.warn('Console is disabled, you will not see this message.');\nlog.enable();\nlog.warn('Console is enabled again.');\n```\n\n## Missing polyfills\n- ES5 `JSON` is missing now only in IE7- and never it will be added to `core-js`, if you need it in these old browsers available many implementations, for example, [json3](https://github.com/bestiejs/json3).\n- ES6 Typed Arrays can be polyfilled without serious problems, but it will be slow - getter / setter for each element and they are missing completely only in IE9-. You can use [this polyfill](https://github.com/inexorabletash/polyfill/blob/master/typedarray.js). *Possible*, it will be added to `core-js` in the future, completely or only missing methods of existing arrays. \n- ES6 `String#normalize` is not very usefull feature, but this polyfill will be very large. If you need it, you can use [unorm](https://github.com/walling/unorm/).\n- ES6 `Proxy` can't be polyfilled, but for Node.js / Chromium with additional flags you can try [harmony-reflect](https://github.com/tvcutsem/harmony-reflect) for adapt old style `Proxy` API to final ES6 version.\n- ES6 logic for `@@isConcatSpreadable` and `@@species` (in most places) can be polyfilled without problems, but it will cause serious slowdown in popular cases in some engines. It will be polyfilled when it will be implemented in modern engines.\n- ES7 `Object.observe` can be polyfilled with many limitations, but it will be very slow - dirty checking on each tick. In nearest future it will not be added to `core-js` - it will cause serious slowdown in applications which uses `Object.observe` and fallback if it's missing. *Possible* it will be added as optional feature then most actual browsers will have this feature. Now you can use [this polyfill](https://github.com/MaxArt2501/object-observe).\n- ES7 `SIMD`. `core-js` doesn't adds polyfill of this feature because of large size and some other reasons. You can use [this polyfill](https://github.com/tc39/ecmascript_simd/blob/master/src/ecmascript_simd.js).\n- `window.fetch` is not crossplatform feature, in some environments it make no sense. For this reason I don't think it should be in `core-js`. Looking at the large number of requests it *maybe* added in the future. Now you can use, for example, [this polyfill](https://github.com/github/fetch).",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/zloirock/core-js/issues"
},
"_id": "core-js@1.2.6",
"_from": "core-js@^1.0.0",
"realName": "core-js",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/core-js",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/core-js",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
},
"loose-envify": {
"name": "loose-envify",
"version": "1.1.0",
"description": "Fast (and loose) selective `process.env` replacer using js-tokens instead of an AST",
"keywords": [
"environment",
"variables",
"browserify",
"browserify-transform",
"transform",
"source",
"configuration"
],
"homepage": "https://github.com/zertosh/loose-envify",
"license": "MIT",
"author": {
"name": "Andres Suarez",
"email": "zertosh@gmail.com"
},
"repository": {
"type": "git",
"url": "git://github.com/zertosh/loose-envify.git"
},
"scripts": {
"test": "tap test/*.js"
},
"dependencies": {
"js-tokens": {
"name": "js-tokens",
"version": "1.0.2",
"author": {
"name": "Simon Lydell"
},
"license": "MIT",
"description": "A regex that tokenizes JavaScript.",
"keywords": [
"JavaScript",
"js",
"token",
"tokenize",
"regex"
],
"files": [
"index.js"
],
"repository": {
"type": "git",
"url": "git://github.com/lydell/js-tokens"
},
"scripts": {
"test": "mocha --ui tdd",
"esprima-compare": "node esprima-compare ./index.js everything.js/es5.js",
"build": "node generate-index.js",
"dev": "npm run build && npm test"
},
"devDependencies": {
"coffee-script": "~1.9.3",
"esprima": "^2.3.0",
"everything.js": "^1.0.3",
"mocha": "^2.2.5"
},
"readme": "Overview [](https://travis-ci.org/lydell/js-tokens)\n========\n\nA regex that tokenizes JavaScript.\n\n```js\nvar jsTokens = require(\"js-tokens\")\n\nvar jsString = \"var foo=opts.foo;\\n...\"\n\njsString.match(jsTokens)\n// [\"var\", \" \", \"foo\", \"=\", \"opts\", \".\", \"foo\", \";\", \"\\n\", ...]\n```\n\n\nInstallation\n============\n\n- `npm install js-tokens`\n\n```js\nvar jsTokens = require(\"js-tokens\")\n```\n\n\nUsage\n=====\n\n### `jsTokens` ###\n\nA regex with the `g` flag that matches JavaScript tokens.\n\nThe regex _always_ matches, even invalid JavaScript and the empty string.\n\nThe next match is always directly after the previous.\n\n### `var token = jsTokens.matchToToken(match)` ###\n\nTakes a `match` returned by `jsTokens.exec(string)`, and returns a `{type:\nString, value: String}` object. The following types are available:\n\n- string\n- comment\n- regex\n- number\n- name\n- punctuator\n- whitespace\n- invalid\n\nMulti-line comments and strings also have a `closed` property indicating if the\ntoken was closed or not (see below).\n\nComments and strings both come in several flavors. To distinguish them, check if\nthe token starts with `//`, `/*`, `'`, `\"` or `` ` ``.\n\nNames are ECMAScript IdentifierNames, that is, including both identifiers and\nkeywords. You may use [is-keyword-js] to tell them apart.\n\nWhitespace includes both line terminators and other whitespace.\n\nFor example usage, please see this [gist].\n\n[is-keyword-js]: https://github.com/crissdev/is-keyword-js\n[gist]: https://gist.github.com/lydell/be49dbf80c382c473004\n\n\nInvalid code handling\n=====================\n\nUnterminated strings are still matched as strings. JavaScript strings cannot\ncontain (unescaped) newlines, so unterminated strings simply end at the end of\nthe line. Unterminated template strings can contain unescaped newlines, though,\nso they go on to the end of input.\n\nUnterminated multi-line comments are also still matched as comments. They\nsimply go on to the end of the input.\n\nUnterminated regex literals are likely matched as division and whatever is\ninside the regex.\n\nInvalid ASCII characters have their own capturing group.\n\nInvalid non-ASCII characters are treated as names, to simplify the matching of\nnames (except unicode spaces which are treated as whitespace).\n\nRegex literals may contain invalid regex syntax. They are still matched as\nregex literals. They may also contain repeated regex flags, to keep the regex\nsimple.\n\nStrings may contain invalid escape sequences.\n\n\nLimitations\n===========\n\nTokenizing JavaScript using regexes—in fact, _one single regex_—won’t be\nperfect. But that’s not the point either.\n\nYou may compare jsTokens with [esprima] by using `esprima-compare.js`.\nSee `npm run esprima-compare`!\n\n[esprima]: http://esprima.org/\n\n### Template string interpolation ###\n\nTemplate strings are matched as single tokens, from the starting `` ` `` to the\nending `` ` ``, including interpolations (whose tokens are not matched\nindividually).\n\nMatching template string interpolations requires recursive balancing of `{` and\n`}`—something that JavaScript regexes cannot do. Only one level of nesting is\nsupported.\n\n### Division and regex literals collision ###\n\nConsider this example:\n\n```js\nvar g = 9.82\nvar number = bar / 2/g\n\nvar regex = / 2/g\n```\n\nA human can easily understand that in the `number` line we’re dealing with\ndivision, and in the `regex` line we’re dealing with a regex literal. How come?\nBecause humans can look at the whole code to put the `/` characters in context.\nA JavaScript regex cannot. It only sees forwards.\n\nWhen the `jsTokens` regex scans throught the above, it will see the following\nat the end of both the `number` and `regex` rows:\n\n```js\n/ 2/g\n```\n\nIt is then impossible to know if that is a regex literal, or part of an\nexpression dealing with division.\n\nHere is a similar case:\n\n```js\nfoo /= 2/g\nfoo(/= 2/g)\n```\n\nThe first line divides the `foo` variable with `2/g`. The second line calls the\n`foo` function with the regex literal `/= 2/g`. Again, since `jsTokens` only\nsees forwards, it cannot tell the two cases apart.\n\nThere are some cases where we _can_ tell division and regex literals apart,\nthough.\n\nFirst off, we have the simple cases where there’s only one slash in the line:\n\n```js\nvar foo = 2/g\nfoo /= 2\n```\n\nRegex literals cannot contain newlines, so the above cases are correctly\nidentified as division. Things are only problematic when there are more than\none non-comment slash in a single line.\n\nSecondly, not every character is a valid regex flag.\n\n```js\nvar number = bar / 2/e\n```\n\nThe above example is also correctly identified as division, because `e` is not a\nvalid regex flag. I initially wanted to future-proof by allowing `[a-zA-Z]*`\n(any letter) as flags, but it is not worth it since it increases the amount of\nambigous cases. So only the standard `g`, `m`, `i`, `y` and `u` flags are\nallowed. This means that the above example will be identified as division as\nlong as you don’t rename the `e` variable to some permutation of `gmiyu` 1 to 5\ncharacters long.\n\nLastly, we can look _forward_ for information.\n\n- If the token following what looks like a regex literal is not valid after a\n regex literal, but is valid in a division expression, then the regex literal\n is treated as division instead. For example, a flagless regex cannot be\n followed by a string, number or name, but all of those three can be the\n denominator of a division.\n- Generally, if what looks like a regex literal is followed by an operator, the\n regex literal is treated as division instead. This is because regexes are\n seldomly used with operators (such as `+`, `*`, `&&` and `==`), but division\n could likely be part of such an expression.\n\nPlease consult the regex source and the test cases for precise information on\nwhen regex or division is matched (should you need to know). In short, you\ncould sum it up as:\n\nIf the end of a statement looks like a regex literal (even if it isn’t), it\nwill be treated as one. Otherwise it should work as expected (if you write sane\ncode).\n\n\nLicense\n=======\n\n[The X11 (“MIT”) License](LICENSE).\n",
"readmeFilename": "readme.md",
"bugs": {
"url": "https://github.com/lydell/js-tokens/issues"
},
"_id": "js-tokens@1.0.2",
"_from": "js-tokens@^1.0.1",
"realName": "js-tokens",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/loose-envify/node_modules/js-tokens",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/loose-envify/node_modules/js-tokens",
"parent": "[Circular]",
"depth": 4,
"peerDependencies": {}
}
},
"devDependencies": {
"browserify": "^11.0.1",
"envify": "^3.4.0",
"tap": "^1.4.0"
},
"readme": "# loose-envify\n\n[](https://travis-ci.org/zertosh/loose-envify)\n\nFast (and loose) selective `process.env` replacer using [js-tokens](https://github.com/lydell/js-tokens) instead of an AST. Works just like [envify](https://github.com/hughsk/envify) but much faster.\n\n## Gotchas\n\n* Doesn't handle broken syntax.\n* Doesn't look inside embedded expressions in template strings.\n - **this won't work:**\n ```js\n console.log(`the current env is ${process.env.NODE_ENV}`);\n ```\n* Doesn't replace oddly-spaced or oddly-commented expressions.\n - **this won't work:**\n ```js\n console.log(process./*won't*/env./*work*/NODE_ENV);\n ```\n\n## Usage/Options\n\nloose-envify has the exact same interface as [envify](https://github.com/hughsk/envify).\n\n## Benchmark\n\n```\nenvify:\n\n $ for i in {1..5}; do node bench/bench.js 'envify'; done\n 708ms\n 727ms\n 791ms\n 719ms\n 720ms\n\nloose-envify:\n\n $ for i in {1..5}; do node bench/bench.js '../'; done\n 51ms\n 52ms\n 52ms\n 52ms\n 52ms\n```\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/zertosh/loose-envify/issues"
},
"_id": "loose-envify@1.1.0",
"_from": "loose-envify@^1.0.0",
"realName": "loose-envify",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/loose-envify",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/loose-envify",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
},
"promise": {
"name": "promise",
"version": "7.1.1",
"description": "Bare bones Promises/A+ implementation",
"main": "index.js",
"scripts": {
"prepublish": "node build",
"pretest": "node build",
"pretest-resolve": "node build",
"pretest-extensions": "node build",
"pretest-memory-leak": "node build",
"test": "mocha --bail --timeout 200 --slow 99999 -R dot && npm run test-memory-leak",
"test-resolve": "mocha test/resolver-tests.js --timeout 200 --slow 999999",
"test-extensions": "mocha test/extensions-tests.js --timeout 200 --slow 999999",
"test-memory-leak": "node --expose-gc test/memory-leak.js",
"coverage": "istanbul cover node_modules/mocha/bin/_mocha -- --bail --timeout 200 --slow 99999 -R dot"
},
"repository": {
"type": "git",
"url": "https://github.com/then/promise.git"
},
"author": {
"name": "ForbesLindesay"
},
"license": "MIT",
"devDependencies": {
"acorn": "^1.0.1",
"better-assert": "*",
"istanbul": "^0.3.13",
"mocha": "*",
"promises-aplus-tests": "*",
"rimraf": "^2.3.2"
},
"dependencies": {
"asap": {
"name": "asap",
"version": "2.0.3",
"description": "High-priority task queue for Node.js and browsers",
"keywords": [
"event",
"task",
"queue"
],
"license": {
"type": "MIT",
"url": "https://github.com/kriskowal/asap/raw/master/LICENSE.md"
},
"repository": {
"type": "git",
"url": "https://github.com/kriskowal/asap.git"
},
"main": "./asap.js",
"browser": {
"./asap.js": "./browser-asap.js",
"./raw.js": "./browser-raw.js",
"./test/domain.js": "./test/browser-domain.js"
},
"files": [
"raw.js",
"asap.js",
"browser-raw.js",
"browser-asap.js"
],
"scripts": {
"test": "npm run lint && npm run test-node",
"test-travis": "npm run lint && npm run test-node && npm run test-saucelabs && npm run test-saucelabs-worker",
"test-node": "node test/asap-test.js",
"test-publish": "node scripts/publish-bundle.js test/asap-test.js | pbcopy",
"test-browser": "node scripts/publish-bundle.js test/asap-test.js | xargs opener",
"test-saucelabs": "node scripts/saucelabs.js test/asap-test.js scripts/saucelabs-spot-configurations.json",
"test-saucelabs-all": "node scripts/saucelabs.js test/asap-test.js scripts/saucelabs-all-configurations.json",
"test-saucelabs-worker": "node scripts/saucelabs-worker-test.js scripts/saucelabs-spot-configurations.json",
"test-saucelabs-worker-all": "node scripts/saucelabs-worker-test.js scripts/saucelabs-all-configurations.json",
"lint": "jshint raw.js asap.js browser-raw.js browser-asap.js $(find scripts -name '*.js' | grep -v gauntlet)"
},
"devDependencies": {
"events": "^1.0.1",
"jshint": "^2.5.1",
"knox": "^0.8.10",
"mr": "^2.0.5",
"opener": "^1.3.0",
"q": "^2.0.3",
"q-io": "^2.0.3",
"saucelabs": "^0.1.1",
"wd": "^0.2.21",
"weak-map": "^1.0.5"
},
"readme": "# ASAP\n\n[](https://travis-ci.org/kriskowal/asap)\n\nPromise and asynchronous observer libraries, as well as hand-rolled callback\nprograms and libraries, often need a mechanism to postpone the execution of a\ncallback until the next available event.\n(See [Designing API’s for Asynchrony][Zalgo].)\nThe `asap` function executes a task **as soon as possible** but not before it\nreturns, waiting only for the completion of the current event and previously\nscheduled tasks.\n\n```javascript\nasap(function () {\n // ...\n});\n```\n\n[Zalgo]: http://blog.izs.me/post/59142742143/designing-apis-for-asynchrony\n\nThis CommonJS package provides an `asap` module that exports a function that\nexecutes a task function *as soon as possible*.\n\nASAP strives to schedule events to occur before yielding for IO, reflow,\nor redrawing.\nEach event receives an independent stack, with only platform code in parent\nframes and the events run in the order they are scheduled.\n\nASAP provides a fast event queue that will execute tasks until it is\nempty before yielding to the JavaScript engine's underlying event-loop.\nWhen a task gets added to a previously empty event queue, ASAP schedules a flush\nevent, preferring for that event to occur before the JavaScript engine has an\nopportunity to perform IO tasks or rendering, thus making the first task and\nsubsequent tasks semantically indistinguishable.\nASAP uses a variety of techniques to preserve this invariant on different\nversions of browsers and Node.js.\n\nBy design, ASAP prevents input events from being handled until the task\nqueue is empty.\nIf the process is busy enough, this may cause incoming connection requests to be\ndropped, and may cause existing connections to inform the sender to reduce the\ntransmission rate or stall.\nASAP allows this on the theory that, if there is enough work to do, there is no\nsense in looking for trouble.\nAs a consequence, ASAP can interfere with smooth animation.\nIf your task should be tied to the rendering loop, consider using\n`requestAnimationFrame` instead.\nA long sequence of tasks can also effect the long running script dialog.\nIf this is a problem, you may be able to use ASAP’s cousin `setImmediate` to\nbreak long processes into shorter intervals and periodically allow the browser\nto breathe.\n`setImmediate` will yield for IO, reflow, and repaint events.\nIt also returns a handler and can be canceled.\nFor a `setImmediate` shim, consider [YuzuJS setImmediate][setImmediate].\n\n[setImmediate]: https://github.com/YuzuJS/setImmediate\n\nTake care.\nASAP can sustain infinite recursive calls without warning.\nIt will not halt from a stack overflow, and it will not consume unbounded\nmemory.\nThis is behaviorally equivalent to an infinite loop.\nJust as with infinite loops, you can monitor a Node.js process for this behavior\nwith a heart-beat signal.\nAs with infinite loops, a very small amount of caution goes a long way to\navoiding problems.\n\n```javascript\nfunction loop() {\n asap(loop);\n}\nloop();\n```\n\nIn browsers, if a task throws an exception, it will not interrupt the flushing\nof high-priority tasks.\nThe exception will be postponed to a later, low-priority event to avoid\nslow-downs.\nIn Node.js, if a task throws an exception, ASAP will resume flushing only if—and\nonly after—the error is handled by `domain.on(\"error\")` or\n`process.on(\"uncaughtException\")`.\n\n## Raw ASAP\n\nChecking for exceptions comes at a cost.\nThe package also provides an `asap/raw` module that exports the underlying\nimplementation which is faster but stalls if a task throws an exception.\nThis internal version of the ASAP function does not check for errors.\nIf a task does throw an error, it will stall the event queue unless you manually\ncall `rawAsap.requestFlush()` before throwing the error, or any time after.\n\nIn Node.js, `asap/raw` also runs all tasks outside any domain.\nIf you need a task to be bound to your domain, you will have to do it manually.\n\n```js\nif (process.domain) {\n task = process.domain.bind(task);\n}\nrawAsap(task);\n```\n\n## Tasks\n\nA task may be any object that implements `call()`.\nA function will suffice, but closures tend not to be reusable and can cause\ngarbage collector churn.\nBoth `asap` and `rawAsap` accept task objects to give you the option of\nrecycling task objects or using higher callable object abstractions.\nSee the `asap` source for an illustration.\n\n\n## Compatibility\n\nASAP is tested on Node.js v0.10 and in a broad spectrum of web browsers.\nThe following charts capture the browser test results for the most recent\nrelease.\nThe first chart shows test results for ASAP running in the main window context.\nThe second chart shows test results for ASAP running in a web worker context.\nTest results are inconclusive (grey) on browsers that do not support web\nworkers.\nThese data are captured automatically by [Continuous\nIntegration][].\n\n[Continuous Integration]: https://github.com/kriskowal/asap/blob/master/CONTRIBUTING.md\n\n\n\n\n\n## Caveats\n\nWhen a task is added to an empty event queue, it is not always possible to\nguarantee that the task queue will begin flushing immediately after the current\nevent.\nHowever, once the task queue begins flushing, it will not yield until the queue\nis empty, even if the queue grows while executing tasks.\n\nThe following browsers allow the use of [DOM mutation observers][] to access\nthe HTML [microtask queue][], and thus begin flushing ASAP's task queue\nimmediately at the end of the current event loop turn, before any rendering or\nIO:\n\n[microtask queue]: http://www.whatwg.org/specs/web-apps/current-work/multipage/webappapis.html#microtask-queue\n[DOM mutation observers]: http://dom.spec.whatwg.org/#mutation-observers\n\n- Android 4–4.3\n- Chrome 26–34\n- Firefox 14–29\n- Internet Explorer 11\n- iPad Safari 6–7.1\n- iPhone Safari 7–7.1\n- Safari 6–7\n\nIn the absense of mutation observers, there are a few browsers, and situations\nlike web workers in some of the above browsers, where [message channels][]\nwould be a useful way to avoid falling back to timers.\nMessage channels give direct access to the HTML [task queue][], so the ASAP\ntask queue would flush after any already queued rendering and IO tasks, but\nwithout having the minimum delay imposed by timers.\nHowever, among these browsers, Internet Explorer 10 and Safari do not reliably\ndispatch messages, so they are not worth the trouble to implement.\n\n[message channels]: http://www.whatwg.org/specs/web-apps/current-work/multipage/web-messaging.html#message-channels\n[task queue]: http://www.whatwg.org/specs/web-apps/current-work/multipage/webappapis.html#concept-task\n\n- Internet Explorer 10\n- Safair 5.0-1\n- Opera 11-12\n\nIn the absense of mutation observers, these browsers and the following browsers\nall fall back to using `setTimeout` and `setInterval` to ensure that a `flush`\noccurs.\nThe implementation uses both and cancels whatever handler loses the race, since\n`setTimeout` tends to occasionally skip tasks in unisolated circumstances.\nTimers generally delay the flushing of ASAP's task queue for four milliseconds.\n\n- Firefox 3–13\n- Internet Explorer 6–10\n- iPad Safari 4.3\n- Lynx 2.8.7\n\n\n## Heritage\n\nASAP has been factored out of the [Q][] asynchronous promise library.\nIt originally had a naïve implementation in terms of `setTimeout`, but\n[Malte Ubl][NonBlocking] provided an insight that `postMessage` might be\nuseful for creating a high-priority, no-delay event dispatch hack.\nSince then, Internet Explorer proposed and implemented `setImmediate`.\nRobert Katić began contributing to Q by measuring the performance of\nthe internal implementation of `asap`, paying particular attention to\nerror recovery.\nDomenic, Robert, and Kris Kowal collectively settled on the current strategy of\nunrolling the high-priority event queue internally regardless of what strategy\nwe used to dispatch the potentially lower-priority flush event.\nDomenic went on to make ASAP cooperate with Node.js domains.\n\n[Q]: https://github.com/kriskowal/q\n[NonBlocking]: http://www.nonblocking.io/2011/06/windownexttick.html\n\nFor further reading, Nicholas Zakas provided a thorough article on [The\nCase for setImmediate][NCZ].\n\n[NCZ]: http://www.nczonline.net/blog/2013/07/09/the-case-for-setimmediate/\n\nEmber’s RSVP promise implementation later [adopted][RSVP ASAP] the name ASAP but\nfurther developed the implentation.\nParticularly, The `MessagePort` implementation was abandoned due to interaction\n[problems with Mobile Internet Explorer][IE Problems] in favor of an\nimplementation backed on the newer and more reliable DOM `MutationObserver`\ninterface.\nThese changes were back-ported into this library.\n\n[IE Problems]: https://github.com/cujojs/when/issues/197\n[RSVP ASAP]: https://github.com/tildeio/rsvp.js/blob/cddf7232546a9cf858524b75cde6f9edf72620a7/lib/rsvp/asap.js\n\nIn addition, ASAP factored into `asap` and `asap/raw`, such that `asap` remained\nexception-safe, but `asap/raw` provided a tight kernel that could be used for\ntasks that guaranteed that they would not throw exceptions.\nThis core is useful for promise implementations that capture thrown errors in\nrejected promises and do not need a second safety net.\nAt the same time, the exception handling in `asap` was factored into separate\nimplementations for Node.js and browsers, using the the [Browserify][Browser\nConfig] `browser` property in `package.json` to instruct browser module loaders\nand bundlers, including [Browserify][], [Mr][], and [Mop][], to use the\nbrowser-only implementation.\n\n[Browser Config]: https://gist.github.com/defunctzombie/4339901\n[Browserify]: https://github.com/substack/node-browserify\n[Mr]: https://github.com/montagejs/mr\n[Mop]: https://github.com/montagejs/mop\n\n## License\n\nCopyright 2009-2014 by Contributors\nMIT License (enclosed)\n\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/kriskowal/asap/issues"
},
"_id": "asap@2.0.3",
"_from": "asap@~2.0.3",
"realName": "asap",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/promise/node_modules/asap",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/promise/node_modules/asap",
"parent": "[Circular]",
"depth": 4,
"peerDependencies": {}
}
},
"readme": " \n# promise\n\nThis is a simple implementation of Promises. It is a super set of ES6 Promises designed to have readable, performant code and to provide just the extensions that are absolutely necessary for using promises today.\n\nFor detailed tutorials on its use, see www.promisejs.org\n\n**N.B.** This promise exposes internals via underscore (`_`) prefixed properties. If you use these, your code will break with each new release.\n\n[![travis][travis-image]][travis-url]\n[![dep][dep-image]][dep-url]\n[![npm][npm-image]][npm-url]\n[![downloads][downloads-image]][downloads-url]\n\n[travis-image]: https://img.shields.io/travis/then/promise.svg?style=flat\n[travis-url]: https://travis-ci.org/then/promise\n[dep-image]: https://img.shields.io/gemnasium/then/promise.svg?style=flat\n[dep-url]: https://gemnasium.com/then/promise\n[npm-image]: https://img.shields.io/npm/v/promise.svg?style=flat\n[npm-url]: https://npmjs.org/package/promise\n[downloads-image]: https://img.shields.io/npm/dm/promise.svg?style=flat\n[downloads-url]: https://npmjs.org/package/promise\n\n## Installation\n\n**Server:**\n\n $ npm install promise\n\n**Client:**\n\nYou can use browserify on the client, or use the pre-compiled script that acts as a polyfill.\n\n```html\n\n```\n\nNote that the [es5-shim](https://github.com/es-shims/es5-shim) must be loaded before this library to support browsers pre IE9.\n\n```html\n\n```\n\n## Usage\n\nThe example below shows how you can load the promise library (in a way that works on both client and server using node or browserify). It then demonstrates creating a promise from scratch. You simply call `new Promise(fn)`. There is a complete specification for what is returned by this method in [Promises/A+](http://promises-aplus.github.com/promises-spec/).\n\n```javascript\nvar Promise = require('promise');\n\nvar promise = new Promise(function (resolve, reject) {\n get('http://www.google.com', function (err, res) {\n if (err) reject(err);\n else resolve(res);\n });\n});\n```\n\nIf you need [domains](https://iojs.org/api/domain.html) support, you should instead use:\n\n```js\nvar Promise = require('promise/domains');\n```\n\nIf you are in an environment that implements `setImmediate` and don't want the optimisations provided by asap, you can use:\n\n```js\nvar Promise = require('promise/setimmediate');\n```\n\nIf you only want part of the features, e.g. just a pure ES6 polyfill:\n\n```js\nvar Promise = require('promise/lib/es6-extensions');\n// or require('promise/domains/es6-extensions');\n// or require('promise/setimmediate/es6-extensions');\n```\n\n## Unhandled Rejections\n\nBy default, promises silence any unhandled rejections.\n\nYou can enable logging of unhandled ReferenceErrors and TypeErrors via:\n\n```js\nrequire('promise/lib/rejection-tracking').enable();\n```\n\nDue to the performance cost, you should only do this during development.\n\nYou can enable logging of all unhandled rejections if you need to debug an exception you think is being swallowed by promises:\n\n```js\nrequire('promise/lib/rejection-tracking').enable(\n {allRejections: true}\n);\n```\n\nDue to the high probability of false positives, I only recommend using this when debugging specific issues that you think may be being swallowed. For the preferred debugging method, see `Promise#done(onFulfilled, onRejected)`.\n\n`rejection-tracking.enable(options)` takes the following options:\n\n - allRejections (`boolean`) - track all exceptions, not just reference errors and type errors. Note that this has a high probability of resulting in false positives if your code loads data optimisticly\n - whitelist (`Array`) - this defaults to `[ReferenceError, TypeError]` but you can override it with your own list of error constructors to track.\n - `onUnhandled(id, error)` and `onHandled(id, error)` - you can use these to provide your own customised display for errors. Note that if possible you should indicate that the error was a false positive if `onHandled` is called. `onHandled` is only called if `onUnhandled` has already been called.\n\nTo reduce the chance of false-positives there is a delay of up to 2 seconds before errors are logged. This means that if you attach an error handler within 2 seconds, it won't be logged as a false positive. ReferenceErrors and TypeErrors are only subject to a 100ms delay due to the higher likelihood that the error is due to programmer error.\n\n## API\n\nBefore all examples, you will need:\n\n```js\nvar Promise = require('promise');\n```\n\n### new Promise(resolver)\n\nThis creates and returns a new promise. `resolver` must be a function. The `resolver` function is passed two arguments:\n\n 1. `resolve` should be called with a single argument. If it is called with a non-promise value then the promise is fulfilled with that value. If it is called with a promise (A) then the returned promise takes on the state of that new promise (A).\n 2. `reject` should be called with a single argument. The returned promise will be rejected with that argument.\n\n### Static Functions\n\n These methods are invoked by calling `Promise.methodName`.\n\n#### Promise.resolve(value)\n\n(deprecated aliases: `Promise.from(value)`, `Promise.cast(value)`)\n\nConverts values and foreign promises into Promises/A+ promises. If you pass it a value then it returns a Promise for that value. If you pass it something that is close to a promise (such as a jQuery attempt at a promise) it returns a Promise that takes on the state of `value` (rejected or fulfilled).\n\n#### Promise.reject(value)\n\nReturns a rejected promise with the given value.\n\n#### Promise.all(array)\n\nReturns a promise for an array. If it is called with a single argument that `Array.isArray` then this returns a promise for a copy of that array with any promises replaced by their fulfilled values. e.g.\n\n```js\nPromise.all([Promise.resolve('a'), 'b', Promise.resolve('c')])\n .then(function (res) {\n assert(res[0] === 'a')\n assert(res[1] === 'b')\n assert(res[2] === 'c')\n })\n```\n\n#### Promise.denodeify(fn)\n\n_Non Standard_\n\nTakes a function which accepts a node style callback and returns a new function that returns a promise instead.\n\ne.g.\n\n```javascript\nvar fs = require('fs')\n\nvar read = Promise.denodeify(fs.readFile)\nvar write = Promise.denodeify(fs.writeFile)\n\nvar p = read('foo.json', 'utf8')\n .then(function (str) {\n return write('foo.json', JSON.stringify(JSON.parse(str), null, ' '), 'utf8')\n })\n```\n\n#### Promise.nodeify(fn)\n\n_Non Standard_\n\nThe twin to `denodeify` is useful when you want to export an API that can be used by people who haven't learnt about the brilliance of promises yet.\n\n```javascript\nmodule.exports = Promise.nodeify(awesomeAPI)\nfunction awesomeAPI(a, b) {\n return download(a, b)\n}\n```\n\nIf the last argument passed to `module.exports` is a function, then it will be treated like a node.js callback and not parsed on to the child function, otherwise the API will just return a promise.\n\n### Prototype Methods\n\nThese methods are invoked on a promise instance by calling `myPromise.methodName`\n\n### Promise#then(onFulfilled, onRejected)\n\nThis method follows the [Promises/A+ spec](http://promises-aplus.github.io/promises-spec/). It explains things very clearly so I recommend you read it.\n\nEither `onFulfilled` or `onRejected` will be called and they will not be called more than once. They will be passed a single argument and will always be called asynchronously (in the next turn of the event loop).\n\nIf the promise is fulfilled then `onFulfilled` is called. If the promise is rejected then `onRejected` is called.\n\nThe call to `.then` also returns a promise. If the handler that is called returns a promise, the promise returned by `.then` takes on the state of that returned promise. If the handler that is called returns a value that is not a promise, the promise returned by `.then` will be fulfilled with that value. If the handler that is called throws an exception then the promise returned by `.then` is rejected with that exception.\n\n#### Promise#catch(onRejected)\n\nSugar for `Promise#then(null, onRejected)`, to mirror `catch` in synchronous code.\n\n#### Promise#done(onFulfilled, onRejected)\n\n_Non Standard_\n\nThe same semantics as `.then` except that it does not return a promise and any exceptions are re-thrown so that they can be logged (crashing the application in non-browser environments)\n\n#### Promise#nodeify(callback)\n\n_Non Standard_\n\nIf `callback` is `null` or `undefined` it just returns `this`. If `callback` is a function it is called with rejection reason as the first argument and result as the second argument (as per the node.js convention).\n\nThis lets you write API functions that look like:\n\n```javascript\nfunction awesomeAPI(foo, bar, callback) {\n return internalAPI(foo, bar)\n .then(parseResult)\n .then(null, retryErrors)\n .nodeify(callback)\n}\n```\n\nPeople who use typical node.js style callbacks will be able to just pass a callback and get the expected behavior. The enlightened people can not pass a callback and will get awesome promises.\n\n## License\n\n MIT\n",
"readmeFilename": "Readme.md",
"bugs": {
"url": "https://github.com/then/promise/issues"
},
"_id": "promise@7.1.1",
"_from": "promise@^7.0.3",
"realName": "promise",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/promise",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/promise",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
},
"ua-parser-js": {
"title": "UAParser.js",
"name": "ua-parser-js",
"version": "0.7.10",
"author": {
"name": "Faisal Salman",
"email": "fyzlman@gmail.com",
"url": "http://faisalman.com"
},
"description": "Lightweight JavaScript-based user-agent string parser",
"keywords": [
"user-agent",
"parser",
"browser",
"engine",
"os",
"device",
"cpu"
],
"homepage": "http://github.com/faisalman/ua-parser-js",
"contributors": [
{
"name": "Faisal Salman",
"email": "fyzlman@gmail.com"
},
{
"name": "Benjamin Bertrand",
"email": "bertrand.design@gmail.com"
},
{
"name": "Carl C Von Lewin",
"email": "carlchristianlewin@gmail.com"
},
{
"name": "Christopher De Cairos",
"email": "chris.decairos@gmail.com"
},
{
"name": "Davit Barbakadze",
"email": "jayarjo@gmail.com"
},
{
"name": "Dmitry Tyschenko",
"email": "dtyschenko@gmail.com"
},
{
"name": "Douglas Li",
"email": "doug@knotch.it"
},
{
"name": "Dumitru Uzun",
"email": "contact@duzun.me"
},
{
"name": "Erik Hesselink",
"email": "hesselink@gmail.com"
},
{
"name": "Fabian Becker",
"email": "halfdan@xnorfz.de"
},
{
"name": "Hendrik Helwich",
"email": "h.helwich@iplabs.de"
},
{
"name": "Jackpoll",
"email": "jackpoll123456@gmail.com"
},
{
"name": "Jake Mc",
"email": "startswithaj@users.noreply.github.com"
},
{
"name": "John Tantalo",
"email": "john.tantalo@gmail.com"
},
{
"name": "John Yanarella",
"email": "jmy@codecatalyst.com"
},
{
"name": "Jon Buckley",
"email": "jon@jbuckley.ca"
},
{
"name": "Kendall Buchanan",
"email": "kendall@kendagriff.com"
},
{
"name": "Lee Treveil",
"email": "leetreveil@gmail.com"
},
{
"name": "Leonardo",
"email": "leofiore@libero.it"
},
{
"name": "Max Maurer",
"email": "maxemanuel.maurer@gmail.com"
},
{
"name": "Michael Hess",
"email": "mhess@connectify.me"
},
{
"name": "OtakuSiD",
"email": "otakusid@gmail.com"
},
{
"name": "Ross Noble",
"email": "rosshnoble@gmail.com"
},
{
"name": "Sandro Sonntag",
"email": "sandro.sonntag@adorsys.de"
}
],
"main": "src/ua-parser.js",
"scripts": {
"build": "uglifyjs src/ua-parser.js > dist/ua-parser.min.js --comments '/UAParser\\.js/' && uglifyjs src/ua-parser.js > dist/ua-parser.pack.js --comments '/UAParser\\.js/' --compress --mangle",
"test": "jshint src/ua-parser.js && mocha -R nyan test/test.js",
"verup": "node ./node_modules/verup",
"version": "node ./node_modules/verup 0"
},
"verup": {
"files": [
"ua-parser-js.jquery.json",
"component.json",
"bower.json",
"package.js",
"src/ua-parser.js"
],
"regs": [
"^((?:\\$|(\\s*\\*\\s*@)|(\\s*(?:var|,)?\\s+))(?:LIBVERSION|version)[\\s\\:='\"]+)([0-9]+(?:\\.[0-9]+){2,2})",
"^(\\s?\\*.*v)([0-9]+(?:\\.[0-9]+){2,2})"
]
},
"devDependencies": {
"jshint": "~1.1.0",
"mocha": "~1.8.0",
"uglify-js": "~1.3.4",
"verup": "^1.3.x"
},
"repository": {
"type": "git",
"url": "https://github.com/faisalman/ua-parser-js.git"
},
"licenses": [
{
"type": "GPLv2",
"url": "http://www.gnu.org/licenses/gpl-2.0.html"
},
{
"type": "MIT",
"url": "http://www.opensource.org/licenses/mit-license.php"
}
],
"engines": {
"node": "*"
},
"directories": {
"dist": "dist",
"src": "src",
"test": "test"
},
"readme": "# UAParser.js\r\n\r\nLightweight JavaScript-based User-Agent string parser. Supports browser & node.js environment. Also available as jQuery/Zepto plugin, Component/Bower/Meteor package, & RequireJS/AMD module\r\n\r\n[](https://travis-ci.org/faisalman/ua-parser-js)\r\n[](http://flattr.com/thing/3867907/faisalmanua-parser-js-on-GitHub)\r\n\r\n* Author : Faisal Salman <>\r\n* Demo : http://faisalman.github.io/ua-parser-js\r\n* Source : https://github.com/faisalman/ua-parser-js\r\n\r\n## Features\r\n\r\nExtract detailed type of web browser, layout engine, operating system, cpu architecture, and device type/model purely from user-agent string with relatively lightweight footprint (~11KB minified / ~4KB gzipped). Written in vanilla js, which means it doesn't depends on any other library.\r\n\r\n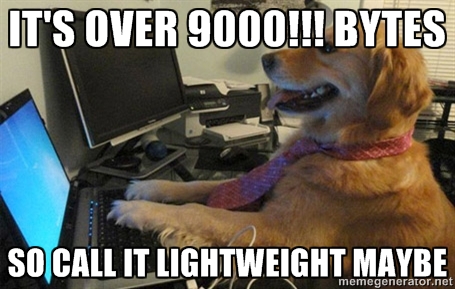\r\n\r\n## Methods\r\n\r\n* `getBrowser()`\r\n * returns `{ name: '', version: '' }`\r\n\r\n```\r\n# Possible 'browser.name':\r\nAmaya, Android Browser, Arora, Avant, Baidu, Blazer, Bolt, Camino, Chimera, Chrome, \r\nChromium, Comodo Dragon, Conkeror, Dillo, Dolphin, Doris, Edge, Epiphany, Fennec,\r\nFirebird, Firefox, Flock, GoBrowser, iCab, ICE Browser, IceApe, IceCat, IceDragon, \r\nIceweasel, IE [Mobile], Iron, Jasmine, K-Meleon, Konqueror, Kindle, Links, \r\nLunascape, Lynx, Maemo, Maxthon, Midori, Minimo, MIUI Browser, [Mobile] Safari, \r\nMosaic, Mozilla, Netfront, Netscape, NetSurf, Nokia, OmniWeb, Opera [Mini/Mobi/Tablet], \r\nPhantomJS, Phoenix, Polaris, QQBrowser, RockMelt, Silk, Skyfire, SeaMonkey, SlimBrowser,\r\nSwiftfox, Tizen, UCBrowser, Vivaldi, w3m, Yandex\r\n\r\n# 'browser.version' determined dynamically\r\n```\r\n\r\n* `getDevice()`\r\n * returns `{ model: '', type: '', vendor: '' }` \r\n\r\n```\r\n# Possible 'device.type':\r\nconsole, mobile, tablet, smarttv, wearable, embedded\r\n\r\n# Possible 'device.vendor':\r\nAcer, Alcatel, Amazon, Apple, Archos, Asus, BenQ, BlackBerry, Dell, GeeksPhone, \r\nGoogle, HP, HTC, Huawei, Jolla, Lenovo, LG, Meizu, Microsoft, Motorola, Nexian, \r\nNintendo, Nokia, Nvidia, Ouya, Palm, Panasonic, Polytron, RIM, Samsung, Sharp, \r\nSiemens, Sony-Ericsson, Sprint, Xbox, ZTE\r\n\r\n# 'device.model' determined dynamically\r\n```\r\n\r\n* `getEngine()`\r\n * returns `{ name: '', version: '' }`\r\n\r\n```\r\n# Possible 'engine.name'\r\nAmaya, EdgeHTML, Gecko, iCab, KHTML, Links, Lynx, NetFront, NetSurf, Presto, \r\nTasman, Trident, w3m, WebKit\r\n\r\n# 'engine.version' determined dynamically\r\n```\r\n\r\n* `getOS()`\r\n * returns `{ name: '', version: '' }`\r\n\r\n```\r\n# Possible 'os.name'\r\nAIX, Amiga OS, Android, Arch, Bada, BeOS, BlackBerry, CentOS, Chromium OS, Contiki,\r\nFedora, Firefox OS, FreeBSD, Debian, DragonFly, Gentoo, GNU, Haiku, Hurd, iOS, \r\nJoli, Linpus, Linux, Mac OS, Mageia, Mandriva, MeeGo, Minix, Mint, Morph OS, NetBSD, \r\nNintendo, OpenBSD, OpenVMS, OS/2, Palm, PCLinuxOS, Plan9, Playstation, QNX, RedHat, \r\nRIM Tablet OS, RISC OS, Sailfish, Series40, Slackware, Solaris, SUSE, Symbian, Tizen, \r\nUbuntu, UNIX, VectorLinux, WebOS, Windows [Phone/Mobile], Zenwalk\r\n\r\n# 'os.version' determined dynamically\r\n```\r\n\r\n* `getCPU()`\r\n * returns `{ architecture: '' }`\r\n\r\n```\r\n# Possible 'cpu.architecture'\r\n68k, amd64, arm, arm64, avr, ia32, ia64, irix, irix64, mips, mips64, pa-risc, \r\nppc, sparc, sparc64\r\n```\r\n\r\n* `getResult()`\r\n * returns `{ ua: '', browser: {}, cpu: {}, device: {}, engine: {}, os: {} }`\r\n\r\n* `getUA()`\r\n * returns UA string of current instance\r\n\r\n* `setUA(uastring)`\r\n * set & parse UA string\r\n\r\n## Example\r\n\r\n```html\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n```\r\n\r\n### Using node.js\r\n\r\n```sh\r\n$ npm install ua-parser-js\r\n```\r\n\r\n```js\r\nvar http = require('http');\r\nvar parser = require('ua-parser-js');\r\n\r\nhttp.createServer(function (req, res) {\r\n // get user-agent header\r\n var ua = parser(req.headers['user-agent']);\r\n // write the result as response\r\n res.end(JSON.stringify(ua, null, ' '));\r\n})\r\n.listen(1337, '127.0.0.1');\r\n\r\nconsole.log('Server running at http://127.0.0.1:1337/');\r\n```\r\n\r\n### Using requirejs\r\n\r\n```js\r\nrequire(['ua-parser'], function(UAParser) {\r\n var parser = new UAParser();\r\n console.log(parser.getResult());\r\n});\r\n```\r\n\r\n### Using component\r\n\r\n```sh\r\n$ component install faisalman/ua-parser-js\r\n```\r\n\r\n### Using bower\r\n\r\n```sh\r\n$ bower install ua-parser-js\r\n```\r\n\r\n### Using meteor\r\n\r\n```sh\r\n$ meteor add faisalman:ua-parser-js\r\n```\r\n\r\n### Using jQuery/Zepto ($.ua)\r\n\r\nAlthough written in vanilla js (which means it doesn't depends on jQuery), this library will automatically detect if jQuery/Zepto is present and create `$.ua` object based on browser's user-agent (although in case you need, `window.UAParser` constructor is still present). To get/set user-agent you can use: `$.ua.get()` / `$.ua.set(uastring)`. \r\n\r\n```js\r\n// In browser with default user-agent: 'Mozilla/5.0 (Linux; U; Android 2.3.4; en-us; Sprint APA7373KT Build/GRJ22) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0':\r\n\r\n// Do some tests\r\nconsole.log($.ua.device); // {vendor: \"HTC\", model: \"Evo Shift 4G\", type: \"mobile\"}\r\nconsole.log($.ua.os); // {name: \"Android\", version: \"2.3.4\"}\r\nconsole.log($.ua.os.name); // \"Android\"\r\nconsole.log($.ua.get()); // \"Mozilla/5.0 (Linux; U; Android 2.3.4; en-us; Sprint APA7373KT Build/GRJ22) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0\"\r\n\r\n// reset to custom user-agent\r\n$.ua.set('Mozilla/5.0 (Linux; U; Android 3.0.1; en-us; Xoom Build/HWI69) AppleWebKit/534.13 (KHTML, like Gecko) Version/4.0 Safari/534.13');\r\n\r\n// Test again\r\nconsole.log($.ua.browser.name); // \"Safari\"\r\nconsole.log($.ua.engine.name); // \"Webkit\"\r\nconsole.log($.ua.device); // {vendor: \"Motorola\", model: \"Xoom\", type: \"tablet\"}\r\nconsole.log(parseInt($.ua.browser.version.split('.')[0], 10)); // 4\r\n```\r\n\r\n### Extending regex patterns\r\n\r\n* `UAParser(uastring[, extensions])`\r\n\r\nPass your own regexes to extend the limited matching rules.\r\n\r\n```js\r\n// Example:\r\nvar uaString = 'ownbrowser/1.3';\r\nvar ownBrowser = [[/(ownbrowser)\\/([\\w\\.]+)/i], [UAParser.BROWSER.NAME, UAParser.BROWSER.VERSION]];\r\nvar parser = new UAParser(uaString, {browser: ownBrowser});\r\nconsole.log(parser.getBrowser()); // {name: \"ownbrowser\", version: \"1.3\"}\r\n```\r\n\r\n## Development\r\n\r\nVerify, test, & minify script\r\n\r\n```sh\r\n$ npm run test\r\n$ npm run build\r\n```\r\n\r\nThen submit a pull request to https://github.com/faisalman/ua-parser-js under `develop` branch.\r\n\r\n\r\n## License\r\n\r\nDual licensed under GPLv2 & MIT\r\n\r\nCopyright © 2012-2015 Faisal Salman <>\r\n\r\nPermission is hereby granted, free of charge, to any person obtaining a copy of \r\nthis software and associated documentation files (the \"Software\"), to deal in \r\nthe Software without restriction, including without limitation the rights to use, \r\ncopy, modify, merge, publish, distribute, sublicense, and/or sell copies of the \r\nSoftware, and to permit persons to whom the Software is furnished to do so, \r\nsubject to the following conditions:\r\n\r\nThe above copyright notice and this permission notice shall be included in all \r\ncopies or substantial portions of the Software.\r\n",
"readmeFilename": "readme.md",
"bugs": {
"url": "https://github.com/faisalman/ua-parser-js/issues"
},
"_id": "ua-parser-js@0.7.10",
"_from": "ua-parser-js@^0.7.9",
"realName": "ua-parser-js",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/ua-parser-js",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/ua-parser-js",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
},
"whatwg-fetch": {
"name": "whatwg-fetch",
"version": "0.9.0",
"main": "fetch.js",
"repository": {
"type": "git",
"url": "git://github.com/github/fetch"
},
"licenses": [
{
"type": "MIT",
"url": "https://github.com/github/fetch/blob/master/LICENSE"
}
],
"devDependencies": {
"bower": "1.3.8",
"chai": "1.10.0",
"jshint": "2.5.2",
"mocha-phantomjs": "3.5.2",
"mocha": "2.1.0",
"phantomjs": "1.9.13"
},
"files": [
"LICENSE",
"fetch.js"
],
"readme": "# window.fetch polyfill\n\nThe global `fetch` function is an easier way to make web requests and handle\nresponses than using an XMLHttpRequest. This polyfill is written as closely as\npossible to the standard Fetch specification at https://fetch.spec.whatwg.org.\n\n## Installation\n\nAvailable on [Bower](http://bower.io) as **fetch**.\n\n```sh\n$ bower install fetch\n```\n\nYou'll also need a Promise polyfill for older browsers.\n\n```sh\n$ bower install es6-promise\n```\n\nThis can also be installed with `npm`.\n\n```sh\n$ npm install whatwg-fetch --save\n```\n\n(For a node.js implementation, try [node-fetch](https://github.com/bitinn/node-fetch))\n\n## Usage\n\nThe `fetch` function supports any HTTP method. We'll focus on GET and POST\nexample requests.\n\n### HTML\n\n```javascript\nfetch('/users.html')\n .then(function(response) {\n return response.text()\n }).then(function(body) {\n document.body.innerHTML = body\n })\n```\n\n### JSON\n\n```javascript\nfetch('/users.json')\n .then(function(response) {\n return response.json()\n }).then(function(json) {\n console.log('parsed json', json)\n }).catch(function(ex) {\n console.log('parsing failed', ex)\n })\n```\n\n### Response metadata\n\n```javascript\nfetch('/users.json').then(function(response) {\n console.log(response.headers.get('Content-Type'))\n console.log(response.headers.get('Date'))\n console.log(response.status)\n console.log(response.statusText)\n})\n```\n\n### Post form\n\n```javascript\nvar form = document.querySelector('form')\n\nfetch('/query', {\n method: 'post',\n body: new FormData(form)\n})\n```\n\n### Post JSON\n\n```javascript\nfetch('/users', {\n method: 'post',\n headers: {\n 'Accept': 'application/json',\n 'Content-Type': 'application/json'\n },\n body: JSON.stringify({\n name: 'Hubot',\n login: 'hubot',\n })\n})\n```\n\n### File upload\n\n```javascript\nvar input = document.querySelector('input[type=\"file\"]')\n\nvar form = new FormData()\nform.append('file', input.files[0])\nform.append('user', 'hubot')\n\nfetch('/avatars', {\n method: 'post',\n body: form\n})\n```\n\n### Success and error handlers\n\nThis causes `fetch` to behave like jQuery's `$.ajax` by rejecting the `Promise`\non HTTP failure status codes like 404, 500, etc. The response `Promise` is\nresolved only on successful, 200 level, status codes.\n\n```javascript\nfunction status(response) {\n if (response.status >= 200 && response.status < 300) {\n return response\n }\n throw new Error(response.statusText)\n}\n\nfunction json(response) {\n return response.json()\n}\n\nfetch('/users')\n .then(status)\n .then(json)\n .then(function(json) {\n console.log('request succeeded with json response', json)\n }).catch(function(error) {\n console.log('request failed', error)\n })\n```\n\n### Response URL caveat\n\nThe `Response` object has a URL attribute for the final responded resource.\nUsually this is the same as the `Request` url, but in the case of a redirect,\nits all transparent. Newer versions of XHR include a `responseURL` attribute\nthat returns this value. But not every browser supports this. The compromise\nrequires setting a special server side header to tell the browser what URL it\njust requested (yeah, I know browsers).\n\n``` ruby\nresponse.headers['X-Request-URL'] = request.url\n```\n\nIf you want `response.url` to be reliable, you'll want to set this header. The\nday that you ditch this polyfill and use native fetch only, you can remove the\nheader hack.\n\n## Browser Support\n\n |  |  |  | \n--- | --- | --- | --- | --- |\nLatest ✔ | Latest ✔ | 9+ ✔ | Latest ✔ | 6.1+ ✔ |\n",
"readmeFilename": "README.md",
"description": "The global `fetch` function is an easier way to make web requests and handle responses than using an XMLHttpRequest. This polyfill is written as closely as possible to the standard Fetch specification at https://fetch.spec.whatwg.org.",
"bugs": {
"url": "https://github.com/github/fetch/issues"
},
"_id": "whatwg-fetch@0.9.0",
"_from": "whatwg-fetch@^0.9.0",
"scripts": {},
"realName": "whatwg-fetch",
"dependencies": {},
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/whatwg-fetch",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs/node_modules/whatwg-fetch",
"parent": "[Circular]",
"depth": 3,
"peerDependencies": {}
}
},
"devEngines": {
"node": ">=3",
"npm": "2.x"
},
"browserify": {
"transform": [
"loose-envify"
]
},
"readme": "# FBJS\n\n## Purpose\n\nTo make it easier for Facebook to share and consume our own JavaScript. Primarily this will allow us to ship code without worrying too much about where it lives, keeping with the spirit of `@providesModule` but working in the broader JavaScript ecosystem.\n\n**Note:** If you are consuming the code here and you are not also a Facebook project, be prepared for a bad time. APIs may appear or disappear and we may not follow semver strictly, though we will do our best to. This library is being published with our use cases in mind and is not necessarily meant to be consumed by the broader public. In order for us to move fast and ship projects like React and Relay, we've made the decision to not support everybody. We probably won't take your feature requests unless they align with our needs. There will be overlap in functionality here and in other open source projects.\n\n## Usage\n\nAny `@providesModule` modules that are used by your project should be added to `src/`. They will be built and added to `module-map.json`. This file will contain a map from `@providesModule` name to what will be published as `fbjs`. The `module-map.json` file can then be consumed in your own project, along with the [rewrite-modules](https://github.com/facebook/fbjs/blob/master/scripts/babel/rewrite-modules.js) Babel plugin (which we'll publish with this), to rewrite requires in your own project. Then, just make sure `fbjs` is a dependency in your `package.json` and your package will consume the shared code.\n\n```js\n// Before transform\nvar emptyFunction = require('emptyFunction');\n// After transform\nvar emptyFunction = require('fbjs/lib/emptyFunction');\n```\n\nSee React for an example of this. *Coming soon!*\n\n## Building\n\nIt's as easy as just running gulp. This assumes you've also done `npm install -g gulp`.\n\n```sh\ngulp\n```\n\nAlternatively `npm run build` will also work.\n\n### Layout\n\nRight now these packages represent a subset of packages that we use internally at Facebook. Mostly these are support libraries used when shipping larger libraries, like React and Relay, or products. Each of these packages is in its own directory under `src/`.\n\n### Process\n\nSince we use `@providesModule`, we need to rewrite requires to be relative. Thanks to `@providesModule` requiring global uniqueness, we can do this easily. Eventually we'll try to make this part of the process go away by making more projects use CommmonJS.\n\n\n## TODO\n\n- Flow: Ideally we'd ship our original files with type annotations, however that's not doable right now. We have a couple options:\n - Make sure our transpilation step converts inline type annotations to the comment format.\n - Make our build process also build Flow interface files which we can ship to npm.\n- Split into multiple packages. This will be better for more concise versioning, otherwise we'll likely just be shipping lots of major versions.\n",
"readmeFilename": "README.md",
"bugs": {
"url": "https://github.com/facebook/fbjs/issues"
},
"_id": "fbjs@0.6.1",
"_from": "fbjs@^0.6.1",
"realName": "fbjs",
"path": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react/node_modules/fbjs",
"parent": "[Circular]",
"depth": 2,
"peerDependencies": {}
}
},
"browserify": {
"transform": [
"envify"
]
},
"readme": "# react\n\nAn npm package to get you immediate access to [React](https://facebook.github.io/react/),\nwithout also requiring the JSX transformer. This is especially useful for cases where you\nwant to [`browserify`](https://github.com/substack/node-browserify) your module using\n`React`.\n\n**Note:** by default, React will be in development mode. The development version includes extra warnings about common mistakes, whereas the production version includes extra performance optimizations and strips all error messages.\n\nTo use React in production mode, set the environment variable `NODE_ENV` to `production`. A minifier that performs dead-code elimination such as [UglifyJS](https://github.com/mishoo/UglifyJS2) is recommended to completely remove the extra code present in development mode.\n\n## Example Usage\n\n```js\nvar React = require('react');\n\n// Addons are in separate packages:\nvar createFragment = require('react-addons-create-fragment');\nvar immutabilityHelpers = require('react-addons-update');\nvar CSSTransitionGroup = require('react-addons-css-transition-group');\n```\n\nFor a complete list of addons visit the [addons documentation page](https://facebook.github.io/react/docs/addons.html).\n",
"readmeFilename": "README.md",
"_id": "react@0.14.7",
"_from": "react@^0.14.0",
"scripts": {},
"realName": "react",
"extraneous": false,
"path": "/home/flavorjones/tmp/npm-test/node_modules/react",
"realPath": "/home/flavorjones/tmp/npm-test/node_modules/react",
"parent": "[Circular]",
"depth": 1,
"peerDependencies": {}
}
},
"readme": "ERROR: No README data found!",
"_id": "asdf@0.0.0",
"realName": "asdf",
"path": "/home/flavorjones/tmp/npm-test",
"realPath": "/home/flavorjones/tmp/npm-test",
"depth": 0,
"peerDependencies": {}
}